How to Get File Size in C
-
Get File Size in C Using the
stat
Function -
Get File Size in C Using the
fstat
Function -
Get File Size in C Using
fseek
andftell
Functions -
Get File Size in C Using Windows API
GetFileSize
Function - Conclusion
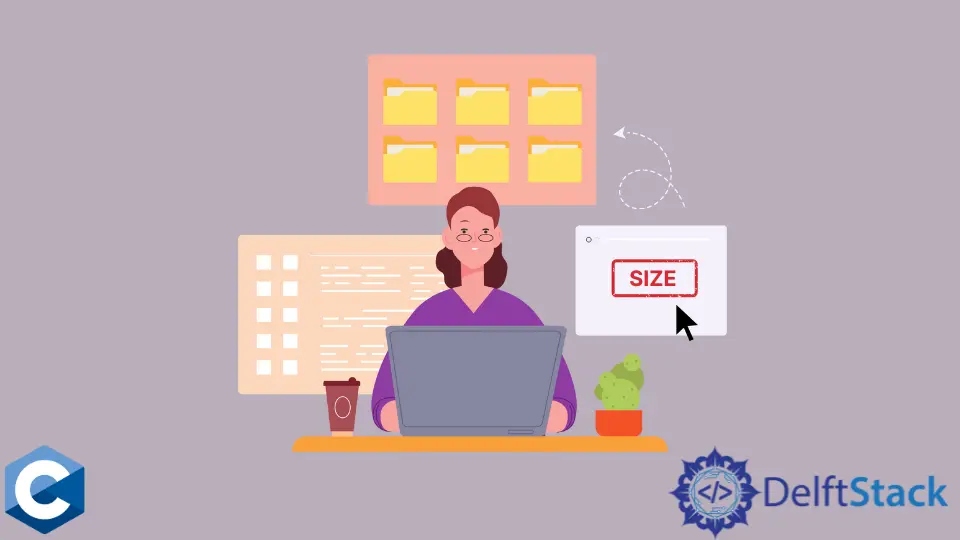
Retrieving the size of a file is a fundamental operation in programming, often required for various file manipulation tasks. In the C programming language, there are several methods and functions available to determine the size of a file.
Each method comes with its advantages and is suitable for different scenarios.
In this article, we will explore various approaches to getting file size in C, ranging from standard C library functions to platform-specific APIs.
Get File Size in C Using the stat
Function
One efficient way to get the size of a file in C is by utilizing the stat
function. The stat
function is a POSIX-compliant system call that retrieves various attributes of a specified file.
The relevant member for the file size in the struct stat
is st_size
. Here is the basic syntax for using stat
and accessing the file size:
#include <sys/stat.h>
int stat(const char *pathname, struct stat *statbuf);
// Accessing file size using st_size
off_t st_size;
Parameters:
pathname
: A pointer to a string containing the path of the file whose information we want to retrieve.statbuf
: A pointer to astruct stat
where the function stores the file information.
Once the stat
function is successfully executed, you can access the file size through the st_size
member of the returned structure.
Let’s explore two examples demonstrating how to use the stat
function to retrieve the file size. The first example showcases a hardcoded filename, while the second allows the user to pass the filename as a program parameter.
Example 1: Hardcoded Filename
Here, we demonstrate how to use the stat
function to retrieve the size of a file when the filename is hardcoded within the program.
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <time.h>
const char *filename = "example.txt";
int main() {
struct stat sb;
if (stat(filename, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
printf("File Size: %ld bytes\n", sb.st_size);
exit(EXIT_SUCCESS);
}
In this example, we include the necessary headers and declare a struct stat
variable (sb
) to store file information. The stat
function is called with the hardcoded filename (example.txt
) and the address of the struct stat
variable.
If the function returns -1
, indicating failure, an error message is printed using perror
, and the program exits with a failure status. Otherwise, the file size is printed using printf
and the st_size
member of the structure.
Code Output:
Example 2: User-Specified Filename
In this example, we extend the functionality to allow users to specify the filename as a parameter when running the program.
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <time.h>
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s <filename>\n", argv[0]);
exit(EXIT_FAILURE);
}
const char *filename = argv[1];
struct stat sb;
if (stat(filename, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
printf("File Size: %ld bytes\n", sb.st_size);
exit(EXIT_SUCCESS);
}
In this example, we modify the program to accept the filename as a command-line argument. The number of command-line arguments is checked, and if it’s not equal to 2 (program name + filename), a usage message is printed, and the program exits with a failure status.
The user-specified filename is then obtained from the command-line arguments, and the rest of the process is similar to Example 1. The stat
function is called with the user-specified filename, and the file size is printed using printf
and the st_size
member of the structure.
Here, the output would be similar:
This output confirms the successful retrieval and display of the file size using the stat
function in a C program. Incorporating this code into your projects allows for efficient access to crucial file attributes, supporting various file manipulation tasks.
Get File Size in C Using the fstat
Function
While the stat
function is powerful for obtaining file information, an alternative method involves using the fstat
function. This function is particularly useful when dealing with file descriptors.
The fstat
function, declared in the <sys/stat.h>
header, is employed to obtain information about an open file identified by a file descriptor. Similar to stat
, the st_size
member in the resulting struct stat
holds the file size.
The syntax is as follows:
#include <fcntl.h>
#include <sys/stat.h>
int fstat(int fd, struct stat *statbuf);
// Accessing file size using st_size
off_t st_size;
Parameters:
fd
: The file descriptor for the open file.statbuf
: A pointer to astruct stat
where the function stores the file information.
After a successful fstat
call, the file size can be extracted from the st_size
member.
Let’s delve into an example showcasing the usage of the fstat
function to retrieve the size of a file.
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <time.h>
#include <unistd.h>
const char *filename = "example.txt";
int main() {
int fd = open(filename, O_RDONLY);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
struct stat sb;
if (fstat(fd, &sb) == -1) {
perror("fstat");
close(fd);
exit(EXIT_FAILURE);
}
printf("File Size: %ld bytes\n", sb.st_size);
close(fd);
exit(EXIT_SUCCESS);
}
In this example, we begin by opening the file using the open
system call, which returns a file descriptor (fd
). If the open operation fails, an error message is printed, and the program exits with a failure status.
Subsequently, we declare a struct stat
variable (sb
) to store the file information. The fstat
function is then called with the file descriptor and the address of the struct stat
variable.
In case of a failure (indicated by the function returning -1
), an error message is printed using perror
, and the program exits with a failure status. Otherwise, the file size is extracted from the st_size
member of the struct stat
and printed to the console using printf
.
Finally, the opened file is closed using the close
system call.
The output of the program will be similar to the following:
This example demonstrates the usage of the fstat
function to retrieve file size, offering an alternative approach to obtaining file information in a C program.
Get File Size in C Using fseek
and ftell
Functions
In addition to the stat
and fstat
functions, another approach to obtaining the file size in C involves using the fseek
and ftell
functions. These functions operate on a FILE
stream, providing a straightforward way to navigate through a file and determine its size.
The fseek
function, found in the <stdio.h>
header, is used to set the file position indicator for the specified stream. The ftell
function, also from <stdio.h>
, returns the current value of the file position indicator.
Combining these functions allows us to determine the file size. The syntax is as follows:
#include <stdio.h>
int fseek(FILE *stream, long offset, int whence);
long ftell(FILE *stream);
Parameters:
stream
: A pointer to theFILE
stream.offset
: The number of bytes to move the file position indicator.whence
: The reference point for the offset (SEEK_SET
,SEEK_CUR
, orSEEK_END
).
Let’s explore a complete example demonstrating the use of fseek
and ftell
functions to retrieve the size of a file.
#include <stdio.h>
#include <stdlib.h>
const char *filename = "example.txt";
long getFileSize(FILE *file) {
long size;
// Move the file pointer to the end of the file
fseek(file, 0, SEEK_END);
// Get the current position of the file pointer (which is the size of the
// file)
size = ftell(file);
// Move the file pointer back to the beginning of the file
fseek(file, 0, SEEK_SET);
return size;
}
int main() {
FILE *file = fopen(filename, "rb");
if (file == NULL) {
perror("fopen");
exit(EXIT_FAILURE);
}
long size = getFileSize(file);
printf("File Size: %ld bytes\n", size);
fclose(file);
exit(EXIT_SUCCESS);
}
In this example, we start by opening the file in binary read mode ("rb"
) using the fopen
function, and a FILE
stream is obtained. If the file opening operation fails, an error message is printed, and the program exits with a failure status.
We then define a function, getFileSize
, that takes a FILE
stream as an argument and utilizes fseek
and ftell
to determine the file size. The function moves the file pointer to the end of the file, retrieves the current position (which is the file size), and then resets the file pointer to the beginning.
In the main
function, we call getFileSize
with the opened file, obtain the file size, and print it to the console using printf
. Finally, the file is closed with fclose
.
The output of the program will be similar to the following:
This example demonstrates how to use the fseek
and ftell
functions to efficiently determine the file size in a C program, providing an alternative method to the stat
and fstat
approaches.
Get File Size in C Using Windows API GetFileSize
Function
For developers working in a Windows environment, an effective way to obtain file size is through the use of the Windows API GetFileSize
function. This function provides a straightforward mechanism to retrieve the size of a file.
The GetFileSize
function is part of the Windows API and is declared in the <windows.h>
header. Its syntax is as follows:
#include <windows.h>
DWORD GetFileSize(HANDLE hFile, LPDWORD lpFileSizeHigh);
Where:
hFile
: A handle for the file whose size is to be retrieved.lpFileSizeHigh
: A pointer to the high-order DWORD of the file size.
Let’s examine a complete example that showcases the use of the GetFileSize
function to obtain the size of a file.
#include <stdio.h>
#include <windows.h>
const char *filename = "example.txt";
int main() {
HANDLE hFile = CreateFile(filename, GENERIC_READ, FILE_SHARE_READ, NULL,
OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
if (hFile == INVALID_HANDLE_VALUE) {
perror("CreateFile");
return EXIT_FAILURE;
}
DWORD fileSize = GetFileSize(hFile, NULL);
if (fileSize == INVALID_FILE_SIZE) {
perror("GetFileSize");
CloseHandle(hFile);
return EXIT_FAILURE;
}
printf("File Size: %lu bytes\n", fileSize);
CloseHandle(hFile);
return EXIT_SUCCESS;
}
In this example, we begin by creating a file handle (hFile
) using the CreateFile
function. If the file creation fails, an error message is printed, and the program returns with a failure status.
Next, we use the GetFileSize
function to retrieve the size of the file associated with the file handle. If GetFileSize
returns INVALID_FILE_SIZE
, indicating an error, an error message is printed, and the file handle is closed using CloseHandle
. The program then returns with a failure status.
Upon successful execution of GetFileSize
, the obtained file size is printed to the console using printf
. Finally, the file handle is closed with CloseHandle
.
Code Output:
This example illustrates how to use the Windows API GetFileSize
function to efficiently determine the file size in a C program. Developers working in a Windows environment can leverage this approach for seamless file size retrieval.
Conclusion
Obtaining the file size in C involves a diverse set of methods, each with its own merits and use cases, whether utilizing standard C library functions like fseek
and ftell
, platform-specific functions such as stat
and fstat
, or leveraging Windows API functions like GetFileSize
, you can choose the method that aligns with your programming environment and project requirements.
A solid understanding of these file size retrieval techniques allows you to efficiently manage file-related operations in your C programs.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook