在 C 語言中獲取檔案大小
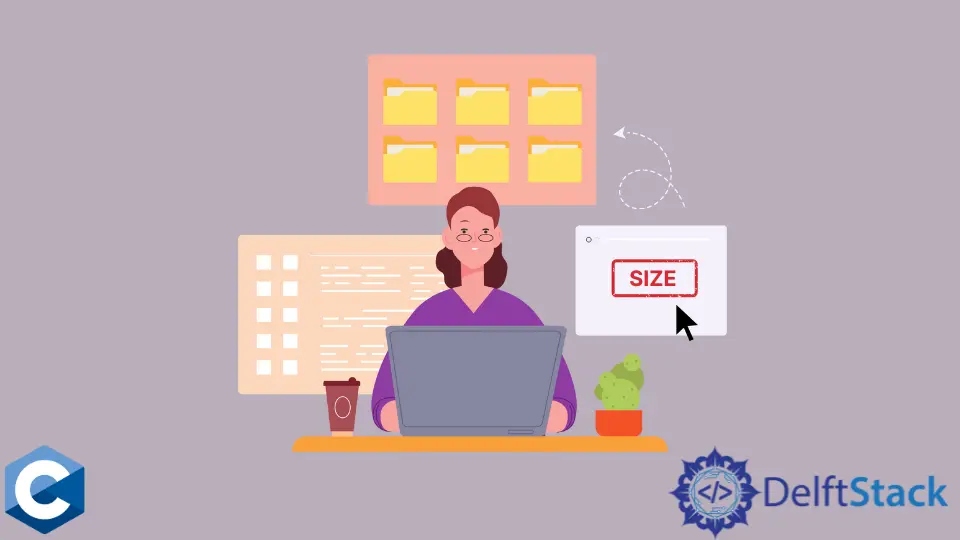
本文將介紹幾種在 C 語言中獲取檔案大小的方法。
使用 stat
函式在 C 語言中獲取檔案大小
stat
系統呼叫是符合 POSIX 標準的函式,可以用來檢索給定檔案的各種屬性。它需要兩個引數-第一個引數是 char
指標,它應該指向檔案的路徑名,第二個引數是 struct stat
指標型別,在函式手冊中有廣泛的描述。當函式呼叫成功返回後,使用者可以通過直接訪問 struct stat
中的成員,從 struct stat
中提取檔案所需的屬性。檔案大小儲存在結構的 st_size
成員中。需要注意的是,stat
函式不需要對檔案的許可權進行檢索,但呼叫者應該對路徑名中包含的每個目錄都有執行許可權。
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <time.h>
const char *filename = "input.txt";
int main(int argc, char *argv[]) {
struct stat sb;
if (stat(filename, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
printf("Inode number: %lu\n", sb.st_ino);
printf("User ID of owner: %u\n", sb.st_uid);
printf("Group ID of owner: %u\n", sb.st_gid);
printf("Total file size: %lu bytes\n", sb.st_size);
printf("Last status change: %s", ctime(&sb.st_ctime));
printf("Last file access: %s", ctime(&sb.st_atime));
printf("Last file modification: %s", ctime(&sb.st_mtime));
exit(EXIT_SUCCESS);
}
輸出:
Inode number: 12638061
User ID of owner: 1000
Group ID of owner: 1000
Total file size: 126 bytes
Last status change: Sat Feb 6 23:35:02 2021
Last file access: Sat Feb 6 23:35:02 2021
Last file modification: Sat Feb 6 23:35:02 2021
st_size
成員表示檔案的總大小,單位是位元組。如果給定的路徑名是符號連結,這個成員表示路徑名指標的長度,由連結表示。我們實現了一個例子,在上面的程式碼示例中,從 const
宣告的硬編碼字串值中獲取檔名,儘管使用者可以將檔名作為程式引數傳遞,如下面的例子所示。stat
呼叫失敗時返回 -1
,並相應設定 errno
,否則返回 0 表示成功。
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <time.h>
int main(int argc, char *argv[]) {
struct stat sb;
char *filename = NULL;
if (argc != 2) {
printf("Usage: ./program filename\n");
exit(EXIT_FAILURE);
}
filename = argv[1];
if (stat(filename, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
printf("Inode number: %lu\n", sb.st_ino);
printf("User ID of owner: %u\n", sb.st_uid);
printf("Group ID of owner: %u\n", sb.st_gid);
printf("Total file size: %lu bytes\n", sb.st_size);
printf("Last status change: %s", ctime(&sb.st_ctime));
printf("Last file access: %s", ctime(&sb.st_atime));
printf("Last file modification: %s", ctime(&sb.st_mtime));
exit(EXIT_SUCCESS);
}
使用 fstat
函式獲取 C 語言中的檔案大小
另外,我們也可以使用 fstat
函式,它以檔案描述符作為第一個引數來指定目標檔案。檔案描述符可以通過 open
系統呼叫獲取,但當不需要開啟的檔案時,一定要用 close
系統呼叫關閉。注意,本文所有的例子都列印了大部分的 struct stat
成員。你可能需要使用附加函式,如 st_mode
和 st_dev
來提取相關的屬性資訊。
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
#include <time.h>
const char *filename = "input.txt";
int main(int argc, char *argv[]) {
struct stat sb;
int fd = open(filename, O_RDONLY);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
if (fstat(fd, &sb) == -1) {
perror("stat");
exit(EXIT_FAILURE);
}
printf("Inode number: %lu\n", sb.st_ino);
printf("User ID of owner: %u\n", sb.st_uid);
printf("Group ID of owner: %u\n", sb.st_gid);
printf("Total file size: %lu bytes\n", sb.st_size);
printf("Last status change: %s", ctime(&sb.st_ctime));
printf("Last file access: %s", ctime(&sb.st_atime));
printf("Last file modification: %s", ctime(&sb.st_mtime));
close(fd);
exit(EXIT_SUCCESS);
}
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jinku Hu