How to Check if String Is Palindrome in C++
-
Use
string
Copy Constructor Withrbegin
/rend
Methods to Check for String Palindrome in C++ -
Use the
std::equal
Method to Check for String Palindrome in C++ - Use Custom Function to Check for String Palindrome in C++
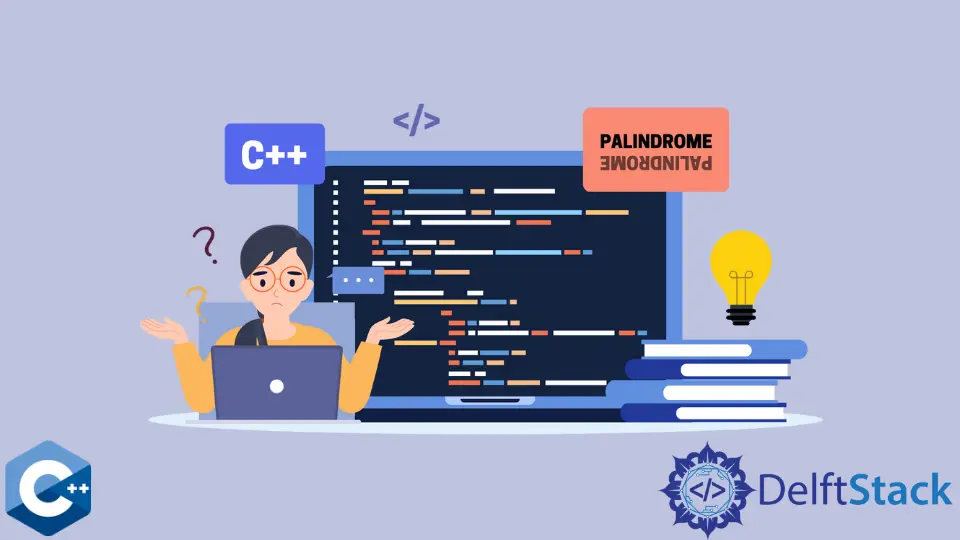
This article will explain several methods of how to check if a string is a palindrome in C++.
Use string
Copy Constructor With rbegin
/rend
Methods to Check for String Palindrome in C++
The string
class objects support comparison using the operator ==
, and it can be utilized to find a string that conforms to the palindrome pattern. Since the palindrome implies matching the characters in reverse order, we need to construct a new string object with rbegin
and rend
iterators. The rest is left to be constructed in the if
statement as needed.
In the following example, we declare two strings - one-word palindrome and multiple-word palindrome. Notice that this method can’t detect the string with spaces as a palindrome, although it fits the definition pattern.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::equal;
using std::remove;
using std::string;
int main() {
string s1 = "radar";
string s2 = "Was it a cat I saw";
if (s1 == string(s1.rbegin(), s1.rend())) {
cout << "s1 is a palindrome" << endl;
} else {
cout << "s1 is not a palindrome" << endl;
}
if (s2 == string(s2.rbegin(), s2.rend())) {
cout << "s2 is a palindrome" << endl;
} else {
cout << "s2 is not a palindrome" << endl;
}
return EXIT_SUCCESS;
}
Output:
s1 is a palindrome
s2 is not a palindrome
Use the std::equal
Method to Check for String Palindrome in C++
Even though the last implementation does the job on one-word strings, it comes with the overhead of creating an object copy and comparing full ranges of them. We can utilize the std::equal
algorithm to compare the first half with the second half of the same string
object range. std::equal
returns the boolean value true
if the elements in the given two ranges are equal. Note that the function takes only one iterator - s1.rbegin()
for the second range, because the end of the range is calculated as first2 + (last1 - first1)
.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::equal;
using std::remove;
using std::string;
int main() {
string s1 = "radar";
string s2 = "Was it a cat I saw";
equal(s1.begin(), s1.begin() + s1.size() / 2, s1.rbegin())
? cout << "s1 is a palindrome" << endl
: cout << "s1 is not a palindrome" << endl;
equal(s2.begin(), s2.begin() + s2.size() / 2, s2.rbegin())
? cout << "s2 is a palindrome" << endl
: cout << "s2 is not a palindrome" << endl;
return EXIT_SUCCESS;
}
Output:
s1 is a palindrome
s2 is not a palindrome
Use Custom Function to Check for String Palindrome in C++
Previous methods fall short on strings with multiple words, which we can solve by implementing a custom function. The example demonstrates the boolean function checkPalindrome
that takes the string&
argument and stores its value in a local string
variable. The local object is then processed with the transform
algorithm to convert it to lowercase and, consequently, by the erase-remove
idiom to delete all white-space characters in it. Finally, we call the equal
algorithm in the if
statement condition and return the corresponding boolean value. Mind though, that this method will fail if the string is consists of multibyte characters. Thus, the lowercase conversion method should be implemented that supports all common character encoding schemes.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::equal;
using std::remove;
using std::string;
bool checkPalindrome(string& s) {
string tmp = s;
transform(tmp.begin(), tmp.end(), tmp.begin(),
[](unsigned char c) { return tolower(c); });
tmp.erase(remove(tmp.begin(), tmp.end(), ' '), tmp.end());
if (equal(tmp.begin(), tmp.begin() + tmp.size() / 2, tmp.rbegin())) {
return true;
} else {
return false;
}
}
int main() {
string s1 = "radar";
string s2 = "Was it a cat I saw";
checkPalindrome(s1) ? cout << "s1 is a palindrome" << endl
: cout << "s1 is not a palindrome" << endl;
checkPalindrome(s2) ? cout << "s2 is a palindrome" << endl
: cout << "s2 is not a palindrome" << endl;
return EXIT_SUCCESS;
}
Output:
s1 is a palindrome
s2 is a palindrome
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++