How to Convert Char to String in C++
-
Use the
+/+=
Operator to Convertchar
tostring
in C++ -
Use
string::string(size_type count, charT ch)
Constructor to Convertchar
tostring
in C++ -
Use the
push_back()
Method to Convertchar
tostring
in C++ -
Use the
append()
Method to Convertchar
tostring
in C++ -
Use the
assign()
Method to Convertchar
tostring
in C++ -
Use the
insert()
Method to Convertchar
tostring
in C++ -
Use the
replace()
Method to Convertchar
tostring
in C++ -
Use
std::stringstream
to Convertchar
tostring
in C++ - Conclusion
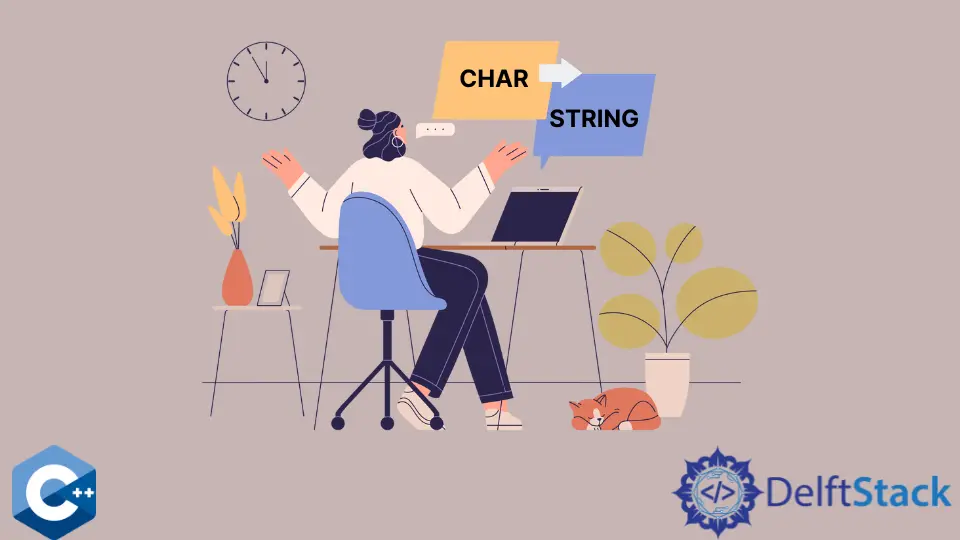
In C++ programming, converting a character (char
) to a string is a common operation that often arises when dealing with text manipulation and formatting. C++ provides several ways to convert a char
to a string, each with advantages and use cases.
This comprehensive guide will delve into all available methods for converting a given char
to a string
in C++, accompanied by examples and comparisons, helping you make an informed decision about the best approach for your coding needs.
Use the +/+=
Operator to Convert char
to string
in C++
The most straightforward method uses the basic +
or +=
operator. These operators are overloaded to assign or append a character to a string.
#include <bits/stdc++.h>
using namespace std;
int main() {
char x = 'D';
string a;
// Using += operator (here we append a char to the end of the string)
a += x;
cout << a << endl;
// using = operator (overwrites the string)
a = "DelftStack";
a = x;
cout << a << endl;
}
Output:
Use string::string(size_type count, charT ch)
Constructor to Convert char
to string
in C++
This method uses one of the std::string
constructors to convert a character to a string object in C++. The constructor takes 2 arguments: a count
value, the number of characters a new string will consist of, and a char
value assigned to each character.
Note that this method defines the CHAR_LENGTH
variable for better readability. We can pass the integer literal directly to the constructor.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
constexpr int CHAR_LENGTH = 1;
int main() {
char character = 'D';
string tmp_string(CHAR_LENGTH, character);
cout << tmp_string << endl;
return EXIT_SUCCESS;
}
Output:
Use the push_back()
Method to Convert char
to string
in C++
Alternatively, we can utilize the push_back
built-in method to convert a character into a string variable. At first, we declare an empty string variable and then use the push_back()
method to append a char
.
Based on the example, we declare the char
variable named character
, later passed as an argument to the push_back
command. Still, you can directly specify the literal value as a parameter.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
char character = 'D';
string tmp_string;
tmp_string.push_back(character);
cout << tmp_string << endl;
return EXIT_SUCCESS;
}
Output:
Use the append()
Method to Convert char
to string
in C++
The append
method is a member function of the std::string
class and can be used to append additional characters to the string object. In this case, we would only need to declare an empty string and add a char
to it, as demonstrated in the following example code.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
char character = 'D';
string tmp_string;
tmp_string.append(1, character);
cout << tmp_string << endl;
return EXIT_SUCCESS;
}
Output:
Use the assign()
Method to Convert char
to string
in C++
The assign()
method is also a member of the std::string
class and functions similar to the =
operator. However, it one-ups the =
operator by giving the advantage of the ability to append as many characters as we would want in the code.
#include <bits/stdc++.h>
using namespace std;
int main() {
string x = "DelftStack";
// assign method overwrites initial content
x.assign(1, 'D');
cout << x << endl;
}
Output:
Use the insert()
Method to Convert char
to string
in C++
The insert
method is also part of the std::string
class. This member function can insert a given char
to a specific position in a string object specified by the first argument.
The second argument denotes the number of copies of the characters to be inserted in the place.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
char character = 'D';
string tmp_string;
tmp_string.insert(0, 1, character);
cout << tmp_string << endl;
return EXIT_SUCCESS;
}
Output:
Use the replace()
Method to Convert char
to string
in C++
Another method belonging to the std::string
class makes this list and can be used to convert a char
to a string
. The replace()
function operates by replacing the portion of the string (len
characters starting from a specified pos
) by a specified number n
copies of the given character.
#include <iostream>
#include <string>
int main() {
char x = 'D';
// using `std::string::replace`
std::string a;
a.replace(0, 1, 1, x);
std::cout << a << std::endl;
return 0;
}
Output:
Use std::stringstream
to Convert char
to string
in C++
The std::stringstream
class from the <sstream>
header offers a flexible way to convert a char
to a string, particularly when more complex string building is required.
This is one method that is utilized rarely and makes sense only if your code needs multiple conversions like string
to int
, string
to floats
, etc.
This method can be used as explained in the following code.
#include <bits/stdc++.h>
using namespace std;
int main() {
stringstream stream;
// adding the specified character to stream
char x = 'D';
stream << x;
// retrieving back the input into a string
string a;
stream >> a;
cout << a << endl;
}
Output:
Conclusion
Converting a single char
to a string in C++ can be achieved through multiple methods, each catering to different scenarios. Utilizing the std::string
constructor provides a straightforward approach, while string concatenation proves useful for quick conversions.
Using methods from the std::string
class is convenient when dealing with numerical characters. For more versatile string building, std::stringstream
offers a powerful solution.
Consider simplicity, readability, and performance when choosing the best method for your char-to-string
conversion needs. By understanding the strengths of each approach, you can ensure efficient and effective coding practices in your C++ projects.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - C++ String
- How to Capitalize First Letter of a String in C++
- How to Find the Longest Common Substring in C++
- How to Find the First Repeating Character in a String in C++
- How to Compare String and Character in C++
- How to Get the Last Character From a String in C++
- How to Remove Last Character From a String in C++