Const at the End of Function in C++
- Understanding the Const Keyword
- Benefits of Using Const at the End of Functions
- Example of a Const Member Function
- Overloading Functions with Const
- Conclusion
- FAQ
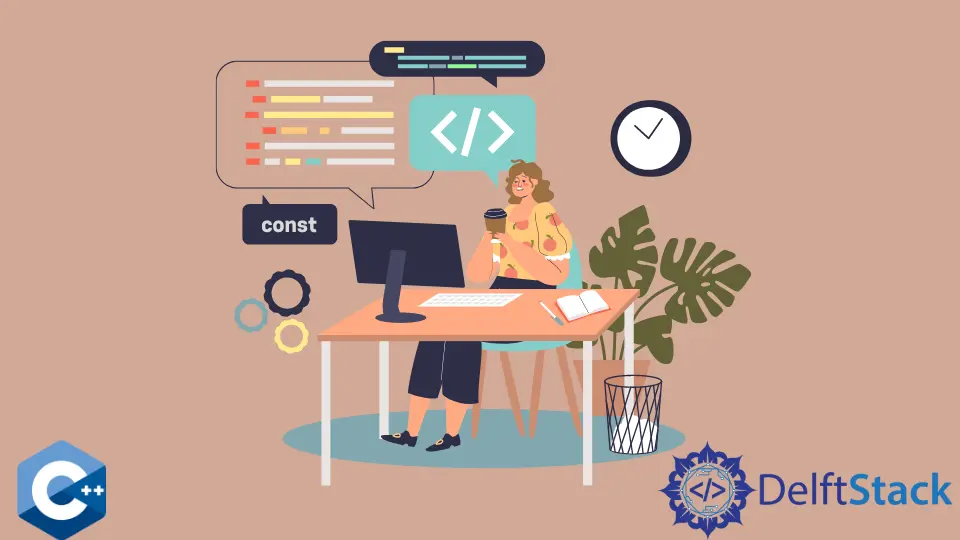
In C++, the const
keyword plays a vital role in ensuring data integrity and promoting safer programming practices. One intriguing aspect of its usage is placing const
at the end of a member function.
This tutorial delves into this concept, explaining how it enhances code reliability and clarity. By the end of this article, you will understand the significance of using const
at the end of functions and how it impacts object-oriented programming in C++. Whether you are a beginner or an experienced developer, this information will bolster your understanding of C++ and improve your coding skills.
Understanding the Const Keyword
The const
keyword in C++ is primarily used to define constants, which means that the value cannot be changed after its initialization. When used at the end of a member function, it indicates that the function will not modify any member variables of the class. This is crucial in maintaining the integrity of objects, especially when working with complex data structures.
For instance, consider a class representing a geometric shape. If you have a method that calculates the area of the shape, marking it as const
ensures that this method does not alter any of the shape’s properties. This not only makes your code safer but also clearer to anyone reading it, as they can immediately see which functions are intended to be read-only.
Benefits of Using Const at the End of Functions
Using const
at the end of member functions provides several advantages:
- Code Safety: It prevents accidental modifications of class members, reducing bugs.
- Clarity: It makes the intent of the function clear to other developers.
- Overloading: It allows function overloading based on constness, providing flexibility in design.
- Optimization: Some compilers can optimize code better when they know certain functions do not modify the state of an object.
By incorporating const
in your member functions, you not only adhere to best programming practices but also create more maintainable and understandable code.
Example of a Const Member Function
Let’s consider a simple example of a class that represents a Circle
. We will implement a member function to calculate the area of the circle, marking it as const
.
#include <iostream>
#define PI 3.14159
class Circle {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() const {
return PI * radius * radius;
}
};
int main() {
Circle circle(5.0);
std::cout << "Area of circle: " << circle.area() << std::endl;
return 0;
}
Output:
Area of circle: 78.5398
In this example, the area()
function is marked as const
, indicating that it does not modify any member variables of the Circle
class. The constructor initializes the radius, and the area()
function calculates the area based on that radius without altering it. This ensures that the state of the Circle
object remains unchanged, adhering to the principles of const-correctness.
Overloading Functions with Const
Another interesting aspect of using const
in C++ is the ability to overload functions based on their constness. This means you can have two versions of the same function: one that modifies the object and one that does not. Let’s take a look at an example involving a class that manages a simple array.
#include <iostream>
class ArrayManager {
private:
int* arr;
int size;
public:
ArrayManager(int s) : size(s) {
arr = new int[size];
for (int i = 0; i < size; i++) {
arr[i] = i + 1;
}
}
int& operator[](int index) {
return arr[index];
}
const int& operator[](int index) const {
return arr[index];
}
~ArrayManager() {
delete[] arr;
}
};
int main() {
ArrayManager array(5);
array[0] = 10;
std::cout << "First element: " << array[0] << std::endl;
const ArrayManager constArray(5);
std::cout << "First element from const object: " << constArray[0] << std::endl;
return 0;
}
Output:
First element: 10
First element from const object: 1
In this example, we define two overloaded operator[]
functions: one that returns a non-const reference and another that returns a const reference. This allows us to modify the array elements through a non-const object while ensuring that const objects can only read the values without modifying them. This feature enhances the flexibility and safety of your code.
Conclusion
In conclusion, using const
at the end of functions in C++ is a powerful feature that enhances the safety, clarity, and maintainability of your code. By marking functions as const
, you signal to both the compiler and other developers that these functions do not modify the state of the object, which can prevent bugs and make your code easier to understand. Whether you are working on simple classes or complex data structures, incorporating const
into your member functions is a best practice that pays off in the long run. Embrace this feature in your C++ programming journey for more robust and reliable applications.
FAQ
-
What does const at the end of a function mean in C++?
It indicates that the function will not modify any member variables of the class. -
Can I overload functions based on constness in C++?
Yes, you can have different versions of the same function for const and non-const objects. -
Why is const-correctness important in C++?
It helps prevent accidental modifications to objects, making the code safer and easier to maintain. -
How does const improve code readability?
It clearly indicates which functions are intended to be read-only, making the intent of the code more apparent. -
Are there performance benefits to using const in C++?
Yes, some compilers can optimize code better when they know certain functions do not alter the state of an object.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook