在 C 语言中获取文件大小
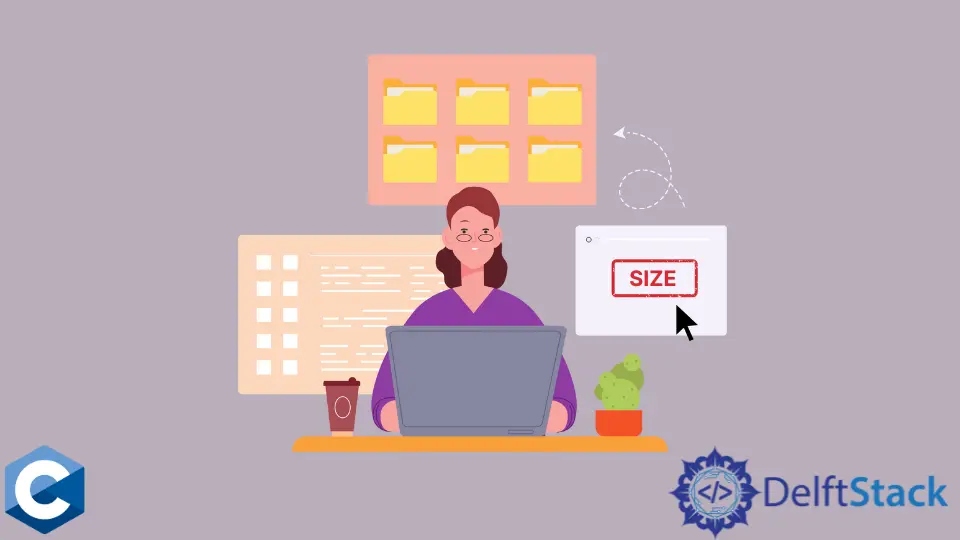
本文将介绍几种在 C 语言中获取文件大小的方法。
使用 stat
函数在 C 语言中获取文件大小
stat
系统调用是符合 POSIX 标准的函数,可以用来检索给定文件的各种属性。它需要两个参数-第一个参数是 char
指针,它应该指向文件的路径名,第二个参数是 struct stat
指针类型,在函数手册中有广泛的描述。当函数调用成功返回后,用户可以通过直接访问 struct stat
中的成员,从 struct stat
中提取文件所需的属性。文件大小存储在结构的 st_size
成员中。需要注意的是,stat
函数不需要对文件的权限进行检索,但调用者应该对路径名中包含的每个目录都有执行权限。
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <time.h>
const char *filename = "input.txt";
int main(int argc, char *argv[]) {
struct stat sb;
if (stat(filename, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
printf("Inode number: %lu\n", sb.st_ino);
printf("User ID of owner: %u\n", sb.st_uid);
printf("Group ID of owner: %u\n", sb.st_gid);
printf("Total file size: %lu bytes\n", sb.st_size);
printf("Last status change: %s", ctime(&sb.st_ctime));
printf("Last file access: %s", ctime(&sb.st_atime));
printf("Last file modification: %s", ctime(&sb.st_mtime));
exit(EXIT_SUCCESS);
}
输出:
Inode number: 12638061
User ID of owner: 1000
Group ID of owner: 1000
Total file size: 126 bytes
Last status change: Sat Feb 6 23:35:02 2021
Last file access: Sat Feb 6 23:35:02 2021
Last file modification: Sat Feb 6 23:35:02 2021
st_size
成员表示文件的总大小,单位是字节。如果给定的路径名是符号链接,这个成员表示路径名指针的长度,由链接表示。我们实现了一个例子,在上面的代码示例中,从 const
声明的硬编码字符串值中获取文件名,尽管用户可以将文件名作为程序参数传递,如下面的例子所示。stat
调用失败时返回 -1
,并相应设置 errno
,否则返回 0 表示成功。
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <time.h>
int main(int argc, char *argv[]) {
struct stat sb;
char *filename = NULL;
if (argc != 2) {
printf("Usage: ./program filename\n");
exit(EXIT_FAILURE);
}
filename = argv[1];
if (stat(filename, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
printf("Inode number: %lu\n", sb.st_ino);
printf("User ID of owner: %u\n", sb.st_uid);
printf("Group ID of owner: %u\n", sb.st_gid);
printf("Total file size: %lu bytes\n", sb.st_size);
printf("Last status change: %s", ctime(&sb.st_ctime));
printf("Last file access: %s", ctime(&sb.st_atime));
printf("Last file modification: %s", ctime(&sb.st_mtime));
exit(EXIT_SUCCESS);
}
使用 fstat
函数获取 C 语言中的文件大小
另外,我们也可以使用 fstat
函数,它以文件描述符作为第一个参数来指定目标文件。文件描述符可以通过 open
系统调用获取,但当不需要打开的文件时,一定要用 close
系统调用关闭。注意,本文所有的例子都打印了大部分的 struct stat
成员。你可能需要使用附加函数,如 st_mode
和 st_dev
来提取相关的属性信息。
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <time.h>
const char *filename = "input.txt";
int main(int argc, char *argv[]) {
struct stat sb;
int fd = open(filename, O_RDONLY);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
if (fstat(fd, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
printf("Inode number: %lu\n", sb.st_ino);
printf("User ID of owner: %u\n", sb.st_uid);
printf("Group ID of owner: %u\n", sb.st_gid);
printf("Total file size: %lu bytes\n", sb.st_size);
printf("Last status change: %s", ctime(&sb.st_ctime));
printf("Last file access: %s", ctime(&sb.st_atime));
printf("Last file modification: %s", ctime(&sb.st_mtime));
close(fd);
exit(EXIT_SUCCESS);
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu