How to Pass Argument by Reference vs by Pointer in C++
-
Use
&variable
Notation to Pass Function Arguments by Reference in C++ -
Use
*variable
Notation to Pass Function Arguments by Reference in C++ -
Use
(&array_variable)[x][y]
Notation to Pass 2D Array by Reference in C++ - When to Use Passing by Reference vs Passing by Pointer
- Conclusion
- FAQ
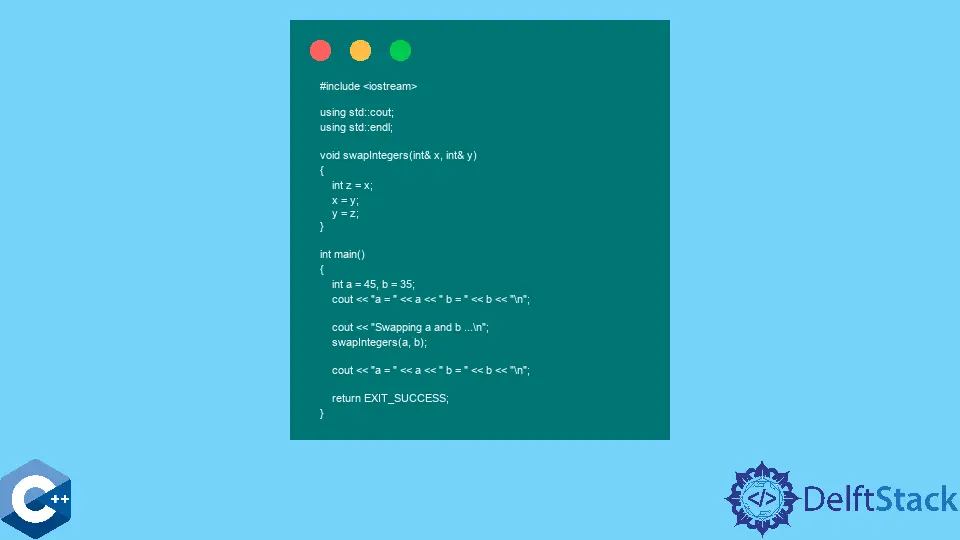
Understanding how to pass arguments by reference and by pointer in C++ is crucial for efficient programming. These two methods allow you to manipulate data without creating unnecessary copies, which can enhance performance, especially when working with large data structures.
In this article, we will dive into the details of each method, highlighting their differences, advantages, and use cases. By the end, you will have a clear grasp of when to use passing by reference versus passing by pointer, along with practical examples to illustrate these concepts. Let’s get started!
Use &variable
Notation to Pass Function Arguments by Reference in C++
Passing arguments is the most common feature of functions to provide a flexible interface for data exchange between different code blocks. Each time function is invoked, its parameters are created and initialized by the arguments that were passed. Generally, two methods are differentiated for argument passing: passed by value and passed by reference, the latter of which causes the parameter to be an alias for the corresponding argument. We demonstrate a basic integer swap function in the following example, where arguments are passed by references and int &var
notation is used. When the swapIntegers
function returns, the int
objects in the main
function scope are modified.
#include <iostream>
using std::cout;
using std::endl;
void swapIntegers(int& x, int& y) {
int z = x;
x = y;
y = z;
}
int main() {
int a = 45, b = 35;
cout << "a = " << a << " b = " << b << "\n";
cout << "Swapping a and b ...\n";
swapIntegers(a, b);
cout << "a = " << a << " b = " << b << "\n";
return EXIT_SUCCESS;
}
Output:
a = 45 b = 35
Swapping a and b ...
a = 35 b = 45
Use *variable
Notation to Pass Function Arguments by Reference in C++
Similar behavior to the previous example can be implemented using pointers. Note that a pointer is an address of the object, and it can be dereferenced with the *
operator to access the object value. Passing the arguments using the pointer means that we can access the given objects from the function scope and modify their values so that the state is preserved after the return. Notice that, to pass pointers to the function, they need to be accessed using the address-of (&
) operator.
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
using std::setw;
void swapIntegers2(int* x, int* y) {
int z = *x;
*x = *y;
*y = z;
}
int main() {
int a = 45, b = 35;
cout << "a = " << a << " b = " << b << "\n";
cout << "Swapping a and b ...\n";
swapIntegers2(&a, &b);
cout << "a = " << a << " b = " << b << "\n";
return EXIT_SUCCESS;
}
Output:
a = 45 b = 35
Swapping a and b ...
a = 35 b = 45
Use (&array_variable)[x][y]
Notation to Pass 2D Array by Reference in C++
Sometimes it can be handy to pass two-dimensional C-style array reference to the function, but the notation is slightly nonintuitive and could lead to erroneous results. If we have an array of integers with the arbitrary SIZE
dimensions, it can be referenced from the function parameter with the following notation - int (&arr)[SIZE][SIZE]
. Mind that, missing the parenthesis ()
will be interpreted as the array of references to int
objects and result in compilation error.
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
using std::setw;
void MultiplyArrayByTwoV2(int (&arr)[SIZE][SIZE]) {
for (auto& i : arr) {
for (int& j : i) j *= 2;
}
}
void printArray(int (&arr)[SIZE][SIZE]) {
for (auto& i : arr) {
cout << " [ ";
for (int j : i) {
cout << setw(2) << j << ", ";
}
cout << "]" << endl;
}
}
constexpr int SIZE = 4;
int main() {
int array_2d[SIZE][SIZE] = {
{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}, {13, 14, 15, 16}};
MultiplyArrayByTwoV2(array_2d);
printArray(array_2d);
return EXIT_SUCCESS;
}
Output:
[ 2, 4, 6, 8, ]
[ 10, 12, 14, 16, ]
[ 18, 20, 22, 24, ]
[ 26, 28, 30, 32, ]
When to Use Passing by Reference vs Passing by Pointer
Choosing between passing by reference and passing by pointer often depends on the specific requirements of your program. Here are some guidelines:
-
Use Passing by Reference when:
- You want to modify the original variable.
- You are working with large data structures and want to avoid copying.
- You want to ensure that the argument is always valid (references cannot be null).
-
Use Passing by Pointer when:
- You need to indicate that the function may not modify the variable.
- You want to allow for the possibility of passing null values.
- You need to work with dynamic memory allocation or arrays.
Understanding these distinctions can help you write more efficient and effective C++ programs.
Conclusion
In summary, passing arguments by reference and by pointer in C++ are both powerful techniques that serve different purposes. Passing by reference is straightforward and prevents unnecessary copies, while passing by pointer offers flexibility with null pointers and dynamic memory management. By mastering both methods, you can optimize your C++ code for performance and clarity. Remember to choose the method that best fits your use case to enhance your programming skills and improve the efficiency of your applications.
FAQ
-
What is the main difference between passing by reference and passing by pointer?
Passing by reference allows direct access to the original variable without copying, while passing by pointer provides access via the variable’s memory address, allowing for more flexibility but requiring careful management. -
Can I pass a constant variable by reference?
Yes, you can pass a constant variable by reference in C++. However, you must declare the reference parameter as a constant reference to prevent modifications. -
What happens if I pass a null pointer to a function?
If you pass a null pointer to a function and attempt to dereference it, your program will likely crash with a segmentation fault. Always check for null pointers before dereferencing. -
Is passing by reference safer than passing by pointer?
Generally, yes. Passing by reference ensures that the variable is valid and cannot be null, reducing the risk of errors compared to passing by pointer. -
Can I use both passing by reference and passing by pointer in the same function?
Yes, you can use both methods in the same function, but it’s essential to ensure that the logic remains clear and understandable for maintainability.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook