Operator Precedence and Associativity in C++
- Operators in C++
- Operator Precedence and Associativity in C++
- Implicit Type Conversion in Expressions in C++
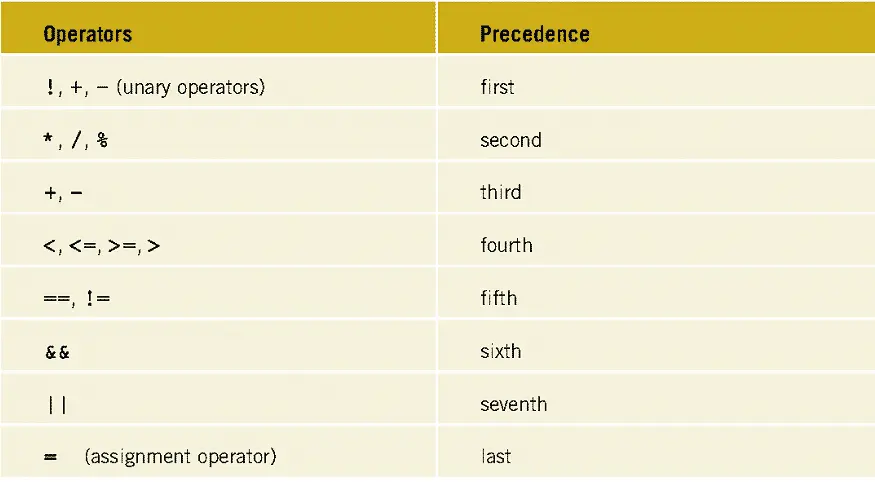
This article will discuss the operators in C++ and how they are evaluated when used in an expression. There are specific rules for their manipulation, so we will look at them to learn about the expression evaluation using C++ operators.
Operators in C++
Operators are symbols used to manipulate variables and values. For example, the +
and -
operators are used for addition and subtraction.
In C++, operators are subdivided into 4 categories:
- Arithmetic Operator
- Assignment Operator
- Relational Operator
- Logical Operator
Arithmetic Operators
These operators are used to perform different arithmetic operations on some data. For example, *
is used to multiply two or more numbers or variables.
There are numerous arithmetic operators are listed below:
Operator Symbol | Description |
---|---|
+ |
Addition operator |
- |
Subtraction Operator |
* |
Multiply Operator |
/ |
Division Operator |
% |
Modulus Operator (Remainder after division operation) |
Assignment Operator
These operators can assign some specific value to a variable; =
is the assignment operator. Consider the example below.
int a;
a = 5;
Here, a
will be assigned the value 5
using the assignment operator =
. There are other assignment operators as well, which are listed below:
Operator | Equivalent Statement |
---|---|
a += 5 |
a = a +5 |
a -= 5 |
a = a -5 |
a *= 5 |
a = a*5 |
a /= 5 |
a = a /5 |
a %= 5 |
a = a%5 |
Relational Operator
Relational operators are used for comparing 2 values or operands. If the relation is evaluated as true, it returns the value 1
, and if it is evaluated as false, it returns 0
.
Multiple relational operators are used in C++:
Operator | Description |
---|---|
< , > |
Greater than and less than |
<= , >= |
Less than and equal to, greater than and equal to |
== |
Equals to |
!= |
Not equal to |
Logical Operators
Logical operators can evaluate whether an expression is true or false. If it is true, the result returned is 1
; otherwise, 0
.
There are multiple logical operators, which are listed below:
Operators | Description |
---|---|
&& |
AND Operator. This returns true if all the expressions are true. |
` | |
! |
NOT Operator. This returns true if the expression is false. |
Operator Precedence and Associativity in C++
If we have an expression with more than one operator, then the expression is evaluated according to the precedence of operators. Precedence defines the priority of operators and which operator is evaluated first.
Consider the example below:
a = 3 * 5 / 2 + 3
Now in this expression, which operator will be manipulated first is decided by the precedence of operators.
The list of operator precedence is shown in the table below:
Now, we can see certain operators have the same precedence level. The expression having such operators with the same precedence level is evaluated using the associativity rule.
Associativity defines the sequence of operators to be evaluated at the same precedence level. Below is the table that describes the associativity of operators:
Implicit Type Conversion in Expressions in C++
When an expression has mixed type variables, the compiler automatically does the type conversion according to operation and data type of operands. For example, consider the following code snippet:
int a = 2, b = 3, c = 5;
double f = 4.5;
double res = a + b / f + c;
In the last line of code, we can see that three variables are type int, and one is type double. According to the operator precedence, /
will be the first evaluated; in this expression, b/f
means an int is divided by a double, so the result will be converted to double.
After that, +
will be evaluated for int and double, so the result will be converted to double. Hence, the res
will be of double datatype.
Here we can see that the result of the expression is always promoted to higher data types that are involved in the expression.
int + double = > double + double = double int* double =
> double* double = double double* int =
> double* double = double int / double =
> double / double =
double double / int =
> double / double =
double int / int = int
You can see in the expressions above how the types are promoted to higher data types in the case of mixed-type expressions.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn