Modulus for Negative Numbers in C++
- Remainder vs. Modulus
-
the
%
Operator in C++ -
Implementation of
%
in C++ -
the
%
Operator in Python -
Implementation of
%
in Python - Methods to Achieve Python’s Equivalent Modulus in C++
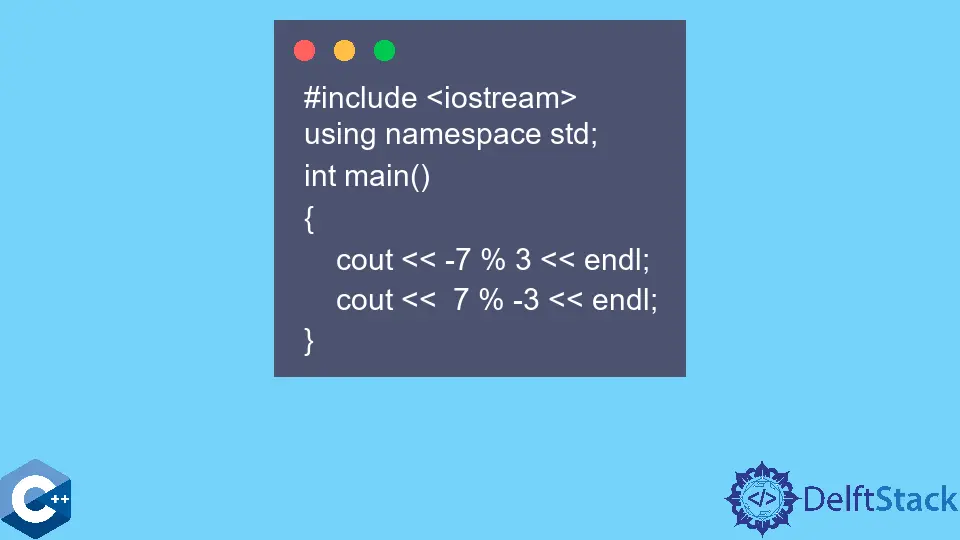
In this tutorial, we will discover the differences between remainder and modulus. We will learn about the fundamentals of the %
operator.
Later, we will learn how the %
operator behaves in Python and C++. Finally, we will summarize this article by discussing a few ways to implement modulus functionality in C++.
Remainder vs. Modulus
The exact behavior of the %
sign may vary across different programming languages. The operator frequently produces surprising outcomes that leave us confused.
Does it create a modulus or a remainder? Before examining its behavior in programming languages, let us first clarify how they differ.
Remainder
The remainder is simply the part that remains after the arithmetic division of two integer numbers. Either positive or negative, the sign for the remainder is always determined by the sign of the dividend.
Check the examples below to understand this clearly.
Examples:
-
7 % 3 = 1
Here dividend is 7, which is positively signed, so the result will also be positively signed.
-
-7 % 3 = -1
Here dividend is -7, which is negatively signed, so the result will also be negatively signed.
-
7 % -3 = 1
Here dividend is 7, which is positively signed, so the result will also be positively signed.
-
-7 % -3 = -1
Here dividend is -7, which is negatively signed, so the result will also be negatively signed.
Modulus
The modulus is the sum of the remainder and divisor when they are oppositely signed, and the remaining part after arithmetic division, when the remainder and divisor are both of the same sign.
The modulus will have the same sign as the divisor. Check the examples below to understand this clearly.
Examples:
-
5 % 3 = 2
Here both divisor and remainder are positively signed, so the remainder will also be positively signed.
-
-5 % 3 = 1
Here, divisor and remainder are of the opposite sign so that the result will be the sum of remainder and divisor. i.e. -2 + 3 = 1
-
5 % -3 = -1
Here both divisor and remainder are opposite signs. The result will be the sum of the remainder and divisor. i.e. 2 + -3 = -1
-
-5 % -3 = -2
Here both divisor and remainder are of the same sign so that the result will be the same as the remainder.
It is clear from the above examples that modulus and remainder are the same if both dividend and divisor are positive. Both are different in the case of negative numbers.
By this point, we hope it is clear how remainder and modulus differ. Now let’s talk about the behavior of the %
operator in Python and C++.
the %
Operator in C++
In C++, the %
is known as the modulo operator. It is an arithmetic operator.
It is used to compute the remainder that results from performing integer division. Although it has the name “modulo”, the important thing to remember is that it doesn’t provide the modulus.
In C++, the %
symbol only returns the remainder. Check the example below.
#include <iostream>
using namespace std;
int main() {
cout << -7 % 3 << endl;
cout << 7 % -3 << endl;
}
Output:
-1
1
As the output suggests, the %
operator doesn’t give us modulus. It is just returning a remainder.
Implementation of %
in C++
Let’s see the implementation of %
in C++. The remainder in C++ is returned according to the equation below.
remainder = a - ( b * trunc ( a / b ) )
Let us develop our logic for calculating the remainder using the equation above without the %
operator.
#include <iostream>
using namespace std;
int calRemainder(int a, int b) {
int q = a / b;
return a - (b * q);
}
int main() {
int a = -7, b = 3;
cout << calRemainder(a, b) << endl;
}
Output:
-1
the %
Operator in Python
In Python, the %
is known as the modulo operator. The modulo operator is considered an arithmetic operation in Python.
It is used to compute the modulus
that results from performing integer division. This is where Python differs from C++.
When we use the modulo operator in C++, it returns the remainder, whereas Python returns the modulus. Check the examples below to observe the behavior of the modulo operator in Python.
print(-7 % 3)
print(7 % -3)
Output:
2
-2
In the first example, -7 % 3
gives us the remainder -1. As the remainder (-1) and divisor (3) are of opposite signs, their sum will give us the modulus. i.e. -1 + 3 = 2
.
The same is true for example 2.
Implementation of %
in Python
Let’s see the implementation of %
in Python. The modulus in Python is returned according to the equation below.
modulus = a - ( b * floor( a / b ) )
Let us develop our logic for calculating the modulus using the equation above without the %
operator.
from math import floor
def calModulus(a, b):
q = floor(a / b)
return a - b * q
print(calModulus(-7, 3))
Output:
2
Methods to Achieve Python’s Equivalent Modulus in C++
We have examined how remainder and modulus differ and how the %
operator behaves in Python and C++. In Python, you will always get non-negative values when you use %
with a positive divisor.
Most of the time, we work with positive divisors, and we need the functionality of the modulus and not just the remainder. Modulus always returns positive values if the divisor is positively signed.
Let us look at different techniques for achieving this behavior in C++.
Method 1: Implement an Equation
We can achieve the Python modulus behavior by implementing the equation modulus = a - ( b * floor( a / b ) )
in C++.
#include <math.h>
#include <iostream>
using namespace std;
int getModulus(int dividend, int divisor) {
// Type casting is necessary as (int)/(int) will give int result, i.e. -5 / 2
// will give -1 and not -2.5
int t = (int)floor((double)dividend / divisor);
return dividend - divisor * t;
}
int main() { cout << getModulus(-8, 3); }
Output:
1
Method 2: Add the Remainder and Divisor
Another way to achieve the modulus functionality in C++ is by adding the remainder and divisor if they are oppositely signed.
We assume that our divisor is positively signed, so whenever we get a negative remainder, we add it to the divisor to get the modulus value.
#include <iostream>
using namespace std;
int getModulus(int dividend, int divisor) {
int t = dividend % divisor;
return t >= 0 ? t : t + divisor;
}
int main() { cout << getModulus(-7, 3); }
Output:
2