How to Append Vector to Vector in C++
-
Append a Vector to Another Vector in C++ Using the
insert
Function -
Append a Vector to Another Vector in C++ Using the
std::copy
Algorithm - Append a Vector to Another Vector in C++ Using a Range-Based Loop
- Conclusion
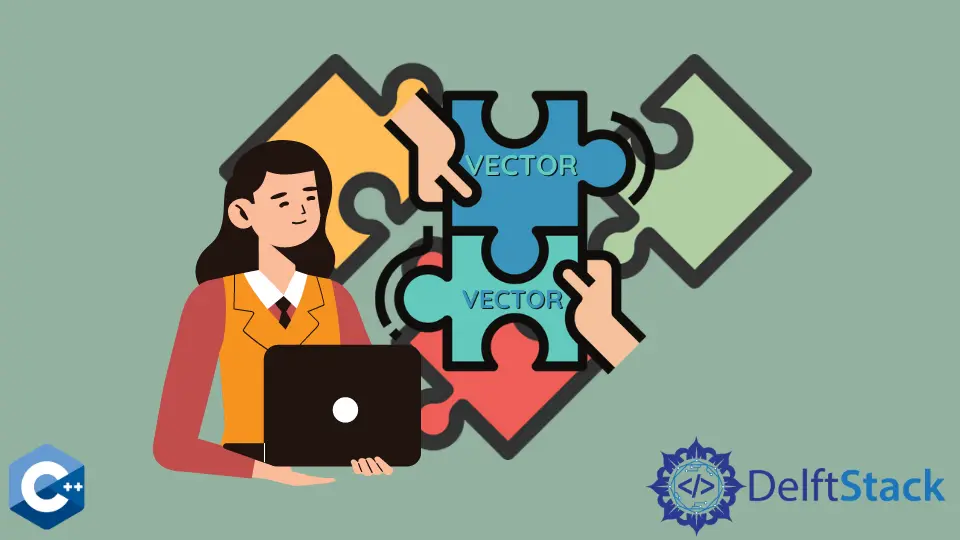
C++ provides a versatile and efficient standard template library (STL) that includes a powerful container called a vector. Vectors are dynamic arrays that can be resized dynamically, making them a popular choice for managing collections of elements.
In certain scenarios, you might need to append the contents of one vector to another. This article will explain several methods of how to append a vector to another vector in C++.
Append a Vector to Another Vector in C++ Using the insert
Function
The insert
function provides an efficient way to append the contents of one vector to another in C++. The insert
function is part of the std::vector
container and allows us to add elements from one vector to another at a specified position.
Syntax of the insert
Function:
iterator insert(iterator pos, const T& value);
iterator insert(iterator pos, size_type count, const T& value);
iterator insert(iterator pos, InputIt first, InputIt last);
Where:
pos
: Iterator pointing to the position where elements will be inserted.value
: The element or value to be inserted.count
: Number of elements to insert.first
andlast
: Range of elements to insert.
The insert
function takes an iterator position and a range of elements to insert. By specifying the end iterator of the source vector as the range, we can effectively append the entire vector.
Example 1: Appending Vectors Using the insert
Function
Let’s consider a scenario where we have two integer vectors, i_vec1
and i_vec2
, and we want to append the elements of i_vec2
to the end of i_vec1
.
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> i_vec1 = {1, 2, 3};
vector<int> i_vec2 = {4, 5, 6};
// Append i_vec2 to i_vec1 using insert
i_vec1.insert(i_vec1.end(), i_vec2.begin(), i_vec2.end());
// Display the result
for (const auto& element : i_vec1) {
cout << element << " ";
}
return 0;
}
In this example, initially, two vectors, i_vec1
with elements {1, 2, 3}
and i_vec2
with elements {4, 5, 6}
, are defined. The insert
function is then employed to append the entire range of i_vec2
to the end of i_vec1
by specifying iterators as arguments.
Finally, the result is displayed by iterating through the combined vector (i_vec1
) using a range-based for
loop.
Output:
Example 2: Generic Approach Using the std::end
and the std::begin
Functions
A more generic approach involves using std::end
and std::begin
to specify iterators, allowing flexibility in passing arguments to the insert
function.
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> i_vec1 = {1, 2, 3};
vector<int> i_vec2 = {4, 5, 6};
// Append i_vec2 to i_vec1 using insert with std::end and std::begin
i_vec1.insert(end(i_vec1), begin(i_vec2), end(i_vec2));
// Display the result
for (const auto& element : i_vec1) {
cout << element << " ";
}
return 0;
}
This example builds upon the previous one but adopts a more generic approach by using the std::end
and std::begin
functions to obtain iterators. The vectors i_vec1
and i_vec2
are the same as in Example 1.
The insert
function is applied to append the elements of i_vec2
to the end of i_vec1
. However, instead of explicitly using i_vec1.end()
, std::end(i_vec1)
is employed for enhanced generality.
The output, displayed using a range-based for
loop, remains identical to Example 1.
Output:
Example 3: Adding Elements With a Specified Value
The insert
function can also be used to add a range of elements with a given value at a specified position in the vector.
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> i_vec = {1, 2, 3, 4, 5};
// Insert four zeros at the beginning of i_vec
i_vec.insert(i_vec.begin(), 4, 0);
// Display the result
for (const auto& element : i_vec) {
cout << element << " ";
}
return 0;
}
In this example, the integer vector i_vec
is initially defined with elements {1, 2, 3, 4, 5}
. The insert
function is applied with i_vec.begin()
specifying the insertion position and 4
indicating the count of zeros to be added.
The output, obtained by iterating through the modified vector, reveals the following result.
Output:
Example 4: Concatenating String Vectors
The insert
function is versatile and can also be applied to concatenate vectors of different types, such as strings.
#include <iostream>
#include <string>
#include <vector>
using namespace std;
int main() {
vector<string> str_vec1 = {"apple", "orange"};
vector<string> str_vec2 = {"banana", "grape"};
// Concatenate str_vec2 to str_vec1
str_vec1.insert(str_vec1.end(), str_vec2.begin(), str_vec2.end());
// Display the result
for (const auto& element : str_vec1) {
cout << element << " ";
}
return 0;
}
Here, two string vectors, str_vec1
with elements {"apple", "orange"}
and str_vec2
with elements {"banana", "grape"}
, are defined. Using the str_vec1.end()
as the insertion point and str_vec2.begin()
with the str_vec2.end()
as the range, the insert
function seamlessly combines the string vectors.
The output is also displayed using a range-based for
loop.
Output:
The insert
function in C++ provides a flexible and efficient way to append vectors. Whether you’re merging numeric vectors, adding elements with a specific value, or concatenating vectors of different types, the insert
function simplifies the process, enhancing code readability and maintainability.
Append a Vector to Another Vector in C++ Using the std::copy
Algorithm
In C++, the std::copy
algorithm provides an alternative and concise way to append one vector to another. This algorithm is part of the <algorithm>
header and offers a straightforward approach to copying elements from one range to another.
Syntax of the std::copy
Algorithm:
template <class InputIt, class OutputIt>
OutputIt copy(InputIt first, InputIt last, OutputIt d_first);
Where:
first
andlast
: Define the range of elements to copy.d_first
: Iterator pointing to the beginning of the destination range.
Example 1: Appending Vectors Using the std::copy
Function
In this example, we have two integer vectors, i_vec1
and i_vec2
, initially containing {1, 2, 3}
and {4, 5, 6}
, respectively. The goal is to append the elements of i_vec2
to the end of i_vec1
using the std::copy
algorithm.
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> i_vec1 = {1, 2, 3};
vector<int> i_vec2 = {4, 5, 6};
// Use std::copy to append i_vec2 to i_vec1
copy(i_vec2.begin(), i_vec2.end(), back_inserter(i_vec1));
// Display the result
for (const auto& element : i_vec1) {
cout << element << " ";
}
return 0;
}
In this example, the std::copy
algorithm is applied to copy the elements from the range defined by i_vec2.begin()
and i_vec2.end()
to the end of i_vec1
.
The back_inserter
function ensures that the elements are appended to the destination vector (i_vec1
). The final output demonstrates the successful appending of vectors.
Output:
Example 2: Concatenating String Vectors
This example showcases the versatility of the std::copy
algorithm by concatenating two vectors of strings, str_vec1
and str_vec2
.
#include <algorithm>
#include <iostream>
#include <string>
#include <vector>
using namespace std;
int main() {
vector<string> str_vec1 = {"apple", "orange"};
vector<string> str_vec2 = {"banana", "grape"};
// Use std::copy to concatenate str_vec2 to str_vec1
copy(str_vec2.begin(), str_vec2.end(), back_inserter(str_vec1));
// Display the result
for (const auto& element : str_vec1) {
cout << element << " ";
}
return 0;
}
Here, the std::copy
algorithm efficiently concatenates the string vectors. The back_inserter
function ensures that the elements from str_vec2
are appended to the end of str_vec1
.
The output reflects the successful concatenation of the string vectors.
Output:
Example 3: Generic Approach With Different Data Types
The std::copy
algorithm can seamlessly handle vectors of different data types, as demonstrated in this example.
#include <algorithm>
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> int_vec = {1, 2, 3};
vector<double> double_vec = {4.0, 5.0, 6.0};
// Use std::copy to append double_vec to int_vec
copy(double_vec.begin(), double_vec.end(), back_inserter(int_vec));
// Display the result
for (const auto& element : int_vec) {
cout << element << " ";
}
return 0;
}
Here, we illustrate the generic capability of the std::copy
algorithm to handle vectors with different data types. Two vectors, int_vec
with integer elements and double_vec
with double elements, are used.
The copy
function appends the elements of double_vec
to the end of int_vec
using the back_inserter
function. The output, displayed using a for
loop, shows the successfully appended vector, highlighting the algorithm’s ability to handle different data types seamlessly.
Output:
The std::copy
algorithm provides a concise and flexible way to append one vector to another in C++. Its simplicity and compatibility with various data types make it a versatile choice for copying and concatenating vectors.
Append a Vector to Another Vector in C++ Using a Range-Based Loop
In C++, a range-based loop provides a concise and readable way to iterate through the elements of a container. Leveraging this loop structure, we can easily append one vector to another.
Syntax of a Range-Based Loop:
for (const auto& element : source_vector) {
destination_vector.push_back(element);
}
Where:
source_vector
: The vector from which elements are extracted.destination_vector
: The vector to which elements are appended.
Example: Appending Vectors Using a Range-Based Loop
In this example, we have two integer vectors, i_vec1
and i_vec2
, initialized with values {1, 2, 3}
and {4, 5, 6}
, respectively. The objective is to append the elements of i_vec2
to the end of i_vec1
using a range-based loop.
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> i_vec1 = {1, 2, 3};
vector<int> i_vec2 = {4, 5, 6};
// Append i_vec2 to i_vec1 using a range-based loop
for (const auto& element : i_vec2) {
i_vec1.push_back(element);
}
// Display the result
for (const auto& element : i_vec1) {
cout << element << " ";
}
return 0;
}
In this C++ code snippet, we use the loop syntax for (const auto& element : i_vec2)
to iterate through each element in i_vec2
. For each iteration, we use the push_back
method to append the current element to the end of i_vec1
.
This loop effectively appends all elements of i_vec2
to the end of i_vec1
.
Following the range-based loop, we proceed to display the result. Another range-based loop is used to iterate through the elements of the modified i_vec1
, and the cout
statement prints each element followed by a space.
The final output, displayed in the console, demonstrates the successful appending of vectors.
Output:
A range-based loop provides a clean and straightforward approach to append one vector to another in C++. The concise syntax enhances code readability, and its simplicity makes it an appealing choice for appending vectors.
Conclusion
Appending one vector to another in C++ can be achieved through various methods, each offering its advantages.
The insert
function, a versatile tool within the std::vector
container, provides flexibility for merging vectors by specifying insertion points and ranges. The std::copy
algorithm simplifies the process, offering a concise and readable solution for copying elements from one vector to another.
Additionally, the use of a range-based loop provides an elegant and straightforward way to iterate through elements and append vectors seamlessly. Whether you choose the insert
function, std::copy
algorithm, or a range-based loop, the key lies in selecting the approach that aligns best with your code style and specific requirements.
With these methods at your disposal, you have the tools to efficiently modify and concatenate vectors in C++, enhancing the flexibility and readability of your code.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook