How to Check if Input Is Integer in C++
-
Use the
std::find_if
Algorithm to Check if Input Is Integer in C++ -
Use the
std::string::find_first_not_of
Function to Check if Input Is Integer in C++ -
Use the
std::string::find_first_not_of
Function to Check if Input Is Integer in C++
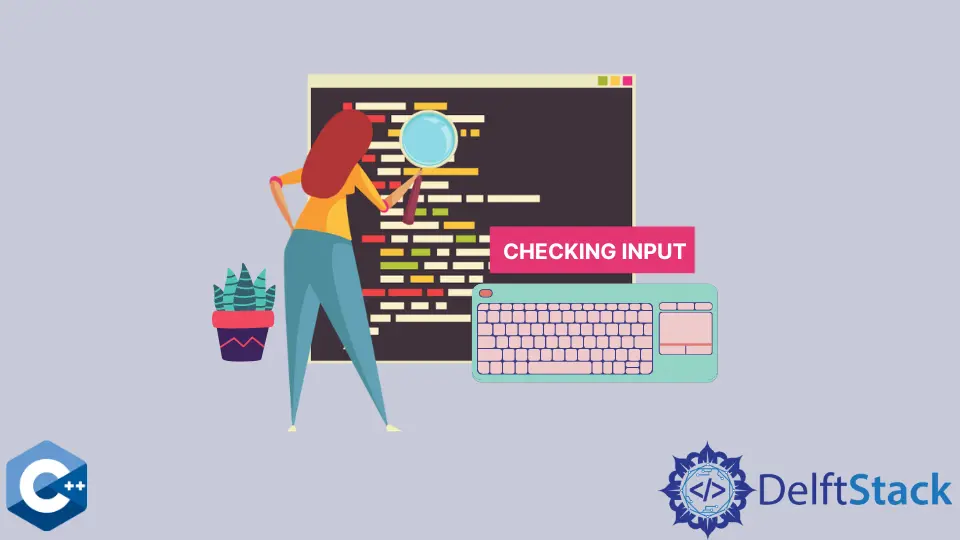
This article will demonstrate multiple methods about how to check if the input is an integer in C++.
Use the std::find_if
Algorithm to Check if Input Is Integer in C++
std::find_if
is part of the STL algorithms library defined in the <alogrithm>
header file, and it can be utilized to search for the specific element in the range. Since the user input is most likely to be a string, we will assume that the input data is stored in a std::string
object. Note that we implement a function called isNumber
that takes a reference to std::string
and returns the bool
value.
The prototype of the std::find_if
function that we used in the following example takes three arguments, the first two of which specify the range elements - [first, last]
. The third argument is a unary predicate, which is a lambda function that returns the bool
value from evaluating the inverted value of the isdigit
function. On the outer layer, the std::find_if
return value is compared to the str.end()
, as the true
value of the expression indicates that no non-digit character was found; thus it’s the number. Additionally, we logically AND the former expression with the !str.empty
to indicate that string is empty and return false
.
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool isNumber(const string& str) {
return !str.empty() &&
std::find_if(str.begin(), str.end(), [](unsigned char c) {
return !std::isdigit(c);
}) == str.end();
}
int main() {
string str1 = "12345.";
string str2 = "12312";
string str3 = "123142.2";
isNumber(str1) ? cout << "Number\n" : cout << "Not number\n";
isNumber(str2) ? cout << "Number\n" : cout << "Not number\n";
isNumber(str3) ? cout << "Number\n" : cout << "Not number\n";
exit(EXIT_SUCCESS);
}
Output:
Not number
Number
Not number
Use the std::string::find_first_not_of
Function to Check if Input Is Integer in C++
Alternatively, we can reimplement isNumber
function using the find_first_not_of
method that is built-in for the std::string
object. find_first_not_of
can take the string value and find the first character that equals none of the characters in the string sequence. If the function does not find such a character, string::npos
is returned. Thus, we specify all ten decimal digits as find_first_not_of
argument and check its equality to npos
, as the expression value is returned from the function.
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool isNumber(const string& str) {
return str.find_first_not_of("0123456789") == string::npos;
}
int main() {
string str1 = "12345.";
string str2 = "12312";
string str3 = "123142.2";
isNumber(str1) ? cout << "Number\n" : cout << "Not number\n";
isNumber(str2) ? cout << "Number\n" : cout << "Not number\n";
isNumber(str3) ? cout << "Number\n" : cout << "Not number\n";
exit(EXIT_SUCCESS);
}
Output:
Not number
Number
Not number
Use the std::string::find_first_not_of
Function to Check if Input Is Integer in C++
Note though, the previous method does not identify the real numbers and treats them as illegitimate. So, we can add the .
character to the string and allow the function to recognize any sequence of digits with a dot symbol as a valid number. We need to eliminate two cases when the .
character is the first and the last symbol in the input sequence, which by our convention would not be a valid real number. We can use string
built-in methods front
and back
to verify that input does not begin/end with a dot symbol. Finally, we logically AND all three expressions with each other and return that value.
#include <algorithm>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
bool isNumber3(const string& str) {
return str.find_first_not_of(".0123456789") == string::npos &&
str.front() != '.' && str.back() != '.';
}
int main() {
string str1 = "12345.";
string str2 = "12312";
string str3 = "123142.2";
isNumber(str1) ? cout << "Number\n" : cout << "Not number\n";
isNumber(str2) ? cout << "Number\n" : cout << "Not number\n";
isNumber(str3) ? cout << "Number\n" : cout << "Not number\n";
exit(EXIT_SUCCESS);
}
Output:
Not number
Number
Number
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook