How to Use the const Keyword With Pointers in C++
- Understanding the const Keyword
- Constant Pointer to Non-Constant Data
- Pointer to Constant Data
- Constant Pointer to Constant Data
- Conclusion
- FAQ
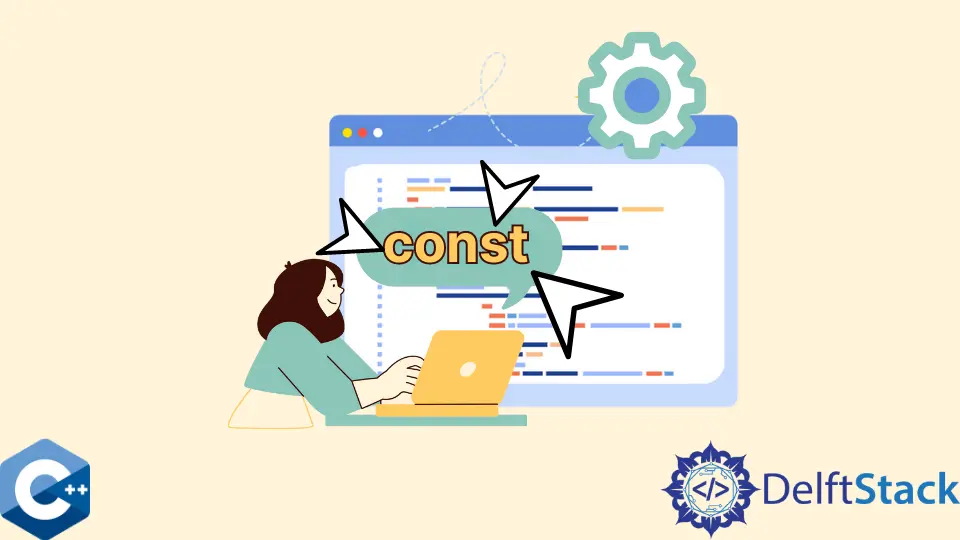
Understanding how to effectively use the const
keyword with pointers in C++ can greatly enhance your coding skills and lead to more robust applications. In C++, pointers are a powerful feature that allows you to manipulate memory directly, but they can also introduce complexity and potential errors. By using const
, you can enforce immutability, ensuring that certain data cannot be modified unintentionally.
This article will explore the common properties of using the const
keyword with pointers, providing examples and explanations to help you grasp these concepts. Whether you are a beginner or an experienced developer, mastering the use of const
with pointers will improve your C++ programming practices.
Understanding the const Keyword
The const
keyword in C++ serves as a modifier that indicates that a variable’s value cannot be changed after its initial assignment. When applied to pointers, it can be used in several ways, each serving a different purpose.
-
Constant Pointer to Non-Constant Data
: This means that the pointer itself cannot change the address it points to, but the data at that address can be modified. -
Pointer to Constant Data
: In this case, the pointer can point to different addresses, but the data at those addresses cannot be modified. -
Constant Pointer to Constant Data
: Here, neither the pointer can change its address nor can the data at that address be modified.
Understanding these distinctions is crucial for writing safe and efficient C++ code.
Constant Pointer to Non-Constant Data
To declare a constant pointer to non-constant data, you can use the following syntax:
int value = 10;
int* const ptr = &value;
*ptr = 20; // This is allowed
ptr = nullptr; // This will cause a compilation error
In this example, ptr
is a constant pointer to an integer variable value
. You can modify the value of value
through ptr
, but you cannot change ptr
to point to a different address. This is useful when you want to ensure that the pointer always points to the same memory location.
Output:
value = 20
This code showcases how ptr
can modify the data it points to, while still being a constant pointer. It provides a layer of safety, ensuring that the pointer itself cannot be redirected elsewhere, which is particularly beneficial in scenarios where the integrity of the pointer is crucial.
Pointer to Constant Data
Now, let’s explore how to create a pointer to constant data. This is done as follows:
const int value = 10;
const int* ptr = &value;
// *ptr = 20; // This will cause a compilation error
ptr = nullptr; // This is allowed
In this snippet, ptr
is a pointer to a constant integer. You can change where ptr
points, but you cannot modify the value of value
through ptr
. This is particularly useful when you want to prevent modification of data that should remain constant throughout its lifecycle.
Output:
value = 10
Using a pointer to constant data helps maintain data integrity, especially when passing parameters to functions. It ensures that the function cannot alter the original data, which can prevent unintended side effects and make your code easier to understand and maintain.
Constant Pointer to Constant Data
Finally, let’s look at a constant pointer to constant data. The syntax is as follows:
const int value = 10;
const int* const ptr = &value;
// *ptr = 20; // This will cause a compilation error
// ptr = nullptr; // This will also cause a compilation error
In this example, ptr
is both a constant pointer and points to constant data. This means you cannot change the address ptr
points to, nor can you change the value of value
. This is the strictest level of immutability, ensuring that both the pointer and the data it points to remain unchanged.
Output:
value = 10
Using a constant pointer to constant data is particularly useful in scenarios where you want to ensure that both the data and the reference to that data remain unchanged throughout your program. This can lead to safer and more predictable code, especially in larger projects where data integrity is paramount.
Conclusion
In conclusion, mastering the use of the const
keyword with pointers in C++ is essential for writing safe and efficient code. By understanding the different types of constant pointers and their applications, you can prevent unintended modifications and improve the maintainability of your programs. Whether you use constant pointers to enforce data integrity or to clarify your code’s intentions, the const
keyword is a powerful tool in your C++ programming arsenal. Embrace these concepts, and you’ll find yourself writing more robust and error-free code.
FAQ
-
What does the
const
keyword do in C++?
Theconst
keyword indicates that a variable’s value cannot be changed after its initial assignment. -
Can I modify the data pointed to by a constant pointer?
Yes, you can modify the data if the pointer itself is not constant. -
What is the difference between a pointer to constant data and a constant pointer?
A pointer to constant data cannot modify the data it points to, while a constant pointer cannot change the address it points to. -
When should I use constant pointers in my code?
Use constant pointers when you want to ensure that the pointer itself does not change, which can help prevent errors in your program. -
How does using
const
improve code safety?
Usingconst
helps prevent unintended modifications to data, making your code more predictable and easier to maintain.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook