How to Use the Modulo Operator in C++
- Understanding the Modulo Operator
- Using Modulo to Check Even or Odd Numbers
- Implementing Cyclic Behavior with Modulo
- Using Modulo in Algorithms
- Conclusion
- FAQ
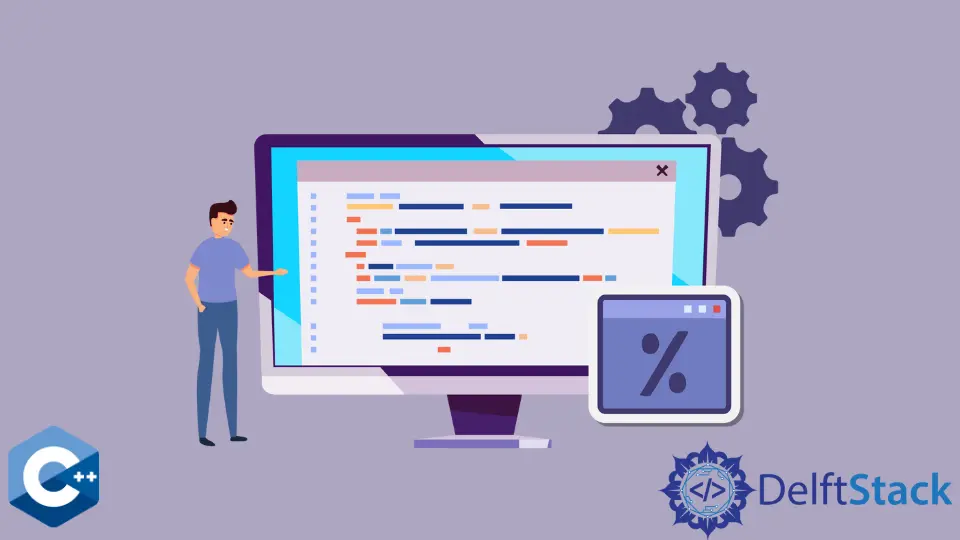
The modulo operator is a fundamental tool in programming that allows you to find the remainder of a division operation. In C++, this operator is represented by the percentage symbol (%). Understanding how to effectively use the modulo operator can help you solve various problems, such as determining even or odd numbers, implementing cyclic behaviors, and more.
In this article, we’ll dive into the practical applications of the modulo operator in C++, providing you with clear examples and explanations that will enhance your coding skills. Whether you’re a beginner or looking to refresh your knowledge, this guide will equip you with the insights you need to use the modulo operator confidently.
Understanding the Modulo Operator
The modulo operator is primarily used to determine the remainder of a division between two integers. For instance, if you divide 10 by 3, the quotient is 3, and the remainder is 1. Hence, 10 % 3 equals 1. This operator is particularly useful in various programming scenarios, such as checking if a number is even or odd, cycling through array indices, and implementing certain algorithms.
In C++, the modulo operator can only be used with integer types. When applied to negative numbers, the behavior can be a bit tricky, so it’s essential to be aware of how it functions in such cases. Here’s a simple example:
#include <iostream>
int main() {
int a = 10;
int b = 3;
int result = a % b;
std::cout << "10 % 3 = " << result << std::endl;
return 0;
}
Output:
10 % 3 = 1
In this code, we declare two integers, a
and b
, and compute the modulo of a
by b
. The result is printed to the console, demonstrating how the modulo operator works in a straightforward manner.
Using Modulo to Check Even or Odd Numbers
One of the most common applications of the modulo operator is to determine whether a number is even or odd. An even number will yield a remainder of 0 when divided by 2, while an odd number will yield a remainder of 1. This simple check can be implemented efficiently in C++. Let’s see how this works with a practical example:
#include <iostream>
int main() {
int number;
std::cout << "Enter an integer: ";
std::cin >> number;
if (number % 2 == 0) {
std::cout << number << " is even." << std::endl;
} else {
std::cout << number << " is odd." << std::endl;
}
return 0;
}
Output:
Enter an integer: 5
5 is odd.
In this example, we prompt the user to enter an integer. The program then checks if the number is even or odd using the modulo operator. If the result of number % 2
equals 0, it confirms that the number is even; otherwise, it is odd. This is a practical implementation that you can use in various applications.
Implementing Cyclic Behavior with Modulo
Another interesting use of the modulo operator is to create cyclic behavior in your programs. For example, if you want to cycle through the indices of an array, the modulo operator can help you wrap around when you exceed the array’s bounds. This is particularly useful in scenarios such as round-robin scheduling or game mechanics where you need to loop through a set of values repeatedly. Here’s how you can implement this:
#include <iostream>
int main() {
int arr[] = {10, 20, 30, 40, 50};
int size = sizeof(arr) / sizeof(arr[0]);
for (int i = 0; i < 10; i++) {
int index = i % size;
std::cout << "Element at index " << index << ": " << arr[index] << std::endl;
}
return 0;
}
Output:
Element at index 0: 10
Element at index 1: 20
Element at index 2: 30
Element at index 3: 40
Element at index 4: 50
Element at index 0: 10
Element at index 1: 20
Element at index 2: 30
Element at index 3: 40
Element at index 4: 50
In this example, we have an array of five integers. The loop runs ten times, but the modulo operator ensures that the index wraps around when it exceeds the size of the array. As a result, we see the elements printed in a cyclic manner. This technique is invaluable when dealing with scenarios that require repetitive access to a limited set of values.
Using Modulo in Algorithms
The modulo operator is also a key component in various algorithms, particularly in hashing and random number generation. For instance, when implementing a hash function, you often use the modulo operator to ensure that the hash value fits within a specific range. This is crucial for data structures like hash tables, where maintaining a balance in data distribution is essential. Here’s a simple example of how you might use the modulo operator in a hashing scenario:
#include <iostream>
int hashFunction(int key, int tableSize) {
return key % tableSize;
}
int main() {
int tableSize = 10;
for (int i = 0; i < 20; i++) {
std::cout << "Hash for " << i << " is: " << hashFunction(i, tableSize) << std::endl;
}
return 0;
}
Output:
Hash for 0 is: 0
Hash for 1 is: 1
Hash for 2 is: 2
Hash for 3 is: 3
Hash for 4 is: 4
Hash for 5 is: 5
Hash for 6 is: 6
Hash for 7 is: 7
Hash for 8 is: 8
Hash for 9 is: 9
Hash for 10 is: 0
Hash for 11 is: 1
Hash for 12 is: 2
Hash for 13 is: 3
Hash for 14 is: 4
Hash for 15 is: 5
Hash for 16 is: 6
Hash for 17 is: 7
Hash for 18 is: 8
Hash for 19 is: 9
In this code snippet, we define a simple hash function that takes a key and a table size as input. The modulo operator ensures that the hash value stays within the bounds of the hash table’s size. This is a critical concept in computer science, especially when working with large datasets and requiring efficient data retrieval.
Conclusion
In conclusion, the modulo operator is a versatile and powerful tool in C++. Whether you are checking for even and odd numbers, implementing cyclic behaviors, or utilizing it in algorithms, mastering the modulo operator can significantly enhance your programming capabilities. By understanding its applications and practicing its use, you can tackle a wide range of problems with confidence. So, the next time you find yourself in a situation where you need to determine remainders, remember the modulo operator and the insights shared in this article.
FAQ
-
What is the modulo operator in C++?
The modulo operator (%) in C++ is used to find the remainder of a division between two integers. -
Can the modulo operator be used with floating-point numbers?
No, the modulo operator can only be used with integer types in C++. -
What happens when you use the modulo operator with negative numbers?
Using the modulo operator with negative numbers can yield results that may vary based on the implementation, so it’s important to understand how your specific compiler handles it. -
How can I use the modulo operator to cycle through an array?
You can use the modulo operator to ensure that the index does not exceed the array size, allowing you to loop back to the beginning when necessary. -
Is the modulo operator useful in algorithms?
Yes, the modulo operator is often used in algorithms for tasks such as hashing and random number generation, helping to maintain data within specified bounds.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook