The const Keyword in C++
-
Declaring
Const
Variables in C++ -
Use the
const
With Pointers in C++ -
Pointer Variable to
const
Variable in C++ -
the
const
Pointer Variable to a Value in C++ -
the
const
Pointer Variable toconst
Variable in C++ -
the
const
Member Function andconst
Object in C++
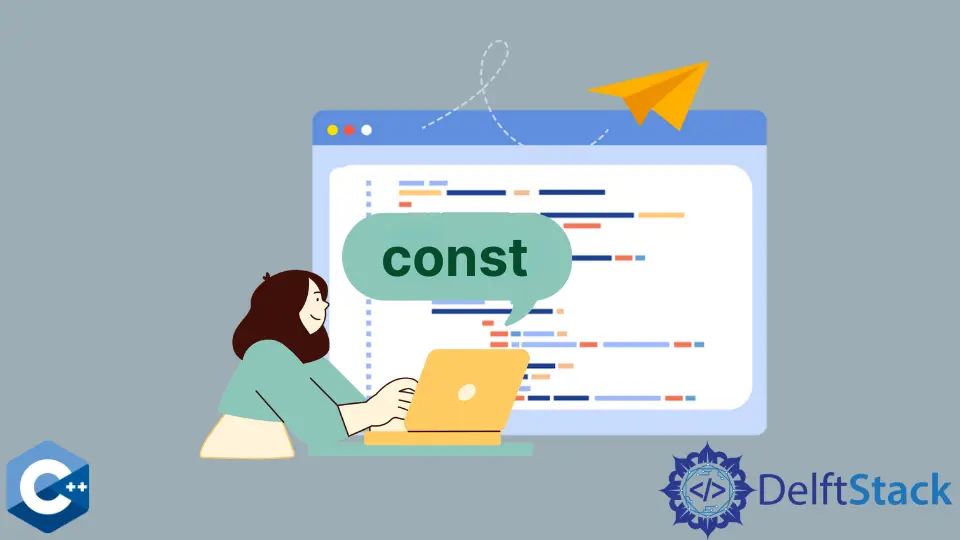
The const
keyword, which stands for Constant in C++, is used to make a specific value/values constant throughout the whole program.
Once a variable/object/function
is declared stable, the compiler will not let the programmer modify the value assigned during the rest of the program.
It ensures that the program runs uninterrupted during its execution. If the programmer tries to change the value later, the compiler will show a compilation error.
The main advantage of the const
is that it allows a specific value/values to be constant throughout the whole program. As mentioned earlier, also it will enable the compiler to make specific optimizations that otherwise are not possible.
The const
keyword can use in specific different ways in a program to address different programming needs.
Declaring Const
Variables in C++
When making a variable constant, the variable must always be initialized while declaring. After the variable is said, it is impossible to change its value in a different part of the code.
To declare a variable as a constant;
const int x = 1;
Use the const
With Pointers in C++
There are three ways to use the const
keyword with pointers.
- Pointer variable to
const
value. - The
const
pointer variable to a value. - The
const
pointer to aconst
variable.
Pointer Variable to const
Variable in C++
It means that the pointer is pointing to a constant
variable, we can use the value of the variable that the pointer is pointing to, but we cannot change the variable’s value using the pointer.
const int* y;
It is beneficial when making a string or an array immutable.
the const
Pointer Variable to a Value in C++
Here the pointer is a constant, but the pointer’s value is not constant; therefore, it is possible to change its value.
Furthermore, though we change the value, we cannot change the memory location of the pointer. It is essential when storage changes its value but not its memory location.
int z = 2;
const int* y = &z;
the const
Pointer Variable to const
Variable in C++
After the values are assigned in this situation, it is impossible to change the pointer variable or the variable’s value that the pointer is pointing to.
int i = 3 const int* const j = &i;
the const
Member Function and const
Object in C++
C++ is an object-oriented programming language, and there are instances in which a created object is not intended for any sought of a change during any part of the program.
Therefore, making an object constant using the const
keyword can be very helpful for situations like this. If an object is needed to be declared as const
, it must be initialized at the moment of declaration.
After the object is initialized, it is impossible to change any of the dimensions given to the object during the rest of the program.
The compiler will throw a compilation error. Here is an example of a const
object.
#include <iostream>
using namespace std;
class length {
public:
int x;
length(int y) { x = y; }
};
int main() {
const length obj1(15);
cout << "The length of object 1 is " << obj1.x << endl;
return 0;
}
Output:
The length of object 1 is 15
When a function is declared followed by const
, a constant function is created. It is mainly used with const
objects.
It is important to note that if a member function is called through a const
object, then the compiler will throw an error regardless of whether the member function intended to change the object or not.
Here is an example of a const
member function.
#include <iostream>
using namespace std;
class length {
public:
int x;
length(int y) { x = y; }
int getvalue() const { return x; }
};
int main() {
const length obj1(15);
cout << "The length of object 1 is " << obj1.getvalue() << endl;
return 0;
}
Output:
The length of object 1 is 15
The point of const
is that it does not allow modifying things that should not modify.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.