How to Check if Pointer Is NULL in C++
- Understanding Null Pointers in C++
- Method 1: Using if Statement
- Method 2: Using Pointer Arithmetic
- Method 3: Using the Ternary Operator
-
Method 4: Use Comparison With
0
to Check if Pointer IsNULL
in C++ - Conclusion
- FAQ
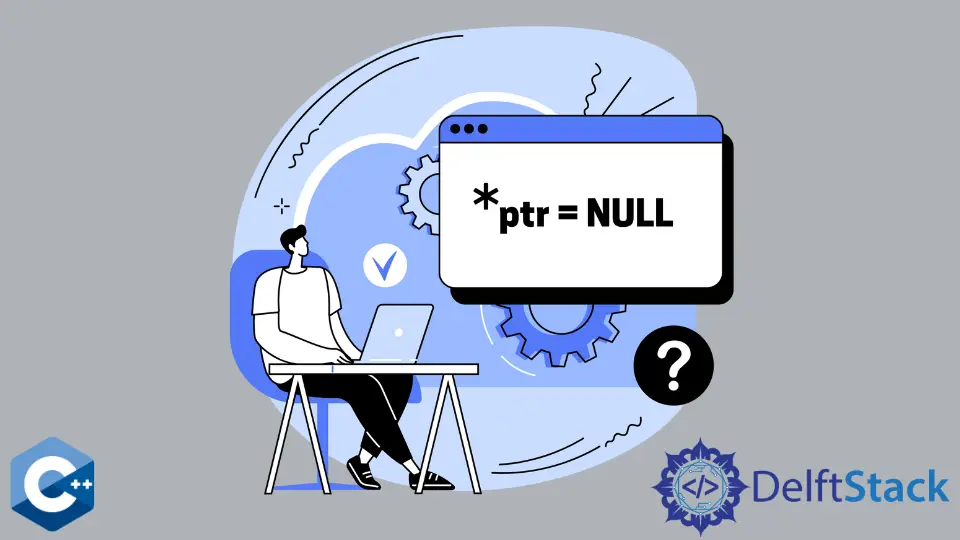
In C++, pointers are a fundamental part of the language, allowing for dynamic memory management and efficient data manipulation. However, working with pointers also comes with its challenges, including the potential for null pointer dereferencing. Knowing how to check if a pointer is null is crucial for writing robust and error-free code.
In this article, we will explore various methods to check if a pointer is null in C++, using clear examples and explanations. Whether you’re a novice programmer or an experienced developer, understanding these techniques will enhance your coding skills and help you avoid common pitfalls.
Understanding Null Pointers in C++
Before diving into the methods of checking for null pointers, it’s essential to understand what a null pointer is. A null pointer is a pointer that does not point to any valid memory location. In C++, null pointers are often represented by the constant nullptr
or the integer value 0
. Dereferencing a null pointer can lead to undefined behavior, crashes, or memory leaks. Therefore, checking if a pointer is null before using it is a best practice that every C++ programmer should adopt.
Method 1: Using if Statement
The most straightforward approach to check if a pointer is null in C++ is by using an if
statement. This method involves a simple comparison between the pointer and nullptr
. Here’s how you can implement it:
#include <iostream>
int main() {
int* ptr = nullptr;
if (ptr == nullptr) {
std::cout << "Pointer is null." << std::endl;
} else {
std::cout << "Pointer is not null." << std::endl;
}
return 0;
}
Output:
Pointer is null.
In this example, we declare a pointer ptr
and initialize it to nullptr
. The if
statement checks whether ptr
is equal to nullptr
. If it is, we print a message indicating that the pointer is null. If the pointer were to point to a valid memory location, the else block would execute, confirming that the pointer is not null. This method is effective and easy to understand, making it a popular choice among C++ developers.
Method 2: Using Pointer Arithmetic
Another interesting way to check if a pointer is null is through pointer arithmetic. While this method is less conventional, it can be useful in specific scenarios. Here’s how you can implement it:
#include <iostream>
int main() {
int* ptr = nullptr;
if (ptr) {
std::cout << "Pointer is not null." << std::endl;
} else {
std::cout << "Pointer is null." << std::endl;
}
return 0;
}
Output:
Pointer is null.
In this code snippet, we leverage the fact that pointers can be evaluated in a boolean context. If ptr
is null, it evaluates to false
, and the else block executes, indicating that the pointer is null. If ptr
were to point to a valid memory address, the if block would execute, confirming that the pointer is not null. This method is concise and takes advantage of C++’s type conversion rules, allowing for a more streamlined check.
Method 3: Using the Ternary Operator
The ternary operator in C++ offers a compact way to perform conditional checks, including checking if a pointer is null. Here’s an example of how to use it for this purpose:
#include <iostream>
int main() {
int* ptr = nullptr;
std::cout << (ptr ? "Pointer is not null." : "Pointer is null.") << std::endl;
return 0;
}
Output:
Pointer is null.
In this example, we use the ternary operator to evaluate ptr
. If ptr
is not null, it prints “Pointer is not null.” Otherwise, it prints “Pointer is null.” This method is particularly useful for quick checks and can help reduce the amount of code you write, making your program cleaner and more readable.
Method 4: Use Comparison With 0
to Check if Pointer Is NULL
in C++
There is also a preprocessor variable named NULL
, which has roots in the C standard library and is often used in legacy code. Mind that it is not recommended to use NULL
in contemporary C++ programming because it’s equivalent to initialization by the integer 0
, and the problems may arise as stated in the previous section. Still, we can check if a pointer is null by comparing it to 0
as demonstrated in the next code sample:
#include <iostream>
using std::cout;
using std::endl;
#define SIZE 123
int main() {
char *arr = (char *)malloc(SIZE);
if (arr != 0) {
cout << "Valid pointer!" << endl;
} else {
cout << "NULL pointer!" << endl;
}
free(arr);
return EXIT_SUCCESS;
}
Output:
Valid pointer!
This code demonstrates the use of null pointer checking in C++, particularly in the context of memory allocation. It allocates memory for a character array using malloc
and then checks if the allocation was successful by comparing the pointer to 0. This method of null checking is compatible with older C-style code but is not the preferred approach in modern C++.
The program allocates memory, checks if the allocation was successful, and then frees the memory. While this code works, it’s worth noting that in modern C++, it’s generally better to use nullptr
instead of 0 for null pointer checks, and to prefer C++ memory management techniques (like new
and delete
, or better yet, smart pointers) over C-style malloc
and free
. This example serves to illustrate how null checks were traditionally done in C and older C++ code.
Conclusion
Checking if a pointer is null in C++ is a critical skill for any programmer. By employing methods such as using if
statements, pointer arithmetic, and the ternary operator, you can effectively avoid null pointer dereferencing and enhance the stability of your applications. Each method has its advantages, and understanding when to use each can improve your overall coding practices. As you continue to develop your skills in C++, always remember the importance of handling pointers carefully to prevent errors and ensure your programs run smoothly.
FAQ
-
what is a null pointer in C++?
A null pointer is a pointer that does not point to any valid memory location, often represented by nullptr. -
how do I initialize a pointer to null?
You can initialize a pointer to null by assigning it nullptr, like this: int* ptr = nullptr; -
what happens if I dereference a null pointer?
Dereferencing a null pointer can lead to undefined behavior, crashes, or memory leaks. -
can I use an integer value to represent a null pointer?
Yes, you can use the integer value 0 to represent a null pointer, but it is recommended to use nullptr for clarity. -
is checking for null pointers necessary in C++?
Yes, checking for null pointers is necessary to avoid runtime errors and ensure your program runs correctly.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook