How to Throw Exception in C++
- Understanding Exceptions in C++
- Throwing Built-in Exceptions
- Creating Custom Exception Classes
- Best Practices for Using Exceptions
- Conclusion
- FAQ
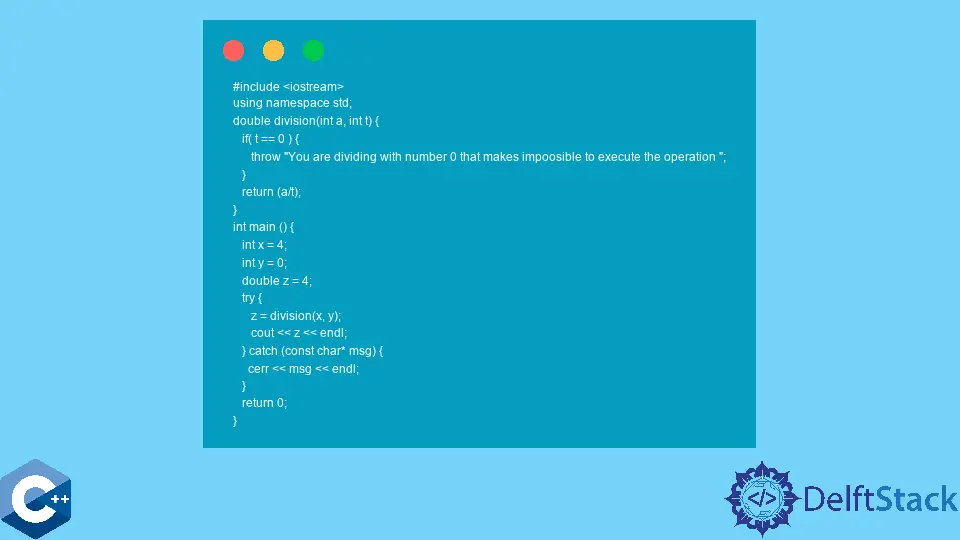
C++ throw exception is a powerful feature of C++ that can be used to handle errors and unexpected events. It is mainly used in the program to terminate the program’s execution, allowing developers to manage errors gracefully. Understanding how to throw exceptions in C++ is crucial for building robust applications.
In this article, we will explore the mechanics of throwing exceptions, examine the different types of exceptions, and provide practical examples to illustrate how you can effectively manage errors in your C++ programs.
Understanding Exceptions in C++
Exceptions in C++ are a mechanism for signaling and handling errors. Instead of the program crashing or producing incorrect results, exceptions allow you to catch errors and handle them in a controlled manner. When an exception is thrown, the normal flow of the program is disrupted, and the control is transferred to a special block of code designed to handle the exception.
To throw an exception, you typically use the throw
keyword followed by an instance of an exception class. This can be a built-in exception type like std::runtime_error
or a user-defined exception type. The thrown exception can then be caught using a try-catch
block, which allows you to define how your program should respond to specific errors.
Throwing Built-in Exceptions
One of the simplest ways to throw an exception in C++ is by using built-in exception classes. The C++ Standard Library provides several predefined exception classes that you can use directly. For example, you can throw a std::runtime_error
when an invalid operation occurs.
Here’s a simple example demonstrating how to throw a built-in exception:
#include <iostream>
#include <stdexcept>
void riskyFunction(int x) {
if (x < 0) {
throw std::runtime_error("Negative value encountered");
}
std::cout << "Value is: " << x << std::endl;
}
int main() {
try {
riskyFunction(-5);
} catch (const std::runtime_error& e) {
std::cerr << "Caught an exception: " << e.what() << std::endl;
}
return 0;
}
Output:
Caught an exception: Negative value encountered
In this example, the riskyFunction
checks if the input value x
is negative. If it is, a std::runtime_error
is thrown with a descriptive message. The main
function then uses a try-catch
block to handle the exception. When the exception is caught, the error message is printed to the standard error output.
Throwing built-in exceptions is straightforward and allows for quick error handling. It’s an effective way to signal problems without complicating your code with extensive error-checking logic.
Creating Custom Exception Classes
While built-in exceptions are handy, sometimes you need more specific error handling that the standard exceptions do not cover. In such cases, you can create your own custom exception classes. This approach allows you to define exception types that are tailored to your application’s needs.
Here’s how you can create and throw a custom exception:
#include <iostream>
#include <exception>
class MyException : public std::exception {
public:
const char* what() const noexcept override {
return "My custom exception occurred";
}
};
void testFunction(bool trigger) {
if (trigger) {
throw MyException();
}
std::cout << "Function executed successfully." << std::endl;
}
int main() {
try {
testFunction(true);
} catch (const MyException& e) {
std::cerr << "Caught an exception: " << e.what() << std::endl;
}
return 0;
}
Output:
Caught an exception: My custom exception occurred
In this code, we define a custom exception class called MyException
that inherits from std::exception
. The what()
method is overridden to provide a custom error message. The testFunction
throws MyException
when the trigger
parameter is true. Again, we handle the exception in the main
function using a try-catch
block.
Creating custom exceptions enhances the readability of your code and allows you to categorize errors more effectively. It also makes your error handling more flexible and specific to your application context.
Best Practices for Using Exceptions
When using exceptions in C++, there are several best practices to keep in mind. Following these practices can help you write cleaner, more maintainable code.
-
Use exceptions for exceptional cases only: Exceptions should not be used for regular control flow. Reserve them for situations that are truly exceptional and cannot be handled through normal means.
-
Catch exceptions by reference: Always catch exceptions by reference to avoid slicing and to ensure that the complete exception object is preserved.
-
Avoid throwing exceptions from destructors: If an exception is thrown during the destruction of an object, it can lead to program termination. Instead, use other mechanisms to handle errors in destructors.
-
Document your exceptions: Clearly document the exceptions that your functions can throw. This helps other developers understand how to use your code effectively.
-
Use standard exceptions when possible: Leverage the built-in exception classes provided by the C++ Standard Library. They are well-defined and widely understood.
By adhering to these best practices, you can enhance the quality and reliability of your C++ applications, making your error handling more robust and effective.
Conclusion
Throwing exceptions in C++ is a fundamental aspect of error handling that allows developers to manage unexpected situations gracefully. By understanding how to throw both built-in and custom exceptions, as well as following best practices, you can create robust applications that handle errors effectively. Whether you’re a beginner or an experienced developer, mastering exception handling is essential for writing high-quality C++ code.
FAQ
-
What is an exception in C++?
An exception in C++ is a mechanism used to signal and handle errors or unexpected events that occur during program execution. -
How do I throw an exception in C++?
You can throw an exception using thethrow
keyword followed by an instance of an exception class. -
What are built-in exceptions in C++?
Built-in exceptions are predefined exception classes in the C++ Standard Library, such asstd::runtime_error
, that you can use to signal errors.
-
Can I create custom exceptions in C++?
Yes, you can create custom exception classes by inheriting fromstd::exception
and overriding thewhat()
method. -
What are some best practices for handling exceptions in C++?
Best practices include using exceptions for exceptional cases only, catching exceptions by reference, avoiding exceptions in destructors, and documenting your exceptions.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook