How to Overload the == Operator in C++
- Operator Overloading in C++
-
Overload the
==
Operator in C++ -
Overload the
==
Operator in Class Hierarchy
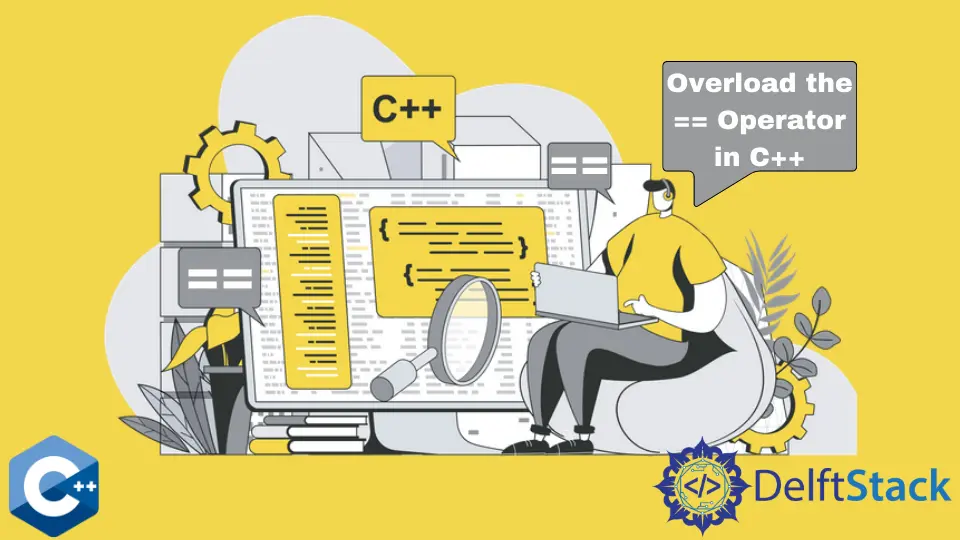
This article will discuss the basics of operator overloading and provides an easy implementation of the ==
operator in a class.
Operator Overloading in C++
Operator overloading refers to changing how an operator must act when used along with the user-defined datatypes like class objects and structs. These are like member functions in a class called upon to use that operator.
For example, if we have a class of Student
and we want to check which students’ marks are greater than the other, then we can overload the >
operator that can compare the marks
data member of the two objects and return the result.
The syntax for operator overloading is as follows:
class className {
public:
returnType operator operatorSymbol([list of arguments]) {
// definition
}
};
Note that operator
is a keyword used for operator overloading, and after that, we specify the symbol of the operator that needs to be overloaded. Remember that we cannot overload operators for fundamental data types like int, char, float, etc.
Almost all the operators can be overloaded except for a few that are not allowed to be overloaded. These operators include:
- the
sizeof
operator - the
typeid
operator - the scope resolution
(::)
- the class member access operators
(.(dot), .*
(pointer to member operator) - the conditional Operator
(?:)
Overload the ==
Operator in C++
==
is also the equal to
operator that falls under the comparison operators classification and returns a Boolean result of true
or false
. It determines whether the two operands on the left and right sides of the operator are equal to each other.
For user-defined data types like class, this operator can be overloaded to compare two objects of a class to check if all the data members of the two objects are equal or not.
Consider a class Employee
having the following data members:
class Employee {
private:
string name;
int salary;
};
In this class, we can overload the ==
operator to check if the two employees’ objects’ are equal to each other or not. This function can be implemented as:
bool operator==(const Employee &emp) {
if (name == emp.name && salary == emp.salary) return true;
return false;
}
Now, we can put them all together to have a complete class.
#include <iostream>
#include <string>
using namespace std;
class Employee {
private:
string name;
int salary;
public:
Employee(string n, int s) {
name = n;
salary = s;
}
bool operator==(const Employee &emp) {
if (name == emp.name && salary == emp.salary) return true;
return false;
}
};
int main() {
Employee e1("David", 1000);
Employee e2("David", 1000);
if (e1 == e2)
cout << "Equal" << endl;
else
cout << "Not Equal" << endl;
}
Output:
Equal
Overload the ==
Operator in Class Hierarchy
If you have multiple classes, such that some classes are a parent and some are child classes, then you need to implement the operator function in all the subclasses and superclasses. For example, if there is a class A
and you implement the ==
operator, this overloaded operator will be available for all its subclasses.
class A {
public:
int foo;
bool operator==(const A &a) { return foo == a.foo; }
};
Now, we have another class, B
, a subclass of A
.
class B : public A {
public:
int bar;
};
If we do not implement the ==
operator in this class, then it will call the operator function of the base class, but the problem is that the base class is comparing only the data members of its own but not that of the B
class. Therefore, we also need to implement the operator in the child class.
After that implementation, the base class implementation will not be called until we call it explicitly. Hence, we call that function explicitly to compare the base class’s data members.
This is demonstrated in the code snippet below:
class B : public A {
public:
int bar;
bool operator==(const B &b) {
if (!A::operator==(static_cast<const A &>(b))) {
return false;
}
return bar == b.bar;
}
};
The driver function will be:
int main() {
B b;
b.bar = 2;
b.foo = 1;
B b2;
b2.bar = 2;
b2.foo = 1;
if (b == b2) {
cout << "Equal" << endl;
}
}
Output:
Equal
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn