How to Split String by Space in C++
-
Use
std::string::find
andstd::string::substr
Functions to Split String by Space in C++ -
Use
std::istringstream
andstd::copy
to Split String by Space in C++ -
Use
std::getline
anderase-remove
Idiom to Split String by Space in C++ -
Use the
strtok()
Function to Split String by Space in C++ -
Use
std::regex
to Split String by Space in C++
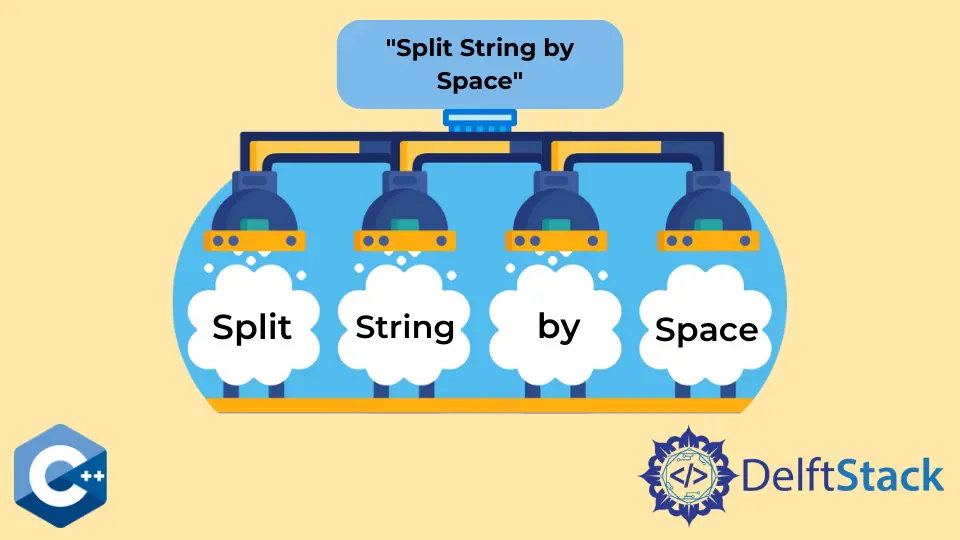
This article will demonstrate multiple methods about how to split string by space delimiter in C++.
Splitting strings involves breaking down a string into smaller segments based on a specific delimiter.
This process is crucial for extracting meaningful information from larger text blocks.
Whether you’re processing user input or parsing data, understanding string splitting techniques is essential.
Use std::string::find
and std::string::substr
Functions to Split String by Space in C++
find
and substr
are std::string
builtin functions that can be utilized to split string by any delimiter specified by the string value or a single character.
The find
function takes a string
argument and returns the position on which the given substring starts; otherwise, if not found, string::npos
is returned.
Thus, we iterate in the while
loop until the find
function returns npos
. Meanwhile, the substr
method can be utilized to access the part of the string before the delimiter, which in this case is a single space character and store into a vector
for later usage.
After that, we call the erase
function to remove the first sequence, including the delimiter, at which point a new iteration may proceed to repeat the operations.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text =
"Lorem ipsum dolor sit amet, consectetur adipiscing elit. "
"Sed laoreet sem leo, in posuere orci elementum.";
string space_delimiter = " ";
vector<string> words{};
size_t pos = 0;
while ((pos = text.find(space_delimiter)) != string::npos) {
words.push_back(text.substr(0, pos));
text.erase(0, pos + space_delimiter.length());
}
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
Output:
Lorem
ipsum
dolor
sit
amet,
consectetur
adipiscing
elit.
Sed
laoreet
sem
leo,
in
posuere
orci
Use std::istringstream
and std::copy
to Split String by Space in C++
Alternatively, we can reimplement the code using the istringstream
class, which provides input/output operations for string
based streams.
Once we initialize the istringstream
object with the string
value that needs to be split, then the std::copy
algorithm can be called to output each space-separated string value to the cout
stream.
Note that this method only supports the space delimiter splitting because that’s what the implementation of the istringstream
class provides.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text =
"Lorem ipsum dolor sit amet, consectetur adipiscing elit. "
"Sed laoreet sem leo, in posuere orci elementum.";
vector<string> words{};
istringstream iss(text);
copy(std::istream_iterator<string>(iss), std::istream_iterator<string>(),
std::ostream_iterator<string>(cout, "\n"));
return EXIT_SUCCESS;
}
Use std::getline
and erase-remove
Idiom to Split String by Space in C++
One downside of the previous solution is the punctuation symbols stored with parsed words. It may be solved with the erase-remove idiom, which is essentially a conditional removal operation for the given range.
In this case, we call this method on each word retrieved by std::getline
to trim all punctuation symbols in it.
Note that the ispunct
function object is passed as the third argument to the remove_if
algorithm to check for the punctuation characters.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text =
"Lorem ipsum dolor sit amet, consectetur adipiscing elit. "
"Sed laoreet sem leo, in posuere orci elementum.";
char space_char = ' ';
vector<string> words{};
stringstream sstream(text);
string word;
while (std::getline(sstream, word, space_char)) {
word.erase(std::remove_if(word.begin(), word.end(), ispunct), word.end());
words.push_back(word);
}
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
Output:
Lorem
ipsum
dolor
sit
amet
consectetur
adipiscing
elit
Sed
laoreet
sem
leo
in
posuere
orci
elementum
Use the strtok()
Function to Split String by Space in C++
The strtok()
function is part of the C standard library and is used for tokenizing strings. It takes a string and a delimiter as inputs, and it returns a sequence of tokens, which are substrings separated by the delimiter.
The function modifies the original string by inserting null characters ('\0'
) at the delimiter positions.
Syntax:
char *strtok(char *str, const char *delimiters);
str
: The input string to be tokenized.delimiters
: A string containing all possible delimiter characters.
The function returns a pointer to the next token found in the string or NULL
if no more tokens are found.
The strtok()
Process: Step by Step
To split a string using strtok()
, follow these steps:
-
Include the required header:
#include <cstring>
-
Declare the input string and delimiter:
char input[] = "Splitting strings using strtok function in C++"; const char delimiter[] = " ";
-
Initialize
strtok()
with the input string:
char *token = strtok(input, delimiter);
-
Use a loop to iterate through the tokens:
while (token != NULL) { // Process the token // ... // Move to the next token token = strtok(NULL, delimiter); }
-
Inside the loop, each
token
points to a substring that doesn’t contain the delimiter. You can perform operations on the token, such as printing it or further processing.
Example: Splitting String by Space Using strtok()
Let’s go through a comprehensive example to split a string into words using the strtok()
function:
#include <cstring>
#include <iostream>
int main() {
char input[] = "Welcome to the world of C++ programming";
const char delimiter[] = " ";
// Initialize strtok with the input string
char *token = strtok(input, delimiter);
// Iterate through the tokens
while (token != NULL) {
std::cout << token << std::endl;
// Move to the next token
token = strtok(NULL, delimiter);
}
return 0;
}
Output:
Welcome
to
the
world
of
C++
programming
In this example, the strtok()
function takes the input string "Welcome to the world of C++ programming"
and splits it into tokens based on the space delimiter. The loop iterates through each token and prints it.
Use std::regex
to Split String by Space in C++
The std::regex
library in C++ provides support for regular expressions, which are patterns used to match and manipulate strings.
The library offers flexible and powerful capabilities for pattern matching and replacement. When it comes to splitting strings, std::regex
allows you to define a pattern that represents the delimiter and use it to split the input string.
The std::regex
Process: Step by Step
To split a string using std::regex
, follow these steps:
-
Include the required header:
#include <regex>
-
Declare the input string and the delimiter pattern:
std::string input = "Using std::regex to split strings in C++";
std::regex delimiter("\\s+");
-
Use
std::sregex_token_iterator
to iterate through the tokens:std::sregex_token_iterator tokenIterator(input.begin(), input.end(), delimiter, -1); std::sregex_token_iterator endIterator;
-
Iterate through the tokens using the iterator:
while (tokenIterator != endIterator) { // Process the token std::cout << *tokenIterator << std::endl; // Move to the next token ++tokenIterator; }
Example: Splitting String by Space Using std::regex
Here’s a comprehensive example that demonstrates how to split a string into words using the std::regex
library:
#include <iostream>
#include <regex>
int main() {
std::string input = "Splitting strings using std::regex in C++";
std::regex delimiter("\\s+");
// Use sregex_token_iterator to tokenize the string
std::sregex_token_iterator tokenIterator(input.begin(), input.end(),
delimiter, -1);
std::sregex_token_iterator endIterator;
// Iterate through the tokens
while (tokenIterator != endIterator) {
std::cout << *tokenIterator << std::endl;
// Move to the next token
++tokenIterator;
}
return 0;
}
Output:
Splitting
strings
using
std::regex
in
C++
In this example, the std::regex
library is used to split the input string "Splitting strings using std::regex in C++"
into tokens based on the space delimiter. The loop iterates through each token and prints it.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++