在 C++ 中重载 == 运算符
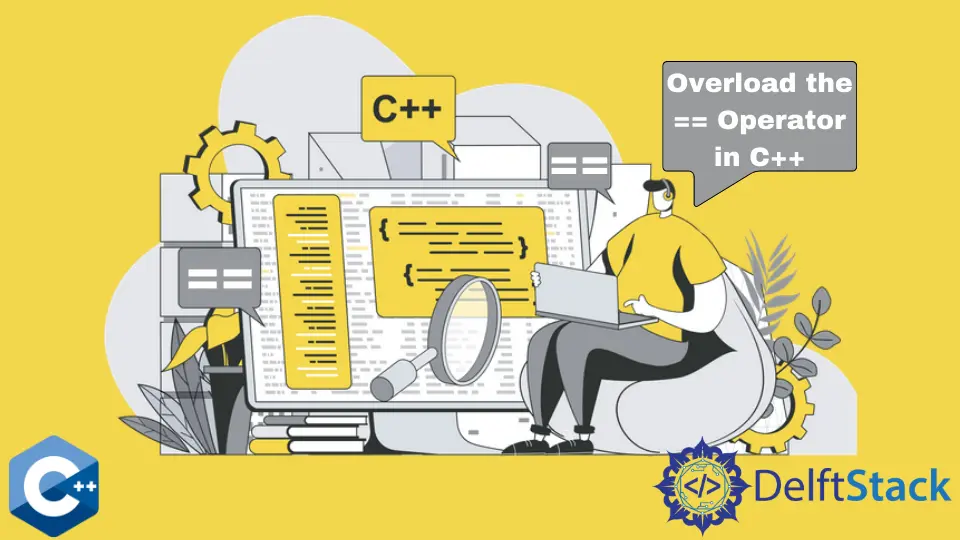
本文将讨论运算符重载的基础知识,并提供类中 ==
运算符的简单实现。
C++ 中的运算符重载
运算符重载是指更改运算符在与用户定义的数据类型(如类对象和结构)一起使用时的行为方式。这些类似于调用该运算符的类中的成员函数。
例如,如果我们有一个 Student
类,并且我们想检查哪些学生的分数高于另一个,那么我们可以重载 >
运算符,该运算符可以比较两个对象的 marks
数据成员,并且返回结果。
运算符重载的语法如下:
class className {
public:
returnType operator operatorSymbol([list of arguments]) {
// definition
}
};
注意 operator
是用于运算符重载的关键字,之后我们指定需要重载的运算符的符号。请记住,对于 int、char、float 等基本数据类型,我们不能重载运算符。
除了少数不允许重载的运算符外,几乎所有运算符都可以重载。这些运算符包括:
sizeof
运算符typeid
运算符- 范围解析
(::)
- 类成员访问运算符
(.(dot), .*
(指向成员运算符的指针) - 条件运算符
(?:)
在 C++ 中重载 ==
运算符
==
也是 equal to
运算符,属于比较运算符分类并返回 true
或 false
的布尔结果。判断运算符左右两侧的两个操作数是否相等。
对于类等用户定义的数据类型,可以重载该运算符来比较一个类的两个对象,以检查两个对象的所有数据成员是否相等。
考虑一个具有以下数据成员的类 Employee
:
class Employee {
private:
string name;
int salary;
};
在这个类中,我们可以重载 ==
运算符来检查两个员工的对象是否相等。这个函数可以实现为:
bool operator==(const Employee &emp) {
if (name == emp.name && salary == emp.salary) return true;
return false;
}
现在,我们可以把它们放在一起来组成一个完整的类。
#include <iostream>
#include <string>
using namespace std;
class Employee {
private:
string name;
int salary;
public:
Employee(string n, int s) {
name = n;
salary = s;
}
bool operator==(const Employee &emp) {
if (name == emp.name && salary == emp.salary) return true;
return false;
}
};
int main() {
Employee e1("David", 1000);
Employee e2("David", 1000);
if (e1 == e2)
cout << "Equal" << endl;
else
cout << "Not Equal" << endl;
}
输出:
Equal
在类层次结构中重载 ==
运算符
如果你有多个类,有些类是父类,有些是子类,那么你需要在所有的子类和超类中实现操作符函数。例如,如果有一个类 A
并且你实现 ==
运算符,则此重载运算符将可用于其所有子类。
class A {
public:
int foo;
bool operator==(const A &a) { return foo == a.foo; }
};
现在,我们有另一个类 B
,它是 A
的子类。
class B : public A {
public:
int bar;
};
如果我们没有在这个类中实现 ==
运算符,那么它会调用基类的运算符函数,但问题是基类只比较自己的数据成员,而不是 B
类。因此,我们还需要在子类中实现操作符。
在该实现之后,在我们显式调用它之前不会调用基类实现。因此,我们显式调用该函数来比较基类的数据成员。
这在下面的代码片段中得到了证明:
class B : public A {
public:
int bar;
bool operator==(const B &b) {
if (!A::operator==(static_cast<const A &>(b))) {
return false;
}
return bar == b.bar;
}
};
驱动函数将是:
int main() {
B b;
b.bar = 2;
b.foo = 1;
B b2;
b2.bar = 2;
b2.foo = 1;
if (b == b2) {
cout << "Equal" << endl;
}
}
输出:
Equal
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn