Custom Exception in C++
- Understanding Custom Exceptions
- Throwing Custom Exceptions
- Catching Custom Exceptions
- Best Practices for Custom Exceptions
- Conclusion
- FAQ
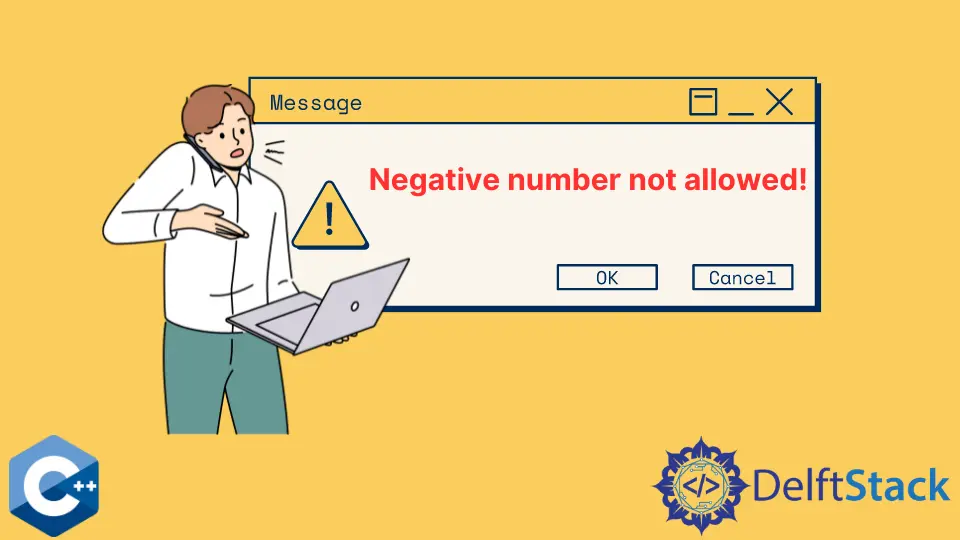
Creating robust applications often requires handling unexpected situations gracefully. In C++, one effective way to manage these scenarios is through custom exceptions. This programming tutorial will guide you through the implementation of custom exception classes in C++.
By the end of this article, you will understand how to create your own exception classes, throw exceptions, and catch them as needed. Custom exceptions not only improve the readability of your code but also provide meaningful error messages that can help you debug your applications efficiently. So, let’s dive into the world of C++ exceptions and learn how to implement them effectively.
Understanding Custom Exceptions
In C++, exceptions are a way to signal that an error has occurred. The standard library provides built-in exceptions, but sometimes you need more specific handling tailored to your application. This is where custom exceptions come into play. A custom exception class allows you to define specific error types, making your code cleaner and easier to maintain.
To create a custom exception, you typically derive a new class from the std::exception
base class. This custom class can then encapsulate additional information about the error, such as error codes or messages. Here’s how you can implement a basic custom exception class:
#include <iostream>
#include <exception>
#include <string>
class CustomException : public std::exception {
private:
std::string message;
public:
CustomException(const std::string& msg) : message(msg) {}
const char* what() const noexcept override {
return message.c_str();
}
};
In this code snippet, we define a CustomException
class that inherits from std::exception
. It has a constructor that accepts a message, which is stored in a private member variable. The what()
method is overridden to return this message when the exception is caught.
The what()
method is particularly important. It provides a way to retrieve the error message when the exception is caught, allowing developers to understand the nature of the error quickly.
Throwing Custom Exceptions
Once you have defined your custom exception class, the next step is to throw it when an error condition arises. This is done using the throw
keyword. Let’s see how you can throw a custom exception in your code.
void riskyFunction(int value) {
if (value < 0) {
throw CustomException("Negative value not allowed");
}
// Continue processing
}
int main() {
try {
riskyFunction(-1);
} catch (const CustomException& e) {
std::cout << "Caught an exception: " << e.what() << std::endl;
}
return 0;
}
In this example, the riskyFunction
checks if the input value is negative. If it is, a CustomException
is thrown with a specific error message. The main
function calls riskyFunction
within a try
block, catching the exception if it occurs.
Output:
Caught an exception: Negative value not allowed
This approach allows you to handle errors gracefully. By throwing a custom exception, you can provide specific feedback about what went wrong, improving the overall robustness of your application.
Catching Custom Exceptions
Catching exceptions is just as important as throwing them. In C++, you can catch exceptions using the catch
block. It’s essential to catch exceptions by reference to avoid slicing issues and ensure that the correct type of exception is caught. Here’s how you can catch custom exceptions effectively:
void anotherRiskyFunction(int value) {
if (value == 0) {
throw CustomException("Division by zero");
}
std::cout << "Result: " << (10 / value) << std::endl;
}
int main() {
try {
anotherRiskyFunction(0);
} catch (const CustomException& e) {
std::cout << "Caught an exception: " << e.what() << std::endl;
}
return 0;
}
In this code, anotherRiskyFunction
checks if the input value is zero before attempting to divide a number. If it is zero, a CustomException
is thrown. The main
function again utilizes a try
block to catch and handle the exception.
Output:
Caught an exception: Division by zero
By catching the exception, you can provide meaningful feedback to the user or log the error for further analysis. This practice enhances the user experience and helps developers maintain the code more effectively.
Best Practices for Custom Exceptions
When implementing custom exceptions, there are several best practices to keep in mind. Firstly, ensure that your custom exception classes are derived from std::exception
to maintain compatibility with the C++ exception handling model. Secondly, provide meaningful messages in your exceptions. This helps during debugging and allows developers to understand the context of the error quickly.
Additionally, consider creating a hierarchy of exceptions. For instance, you could have a base exception class and derive more specific exceptions from it. This approach allows you to catch specific exceptions without losing the ability to catch all exceptions at a higher level.
Lastly, document your exceptions. Make it clear in your code or documentation what types of exceptions can be thrown and under what conditions. This information is invaluable for other developers who may work with your code in the future.
Conclusion
Custom exceptions in C++ are a powerful tool for managing errors in your applications. By defining your own exception classes, throwing them when necessary, and catching them effectively, you can create robust and maintainable code. Remember to follow best practices, such as providing meaningful messages and creating a hierarchy of exceptions. With these techniques, you’ll be well-equipped to handle unexpected situations in your C++ applications.
FAQ
-
what are custom exceptions in C++?
Custom exceptions are user-defined exception classes that allow developers to handle specific error conditions in a more meaningful way than standard exceptions. -
how do you create a custom exception class in C++?
You create a custom exception class by inheriting from thestd::exception
class and overriding thewhat()
method to provide a custom error message. -
when should you use custom exceptions?
Use custom exceptions when standard exceptions do not provide sufficient information about the error or when you need to handle specific error conditions in your application. -
can you catch multiple exceptions in C++?
Yes, you can catch multiple exceptions by using multiplecatch
blocks, each designed to handle different types of exceptions. -
what is the importance of the
what()
method in custom exceptions?
Thewhat()
method returns a C-style string that describes the exception, providing essential information for debugging and error handling.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn