How to Call Function Within a Function in C++
- Argument Evaluation and Function Chaining in C++
-
Use the
return
Statement to Call a Function Within a Function in C++ -
Use
std::pair
to Return Two Values From the Function in C++ - Use Function Pointers to Call a Function Within a Function in C++
- Conclusion
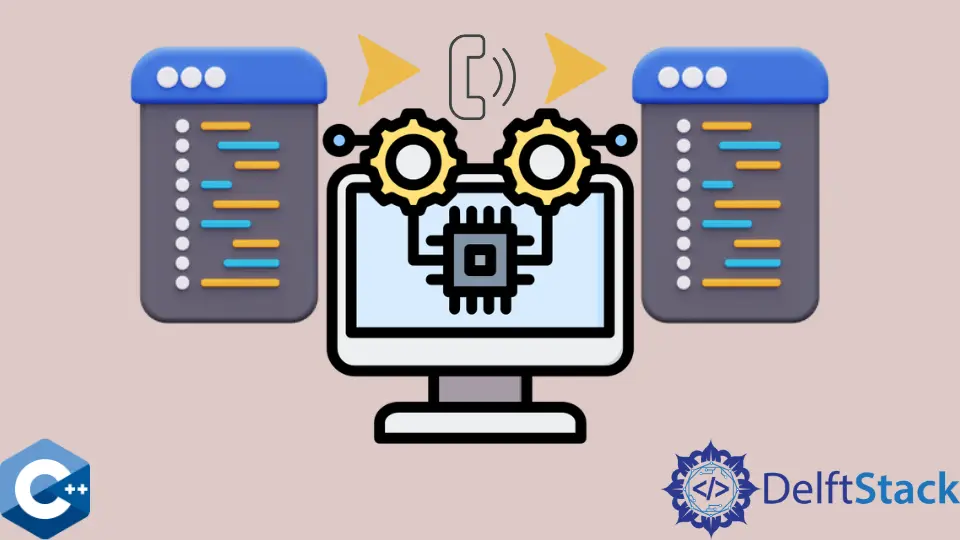
C++ is a powerful and versatile programming language that allows you to create complex and organized code structures. One of the fundamental concepts in C++ is the ability to call functions from within other functions.
This feature allows you to break down complex tasks into smaller, more manageable pieces, improving code readability and maintainability. In this article, we’ll explore several methods of how to call a function within a function in C++.
Argument Evaluation and Function Chaining in C++
One of the captivating features of functions in C++ is their ability to be chained together. This means you can call one function within another function, and the result of the inner function can be used as a parameter for the outer function.
This technique opens up opportunities for building complex and organized code.
Calling one function from within another involves several key steps:
-
Argument Evaluation
The initial step is to compute the values for the function’s arguments. These arguments are placed in the local scope of the function, making them accessible within its body.
-
Stack Frame Allocation
Given that most functions involve local variables, a separate memory space known as a stack frame is created. This space stores local variables, including function arguments.
-
Argument Transmission
Function arguments can be passed to the called function in two ways: by copying their values or by referring to them using corresponding names. In cases requiring type conversion, the arguments are adjusted to ensure the called function operates on valid data.
-
Execution Flow
Once argument evaluation is complete, the statements within the function’s block are executed sequentially. Execution continues until a
return
statement is encountered. -
Return and Control Flow
The
return
statement plays a vital role as it not only specifies the value that the function should return but also triggers the return of control flow to the calling function. -
Memory Cleanup
At this point, the automatically allocated stack frame is no longer needed and is discarded, enabling the program to reclaim memory resources. Control flow resumes from where it left off in the calling function.
Moreover, functions in C++ come with a return type, defining the type of object that the function will pass back to the calling code. You have the flexibility to define functions that don’t return any value, denoted by the void
type.
Alternatively, if your function serves a purpose, it can return data of a specific type, allowing you to convey meaningful results to the calling code.
To illustrate the concept of calling functions within functions, consider the following example code:
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int addTwoInts(int i, int j) { return i + j; }
int main() {
cout << "2 + 1 + -3 = " << addTwoInts(addTwoInts(2, 1), -3) << endl;
cout << "(10 + 10) + (11 + -11) = "
<< addTwoInts(addTwoInts(10, 10), addTwoInts(11, -11)) << endl;
cout << "(12 + -9) + 10) + -11) = "
<< addTwoInts(addTwoInts(addTwoInts(12, -9), 10), -11) << endl;
return EXIT_SUCCESS;
}
Output:
2 + 1 + -3 = 0
(10 + 10) + (11 + -11) = 20
(12 + -9) + 10) + -11) = 2
In this code, we can observe how multiple function calls are connected.
Now, let’s take a look at the addTwoInts
function. This function simply adds these two integers together and returns the result.
Moving on to the main
function, this is where the action happens. We have a series of calls to the addTwoInts
function nested within other function calls.
The results of these operations are printed to the console using the cout
statement. In the first cout
statement, we add 2
and 1
together, and then we add -3
to the result. The output will display 2 + 1 + -3 = -1
.
In the second cout
statement, we add 10
and 10
, and then we add 11
and -11
. This leads to the output 10 + 10 + 11 + -11 = 20
.
The third cout
statement follows a similar pattern, with even more nested function calls. This results in the output 12 + -9 + 10 + -11 = 2
.
Use the return
Statement to Call a Function Within a Function in C++
Another effective approach involves using the return
statement to invoke one function within another. However, there are two critical points to remember for this to work smoothly.
First, the called function must return a value; otherwise, your code won’t compile. Second, the calling function should have a return type that matches the returned value from the invoked function.
This is especially crucial when the return
statement is followed by the caller function, as exemplified in the code snippet below.
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int addTwoInts(int i, int j) {
return i + j;
}
int multiplyAccumulate(int i, int j) {
return addTwoInts(i, i * j);
}
int main() {
cout << "multiplyAccumulate(2, 2) = " << multiplyAccumulate(2, 2) << endl;
return EXIT_SUCCESS;
}
Output:
multiplyAccumulate(2, 2) = 6
In this code snippet, we have two functions, addTwoInts
and multiplyAccumulate
, which showcase the concept of invoking one function within another.
The addTwoInts
function takes two integers and returns their sum. The multiplyAccumulate
function takes two integers, multiplies the second one by the first, and then passes the result to the addTwoInts
function.
Within the main
function, we invoke the multiplyAccumulate
function, passing it the values 2
and 2
. This results in a call to addTwoInts(2, 4)
, which computes the sum of 2
and 4
.
The final output is the result of this computation, which is displayed as multiplyAccumulate(1,2) = 6
using the cout
statement.
Use std::pair
to Return Two Values From the Function in C++
While functions typically return a single value, there are scenarios in which you may need to return multiple values to the caller. In such cases, a convenient approach is to employ the std::pair
structure or other data structures like arrays or containers provided by the Standard Template Library (STL) to store and pass these multiple values.
The std::pair
structure is a versatile tool in C++ that enables you to package two values together as a single unit. It is a part of the STL and is particularly useful when you need to return a pair of values from a function.
However, if your requirements involve returning more than two values, you can resort to using arrays or STL containers that offer the necessary functionality for your specific problem.
Let’s delve into the code snippet provided to illustrate this concept:
#include <iostream>
#include <vector>
#include <algorithm>
#include <utility>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
std::pair<int, int> findMaxMin(vector<int> &arr) {
std::pair<int, int> ret;
auto max = std::max_element(arr.begin(), arr.end());
auto min = std::min_element(arr.begin(), arr.end());
ret.first = *max; // Use '*' to dereference the iterator
ret.second = *min; // Use '*' to dereference the iterator
return ret;
}
int main() {
vector<int> array = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
auto ret = findMaxMin(array);
cout << "Maximum element is " << ret.first << ", Minimum is " << ret.second << endl;
return 0;
}
Output:
Maximum element is 10, Minimum is 1
In this code example, we demonstrate how to use the std::pair
structure to return two values, which, in this case, represent the maximum and minimum elements of a given integer vector.
The findMaxMin
function accepts a reference to a vector of integers, arr
, as its input. It then proceeds to find the maximum and minimum elements within this vector using the std::max_element
and std::min_element
functions provided by the STL.
To store and return these two values, the function creates a std::pair<int, int>
named ret
, where the first value (.first
) is set to the maximum element, and the second value (.second
) is set to the minimum element.
The std::distance
function is used to find the index of the maximum and minimum elements within the vector, and these indices are used to retrieve the actual values from the vector.
In the main
function, we create a sample integer vector, array
, and call the findMaxMin
function to find the maximum and minimum elements. Finally, we print out these results.
It’s important to note that if your requirements involve returning more than two values, you can explore other data structures, such as arrays or STL containers, to meet your needs effectively.
Use Function Pointers to Call a Function Within a Function in C++
Another approach to calling a function within another function is by using function pointers. Function pointers are variables that store the memory addresses of functions, allowing you to invoke functions dynamically at runtime.
Function pointers can be a bit daunting for newcomers, but they offer immense flexibility. In order to use function pointers effectively, it’s essential to understand the following concepts:
-
Function Signature: A function signature includes the return type and parameter types of a function. Function pointers must match the signature of the functions they point to.
-
Declaring Function Pointers: Function pointers are declared by specifying the return type and parameter types they point to. For example, to declare a function pointer to a function that takes an integer and returns a float, you would use
float (*funcPtr)(int)
. -
Assigning Function Addresses: Function pointers are assigned the memory addresses of functions. You can initialize a function pointer by assigning it to a function name, like this:
funcPtr = &myFunction;
. -
Invoking Functions: To call a function through a function pointer, you use the
(*funcPtr)
syntax. For example, to callmyFunction
throughfuncPtr
, you would writeresult = (*funcPtr)(arg1);
.
Now, let’s walk through an example of calling functions within functions using function pointers.
Suppose we have two functions, add
and multiply
, and we want to create a higher-order function called calculate
that takes two integers and a function pointer. The calculate
function should dynamically call either add
or multiply
based on the function pointer provided.
Here’s the code:
#include <iostream>
int add(int a, int b) {
return a + b;
}
int multiply(int a, int b) {
return a * b;
}
int calculate(int x, int y, int (*operation)(int, int)) {
return (*operation)(x, y);
}
int main() {
int a = 5, b = 3;
int (*addPtr)(int, int) = &add;
int sum = calculate(a, b, addPtr);
std::cout << a << " + " << b << " = " << sum << std::endl;
int (*multiplyPtr)(int, int) = &multiply;
int product = calculate(a, b, multiplyPtr);
std::cout << a << " * " << b << " = " << product << std::endl;
return 0;
}
In this example, we have two functions, add
and multiply
, that perform addition and multiplication, respectively. The calculate
function takes two integers and a function pointer as arguments, allowing us to perform either addition or multiplication.
We demonstrate this by using function pointers, addPtr
and multiplyPtr
, to call the calculate
function.
When you run this program, it will output:
5 + 3 = 8
5 * 3 = 15
As shown in this example, function pointers provide a powerful mechanism for creating higher-order functions that can dynamically call other functions based on the provided function pointers.
Conclusion
In C++, the ability to call functions within functions and chain their results is a powerful tool for creating efficient, organized, and modular code. We’ve covered various methods to achieve this, from understanding argument evaluation and the return
statement to returning multiple values using std::pair
and dynamically invoking functions using function pointers.
These techniques open up a world of possibilities for us to design complex and flexible programs in C++.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook