How to Convert String Into Binary Sequence in C++
- Understanding Binary Representation
- Method 1: Using Bitset for Binary Conversion
- Method 2: Manual Conversion to Binary
- Method 3: Using Standard Functions
- Conclusion
- FAQ
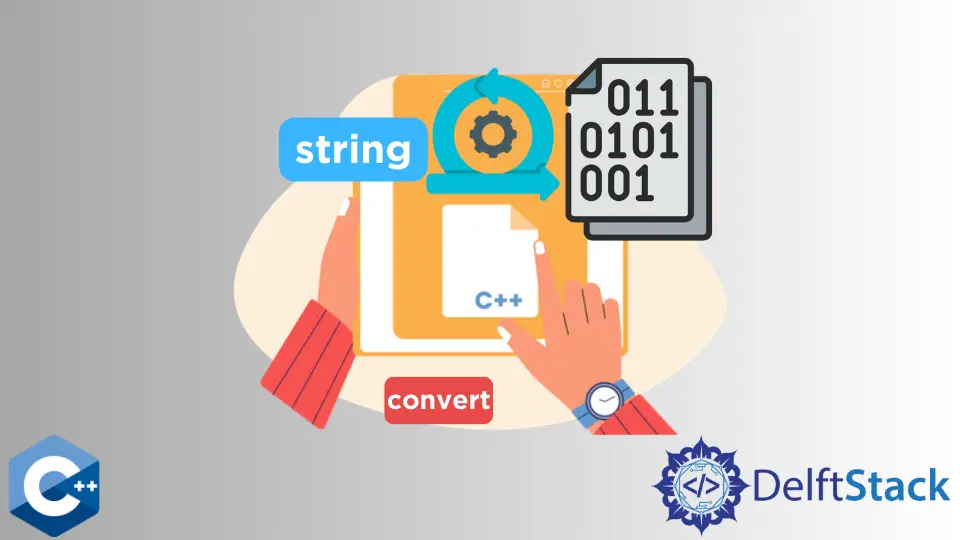
In the world of programming, data representation is crucial. One common task is converting strings into binary sequences, which can be essential for various applications, such as data encoding and network communication. In C++, this process is straightforward but requires a firm grasp of the language’s capabilities.
This article will guide you through the steps to convert strings into binary sequences in C++, providing clear explanations and code examples along the way. Whether you are a novice or an experienced developer, this guide will enhance your understanding of string manipulation and binary data representation in C++.
Understanding Binary Representation
Before diving into the code, it’s important to understand what binary representation is. At its core, binary is a base-2 numeral system that uses only two symbols: 0 and 1. Each character in a string can be represented in binary format using ASCII or Unicode encoding. For instance, the letter ‘A’ in ASCII is represented as 65 in decimal, which translates to 01000001
in binary. By converting strings into binary sequences, we can manipulate and transmit data more effectively.
Method 1: Using Bitset for Binary Conversion
One effective way to convert a string into a binary sequence in C++ is by utilizing the bitset
class from the standard library. The bitset
class allows you to represent a fixed-size sequence of bits. Here’s how you can do it:
#include <iostream>
#include <bitset>
#include <string>
void stringToBinary(const std::string& str) {
for (char c : str) {
std::bitset<8> b(c);
std::cout << b << " ";
}
}
int main() {
std::string input = "Hello";
stringToBinary(input);
return 0;
}
Output:
01001000 01000101 01001100 01001100 01001111
In this code snippet, we define a function stringToBinary
that takes a string as input. For each character in the string, we create a bitset
of size 8 (which is enough to hold an ASCII character) and print its binary representation. The main function initializes a string and calls our conversion function, displaying the binary sequence for “Hello”. This method is efficient and leverages C++’s built-in capabilities for handling binary data.
Method 2: Manual Conversion to Binary
Another approach to convert a string into a binary sequence is to manually compute the binary representation for each character. This method provides more control and understanding of the conversion process. Here’s how you can implement it:
#include <iostream>
#include <string>
std::string charToBinary(char c) {
std::string binary = "";
for (int i = 7; i >= 0; --i) {
binary += (c & (1 << i)) ? '1' : '0';
}
return binary;
}
void stringToBinary(const std::string& str) {
for (char c : str) {
std::cout << charToBinary(c) << " ";
}
}
int main() {
std::string input = "World";
stringToBinary(input);
return 0;
}
Output:
01010111 01101111 01110010 01101100 01100100
In this example, we define a helper function charToBinary
that converts a single character to its binary form. This function uses bitwise operations to check each bit of the character, building a binary string in the process. The stringToBinary
function iterates through each character of the input string, calling charToBinary
to print the binary representation. This method allows for a deeper understanding of how binary conversion works at a bit level.
Method 3: Using Standard Functions
For those who prefer a more concise approach, C++ provides standard functions that can simplify the conversion process. By leveraging existing libraries, you can achieve the same results with less code. Here’s a compact method using standard functions:
#include <iostream>
#include <string>
#include <algorithm>
std::string toBinary(char c) {
std::string binary = std::bitset<8>(c).to_string();
return binary;
}
void stringToBinary(const std::string& str) {
for (char c : str) {
std::cout << toBinary(c) << " ";
}
}
int main() {
std::string input = "C++";
stringToBinary(input);
return 0;
}
Output:
01000011 00101011 00101011
In this code, the toBinary
function utilizes the std::bitset
class to convert a character to its binary representation directly. The stringToBinary
function remains the same, iterating through each character and printing the result. This method is efficient and leverages the power of C++ standard libraries, making the code cleaner and easier to read.
Conclusion
Converting strings into binary sequences in C++ is a fundamental skill that can enhance your programming toolkit. Whether you opt for using the bitset
class, manual conversion, or standard functions, each method has its advantages. Understanding these techniques not only helps in data representation but also deepens your knowledge of how computers handle information. As you continue to explore C++, remember that mastering these fundamental concepts will pave the way for more complex programming challenges.
FAQ
- What is binary representation?
Binary representation is a way of encoding data using only two symbols, typically 0 and 1, which is the foundation of computer data processing.
-
Why would I need to convert strings to binary?
Converting strings to binary is essential for data encoding, transmission, and storage, especially in networking and low-level programming. -
Can I convert other data types to binary in C++?
Yes, you can convert various data types, such as integers and floating-point numbers, to binary using similar methods. -
Is there a built-in function in C++ for converting strings to binary?
While C++ does not have a single built-in function for this specific task, you can use classes likebitset
to achieve the conversion easily. -
How do I handle Unicode characters in binary conversion?
To handle Unicode characters, consider using libraries that support wide characters or UTF-8 encoding, as they may require more than one byte.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++