How to Solve Control Reaches End of Non-Void Function Error in C++
- Understanding the Error
- Ensuring All Paths Return a Value
- Using Default Return Values
- Debugging with Compiler Warnings
- Conclusion
- FAQ
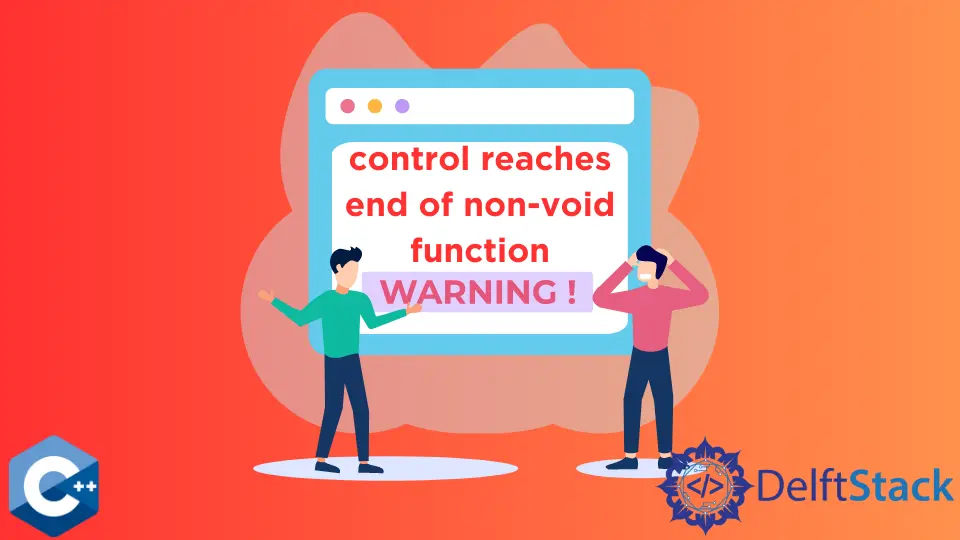
When programming in C++, you may encounter the frustrating “control reaches end of non-void function” error. This error occurs when a function that is supposed to return a value does not have a return statement at the end. It’s a common pitfall for both novice and experienced programmers. Understanding how to resolve this error is crucial for maintaining the flow of your code and ensuring it runs smoothly.
In this article, we will explore effective strategies to fix this issue, along with practical code examples to illustrate each solution. By the end, you’ll be equipped with the knowledge to tackle this error confidently.
Understanding the Error
Before diving into solutions, it’s essential to grasp what this error means. In C++, functions can be categorized as either void or non-void. A non-void function is expected to return a value of a specified type. If the control flow reaches the end of such a function without encountering a return statement, the compiler raises an error. This often happens in conditional structures where the return statement might be inadvertently omitted.
For example, consider the following function:
int add(int a, int b) {
if (a > b) {
return a + b;
}
}
In this case, if a
is not greater than b
, the function reaches the end without returning a value, triggering the error.
Ensuring All Paths Return a Value
One of the most effective ways to resolve the “control reaches end of non-void function” error is to ensure that all possible execution paths in your function return a value. This means examining your code thoroughly to identify any scenarios where the function might exit without providing a return statement.
Here’s an updated version of the previous example that resolves the issue:
int add(int a, int b) {
if (a > b) {
return a + b;
} else {
return a - b; // Added return statement for the else case
}
}
In this revised function, we’ve added an else
clause that guarantees a return value, regardless of the input. This way, the function will always return an integer, satisfying the compiler’s requirements.
Output:
The function returns the sum if a is greater than b, otherwise it returns the difference.
By ensuring that every possible path through the function leads to a return statement, you eliminate the risk of this error. This practice not only resolves the issue but also makes your code more robust and easier to read.
Using Default Return Values
Another method to address the “control reaches end of non-void function” error is to use a default return value. This approach is particularly useful when you want to provide a fallback in case none of the conditions are met.
Consider the following example:
int multiply(int a, int b) {
if (a == 0 || b == 0) {
return 0; // Early return for zero cases
} else if (a < 0 || b < 0) {
return -1; // Return -1 for negative values
}
return a * b; // Main return for positive values
}
In this function, we handle special cases for zero and negative values, ensuring that all paths return a value. If neither condition is met, the function returns the product of a
and b
.
Output:
Returns 0 if either number is 0, -1 for negative values, or the product otherwise.
Using default return values can simplify your code and make it more maintainable. It also helps avoid the dreaded error by ensuring that there’s always a return statement executed, regardless of the input.
Debugging with Compiler Warnings
Sometimes, the error can be elusive, especially in complex functions. In such cases, enabling compiler warnings can be a valuable debugging tool. Most modern C++ compilers provide warning flags that can help you identify potential issues before they lead to runtime errors.
For instance, when using g++
, you can compile your code with the -Wall
flag to enable all warnings:
g++ -Wall your_code.cpp -o your_program
This command will compile your program and display any warnings, including those related to missing return statements. By paying attention to these warnings, you can proactively address potential issues in your code.
Output:
Warning: control reaches end of non-void function
Using compiler warnings not only helps you catch errors early but also encourages better coding practices. It’s a simple yet effective way to enhance the quality of your code and avoid common pitfalls that lead to errors.
Conclusion
The “control reaches end of non-void function” error in C++ can be a stumbling block for many developers. However, by ensuring all paths return a value, using default return values, and leveraging compiler warnings, you can effectively resolve this issue. These strategies not only help you fix the error but also improve the overall quality of your code. Remember, writing clear and maintainable code is just as important as making it functional. With these tips in hand, you’ll be better prepared to tackle this error and enhance your C++ programming skills.
FAQ
-
what causes the “control reaches end of non-void function” error?
This error occurs when a non-void function does not have a return statement for all possible execution paths. -
how can I ensure my function always returns a value?
You can ensure your function returns a value by checking all conditional paths and providing a return statement for each scenario. -
what is a default return value?
A default return value is a value returned by a function when none of the specified conditions are met, ensuring that the function always returns a value. -
how can compiler warnings help me?
Compiler warnings can alert you to potential issues in your code, including missing return statements, allowing you to fix errors before they become problematic. -
is it good practice to use default return values?
Yes, using default return values can make your code cleaner and more robust, as it ensures that every execution path results in a return statement.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook