How to Create Boolean Functions in C++
- Implement String Size Comparison as Boolean Function
- Implement Boolean Function that Returns If the Element With Specific Key Exists in a Map
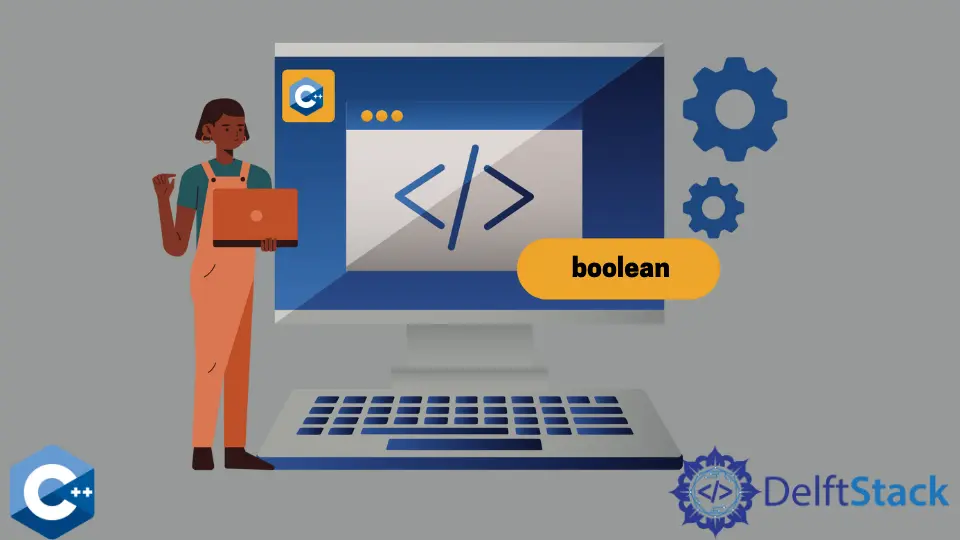
This article will introduce how to create boolean functions in C++.
Implement String Size Comparison as Boolean Function
Boolean function denotes the function that returns a value of type bool
. The structure of the boolean function can be the same as any other function. In the below example, we implement a function isLessString
that compares two strings’ sizes. The function returns true
if the first string’s length is less than the second string; otherwise, it returns false
.
Note that we put the comparison expression after the return
keyword to pass the result value back to the caller function.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
bool isLessString(string &s1, string &s2) { return s1.size() < s2.size(); }
int main() {
string str1 = "This string shall be arbitrary";
string str2 = "Let this string be compared compared";
if (isLessString(str1, str2)) cout << "str1 is shorter than str2";
cout << endl;
return EXIT_SUCCESS;
}
Output:
str1 is shorter than str2
Implement Boolean Function that Returns If the Element With Specific Key Exists in a Map
This example implements the boolean function to find if the element with a specific key exists in a std::map
container. Since the main topic is about function return type bool
, we will utilize the built-in method find
in std::map
instead of implementing the search routine on our own.
find
method takes one argument - key
and returns the iterator to the corresponding element. If no element is found with the specified key, the end
(past-the-end) iterator is returned.
Our keyExistsInMap
function takes map
and string
arguments and calls find
method from the given map
. If the return value of the call is not equal to end
iterator true
is passed back to the caller function; otherwise, it returns false
.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <map>
using std::cout;
using std::endl;
using std::map;
using std::string;
bool keyExistsInMap(map<string, string>& m, const string& key) {
if (m.find(key) != m.end()) {
return true;
} else {
return false;
}
}
int main() {
map<string, string> veggy_map = {{
"a",
"Asparagus",
},
{
"b",
"Beetroot",
},
{
"b",
"Bedsetroot",
},
{
"g",
"Ginger",
},
{
"m",
"Melon",
},
{
"p",
"Pumpkin",
},
{
"s",
"Spinach",
}};
keyExistsInMap(veggy_map, "a") ? cout << "Key exists" << endl
: cout << "Key does not exist\n"
<< endl;
keyExistsInMap(veggy_map, "z") ? cout << "Key exists" << endl
: cout << "Key does not exist\n"
<< endl;
return EXIT_SUCCESS;
}
Output:
Key exists
Key does not exist
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook