How to Check if Element Exists in C++ Vector
-
C++
std::find()
Algorithm to Check if Element Exists in Vector -
C++ Range-Based
for
Loop to Check if Element Exists in Vector -
C++
any_of()
Algorithm to Check if Element Exists in Vector
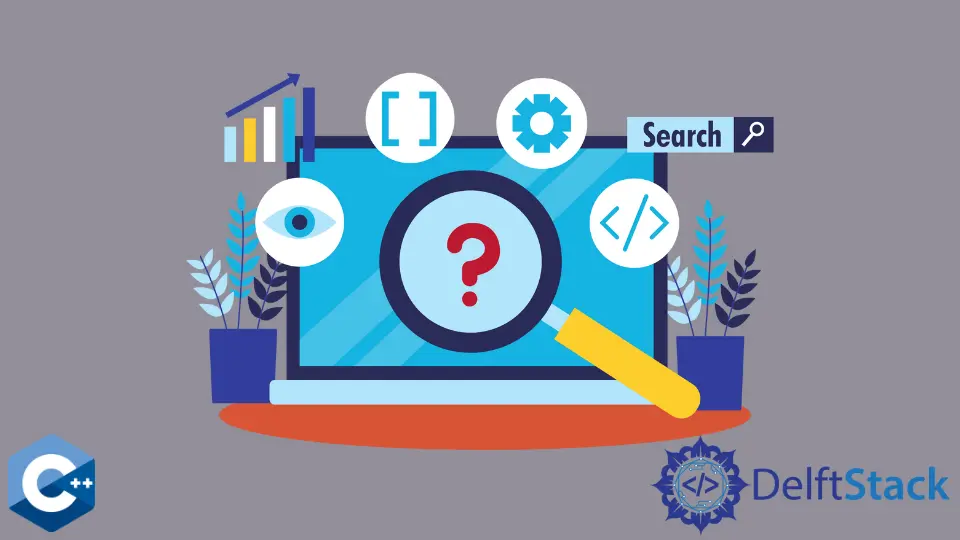
This article demonstrates multiple methods of how you can check if an element exists in a C++ vector.
C++ std::find()
Algorithm to Check if Element Exists in Vector
The find
method is a part of the STL algorithm library; it can check if the given element exists in a particular range. The function searches for a factor that’s equal to the third parameter passed by the user. The corresponding return value is the iterator to the first element found, or in case none is found, the end of the range is returned.
Notice that we use the *
operator to access the returned string value and make a comparison condition in the if
statement, as shown in the following example.
#include <algorithm>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::find;
using std::string;
using std::vector;
int main() {
string element_to_check1 = "nibble";
string element_to_check2 = "nimble";
vector<string> data_types = {"bit", "nibble", "byte", "char",
"int", "long", "long long", "float",
"double", "long double"};
if (*find(data_types.begin(), data_types.end(), element_to_check1) ==
element_to_check1) {
printf("%s is present in the vector\n", element_to_check1.c_str());
} else {
printf("%s is not present in the vector\n", element_to_check1.c_str());
}
if (*find(data_types.begin(), data_types.end(), element_to_check2) ==
element_to_check2) {
printf("%s is present in the vector\n", element_to_check2.c_str());
} else {
printf("%s is not present in the vector\n", element_to_check2.c_str());
}
return EXIT_SUCCESS;
}
Output:
nibble is present in the vector
nimble is not present in the vector
C++ Range-Based for
Loop to Check if Element Exists in Vector
A range-based for
loop can be used as another solution to check if a given element is present in the vector. This method is relatively straightforward because it iterates through the vector; each iteration checks for equality with a given string. If an element matches, a confirmation string is printed, and the loop stops using the break
statement.
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string element_to_check = "nibble";
vector<string> data_types = {"bit", "nibble", "byte", "char",
"int", "long", "long long", "float",
"double", "long double"};
for (const auto &item : data_types) {
if (item == element_to_check) {
printf("%s is present in the vector\n", element_to_check.c_str());
break;
}
}
return EXIT_SUCCESS;
}
Output:
nibble is present in the vector
C++ any_of()
Algorithm to Check if Element Exists in Vector
Another useful method from the <algorithm>
header, which is similar to the find
method, is the any_of
algorithm. The any_of
method checks if the unary predicate, which is specified as the third argument, returns true
for at least one element in a given range. In this example, we use a lambda expression to construct a unary predicate for comparing vector elements.
#include <algorithm>
#include <iostream>
#include <vector>
using std::any_of;
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string element_to_check = "nibble";
vector<string> data_types = {"bit", "nibble", "byte", "char",
"int", "long", "long long", "float",
"double", "long double"};
if (any_of(data_types.begin(), data_types.end(),
[&](const string& elem) { return elem == element_to_check; })) {
printf("%s is present in the vector\n", element_to_check.c_str());
}
return EXIT_SUCCESS;
}
Output:
nibble is present in the vector
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook