How to Use Callback Functions in C++
- Declare Callback Functions With Different Notations in C++
-
Use
std::map
to Store Multiple Callback Functions With Corresponding Keys in C++ - Call the Specific Callback Function Based on the User Input in C++
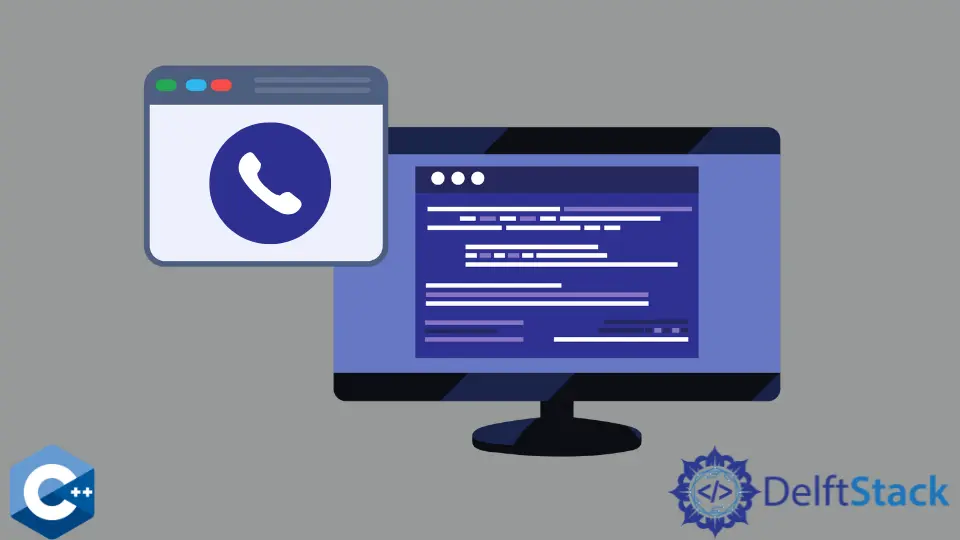
This article will explain several methods of how to use callback functions in C++.
Declare Callback Functions With Different Notations in C++
A callback is a function (i.e., subroutine in the code) passed to other functions as an argument to be called later in program execution.
Callback functions can be implemented using different language-specific tools, but in C++, all of them are known as callable objects. Callable objects can be traditional functions, pointers to the functions, lambda expressions, bind
created objects, classes that overload ()
operator, and std::function
type objects defined in <functional>
header.
Below example code defines two traditional functions addTwoInts
/subtructTwoInts
, one lambda expression stored in the modOfTwoInts1
variable and std::function
type modOfTwoInts2
. These functions implement basic arithmetic operators +
, -
and modulo
for integers.
#include <functional>
#include <iostream>
#include <map>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::function;
using std::map;
using std::string;
int addTwoInts(int i, int j) { return i + j; }
int subtructTwoInts(int i, int j) { return i - j; }
int main() {
auto modOfTwoInts1 = [](int i, int j) { return i % j; };
cout << "modOfTwoInts1(10, 3) = " << modOfTwoInts1(10, 3) << endl;
cout << "addTwoInts(10, 3) = " << addTwoInts(10, 3) << endl;
cout << "subtructTwoInts(10, 3) = " << subtructTwoInts(10, 3) << endl;
function<int(int, int)> modOfTwoInts2 = [](int i, int j) { return i % j; };
cout << "modOfTwoInts2(10, 3) = " << modOfTwoInts2(10, 3) << endl;
return EXIT_SUCCESS;
}
Output:
modOfTwoInts1(10, 3) = 1
addTwoInts(10, 3) = 13
subtructTwoInts(10, 3) = 7
modOfTwoInts2(10, 3) = 1
Use std::map
to Store Multiple Callback Functions With Corresponding Keys in C++
A common way of using callback functions is to store them in the data structure like vector
or map
, from which we can easily access each one of them and call the specific function during program run-time. In this case, we chose a map
container to store arithmetic operators as strings for keys and corresponding functions as values. Note that this code example does not output any content to the console.
#include <functional>
#include <iostream>
#include <map>
using std::cin;
using std::cout;
using std::endl;
using std::function;
using std::map;
using std::string;
int addTwoInts(int i, int j) { return i + j; }
int subtructTwoInts(int i, int j) { return i - j; }
int main() {
auto modOfTwoInts1 = [](int i, int j) { return i % j; };
auto subtruct = subtructTwoInts;
map<string, int (*)(int, int)> op_funcs;
op_funcs.insert({"+", addTwoInts});
op_funcs.insert({"%", modOfTwoInts1});
op_funcs.insert({"-", subtruct});
return EXIT_SUCCESS;
}
Call the Specific Callback Function Based on the User Input in C++
As a consequence of the previous section, stored callback functions in the map
container should be utilized in a practical manner. One way of doing this is to take the operator symbol from the user and pass it to the map
container as a key to call the corresponding function object. This method is often used to handle events in UI applications. Note that we are passing the function called arbitrary integer arguments (10, 3)
.
#include <functional>
#include <iostream>
#include <map>
using std::cin;
using std::cout;
using std::endl;
using std::function;
using std::map;
using std::string;
int addTwoInts(int i, int j) { return i + j; }
int subtructTwoInts(int i, int j) { return i - j; }
int main() {
auto modOfTwoInts1 = [](int i, int j) { return i % j; };
auto subtruct = subtructTwoInts;
map<string, int (*)(int, int)> op_funcs;
op_funcs.insert({"+", addTwoInts});
op_funcs.insert({"%", modOfTwoInts1});
op_funcs.insert({"-", subtruct});
string user_input;
cout << "\nType one of the following ops\n"
"for integers 10 and 3 to be used:\n"
"1) + 2) - 3) % \n";
cin >> user_input;
cout << op_funcs[user_input](10, 3);
return EXIT_SUCCESS;
}
Output (if the input is +
):
Type one of the following ops
for integers 10 and 3 to be used:
1) + 2) - 3) %
+
13
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook