How to Parse Int From String in C++
- Using std::stoi
- Using std::stringstream
-
Use the
std::from_chars
Function to Parse Int From String - Using C-style Functions
- Conclusion
- FAQ
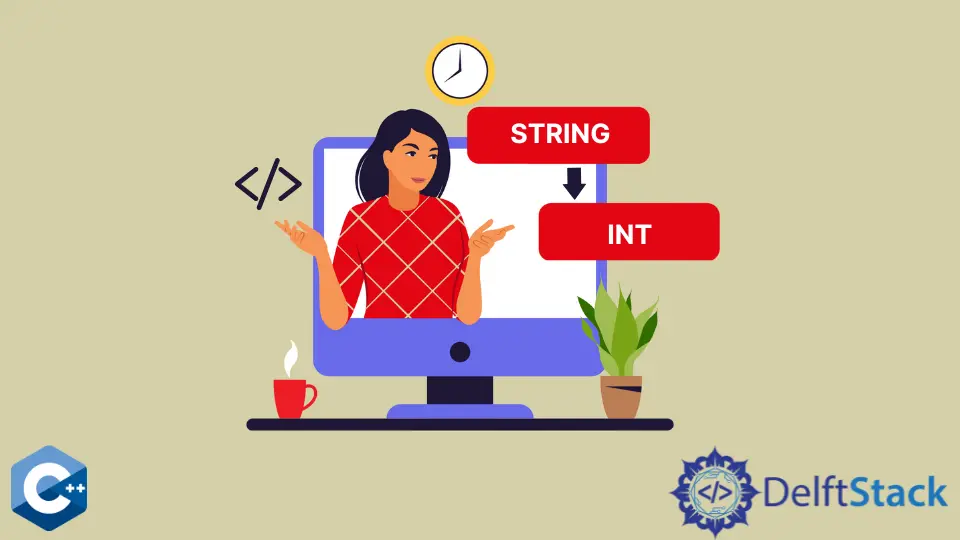
Parsing integers from strings is a common task in programming, especially in C++. Whether you’re reading user input, processing data from files, or handling network communications, knowing how to convert a string to an integer is essential.
In this article, we will explore various methods to parse an int from a string in C++. We’ll cover different techniques, including using standard library functions and string streams, to help you understand the best practices for this task. By the end of this guide, you’ll be equipped with the knowledge to handle string-to-integer conversions efficiently and effectively in your C++ applications.
Using std::stoi
One of the simplest and most straightforward ways to convert a string to an integer in C++ is by using the std::stoi
function. This function is part of the standard library and provides a clean interface for parsing integers from strings.
Here’s an example:
#include <iostream>
#include <string>
int main() {
std::string str = "1234";
int number = std::stoi(str);
std::cout << "Parsed integer: " << number << std::endl;
return 0;
}
Output:
Parsed integer: 1234
In this code snippet, we first include the necessary headers. We then define a string containing the number we want to parse. The std::stoi
function is called with the string as an argument, returning the parsed integer. Finally, we print the result to the console.
Using std::stoi
is efficient and straightforward, but it does come with some caveats. If the string cannot be converted to an integer, std::stoi
will throw a std::invalid_argument
exception. If the number is out of range for the integer type, it will throw a std::out_of_range
exception. Therefore, it’s essential to handle these exceptions appropriately, especially in applications where user input can be unpredictable.
Using std::stringstream
Another effective method to parse an integer from a string in C++ is by utilizing the std::stringstream
class. This approach offers more flexibility, especially when dealing with formatted strings or when you need to extract multiple values from a single string.
Here’s how you can do it:
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string str = "5678";
std::stringstream ss(str);
int number;
ss >> number;
if (ss.fail()) {
std::cerr << "Error: Conversion failed." << std::endl;
return 1;
}
std::cout << "Parsed integer: " << number << std::endl;
return 0;
}
Output:
Parsed integer: 5678
In this example, we include the necessary headers and define a string containing the integer to be parsed. We create a std::stringstream
object initialized with the string. By using the extraction operator (>>
), we attempt to read the integer from the string stream into the number
variable.
One of the benefits of using std::stringstream
is its ability to handle formatted input. If the conversion fails, we can check the state of the stream using ss.fail()
, allowing us to implement error handling gracefully. This method is particularly useful when parsing complex strings or when you expect multiple data types.
Use the std::from_chars
Function to Parse Int From String
As an alternative, the from_chars
function from the utilities library can parse int
values. It’s been part of the standard library since the C++17 version and is defined in the <charconv>
header file.
Much different from the stoi
, from_chars
operates on the ranges of characters, not being aware of the object length and borders. Thus, the programmer should specify the start and end of the range as the first two arguments. The third argument is the int
variable to which the converted value will be assigned.
Mind though, from_chars
can only handle the leading -
sign in the input string; hence it prints garbage values of num5
and num6
in the following example.
#include <charconv>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::from_chars;
using std::stoi;
using std::string;
int main() {
string s1 = "333";
string s2 = "-333";
string s3 = "333xio";
string s4 = "01011101";
string s5 = " 333";
string s6 = "0x33";
int num1, num2, num3, num4, num5, num6;
from_chars(s1.c_str(), s1.c_str() + s1.length(), num1);
from_chars(s2.c_str(), s2.c_str() + s2.length(), num2);
from_chars(s3.c_str(), s3.c_str() + s3.length(), num3);
from_chars(s4.c_str(), s4.c_str() + s4.length(), num4, 2);
from_chars(s5.c_str(), s5.c_str() + s5.length(), num5);
from_chars(s6.c_str(), s6.c_str() + s6.length(), num6);
cout << "num1: " << num1 << " | num2: " << num2 << endl;
cout << "num3: " << num3 << " | num4: " << num4 << endl;
cout << "num5: " << num5 << " | num6: " << num6 << endl;
return EXIT_SUCCESS;
}
Output:
num1: 333 | num2: -333
num3: 333 | num4: 93
num5: -1858679306 | num6: 0
Using C-style Functions
For those who prefer a more traditional approach, C-style functions like atoi
can also be used to parse integers from strings. However, this method is generally less safe compared to the C++ standard library functions.
Here’s a basic example:
#include <iostream>
#include <cstdlib>
int main() {
const char* str = "91011";
int number = std::atoi(str);
std::cout << "Parsed integer: " << number << std::endl;
return 0;
}
Output:
Parsed integer: 91011
In this code, we include the necessary headers and define a C-style string (a character array). The std::atoi
function is called, which converts the string to an integer. The result is printed to the console.
While atoi
is simple to use, it has some significant drawbacks. It does not provide error handling, meaning that if the input string is invalid, it will return zero without any indication of failure. For this reason, it’s generally recommended to use std::stoi
or std::stringstream
for better safety and error handling.
Conclusion
Parsing an integer from a string in C++ can be accomplished using various methods, each with its strengths and weaknesses. The std::stoi
function offers a straightforward approach with built-in error handling, while std::stringstream
provides flexibility for more complex parsing needs. C-style functions like atoi
are available but should be used with caution due to their lack of error handling. By understanding these methods, you’ll be better equipped to handle string-to-integer conversions in your C++ applications.
FAQ
-
What is the best method to parse an int from a string in C++?
The best method depends on your specific needs, butstd::stoi
is generally recommended for its simplicity and built-in error handling. -
Can I parse negative integers from strings using std::stoi?
Yes,std::stoi
can handle negative integers as long as the string is formatted correctly. -
What happens if the string cannot be converted to an integer?
If you usestd::stoi
, it will throw astd::invalid_argument
exception. Withstd::stringstream
, you can check for failure usingss.fail()
. -
Is it safe to use atoi for converting strings to integers?
Whileatoi
is simple, it lacks error handling, making it less safe thanstd::stoi
orstd::stringstream
. It’s better to avoid it in favor of safer alternatives. -
Can I parse multiple integers from a single string?
Yes, you can usestd::stringstream
to extract multiple integers from a single string using the extraction operator multiple times.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook