How to Get Extended Attributes of File in C
-
Use the
getxattr
andlistxattr
Functions to Get Extended Attributes of a File in C -
Use the
setxattr
Function to Set Extended Attributes on a File in C -
Use the
getfattr
Command-Line Utility to Show Extended Attributes of a File in C - Considerations and Best Practices
- Conclusion
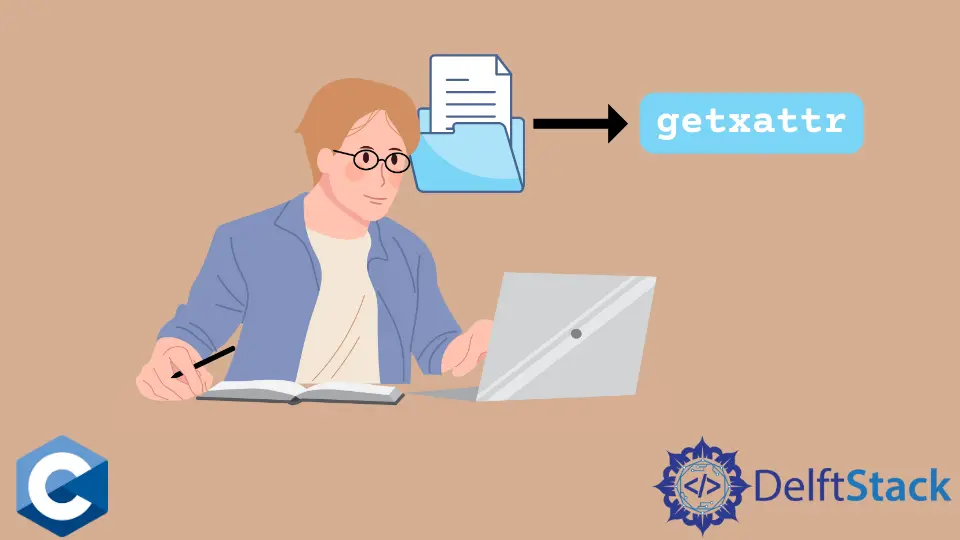
Extended attributes are extra pieces of information that can be associated with files and directories on certain filesystems. They are often used to store metadata that goes beyond traditional file attributes. Examples include file tags, security information, or custom application-specific data.
Extended attributes, also known as extended file attributes or xattrs
, provide a way to associate additional metadata with files on certain filesystems. In C programming, accessing and manipulating extended attributes can be achieved through various methods.
This article will delve into different approaches for obtaining extended attributes of a file in C, providing comprehensive explanations and example codes for each method. We will explain several methods of how to get extended attributes of a file in C.
Use the getxattr
and listxattr
Functions to Get Extended Attributes of a File in C
Some UNIX-based systems provide extended attributes for the files, representing name-value pairs of metadata that can be associated with the file. Extended attributes require the underlying file system support, but the common filesystem types have this feature.
Extended attributes have the specific format of type - namespace.name
, where namespace
is utilized to group functionally similar ones, while the name
part identifies an individual extended attribute. A namespace can have the following values: user
, trusted
, system
, and security
, which distinguish several permission types as a convention (described in detail on the page).
In this case, we implement an example program that takes multiple filenames as command-line arguments and consequently retrieves all extended attributes for each file. Notice that the program processes the multiple file arguments with the getopt
function.
The getxattr
function is part of the <sys/xattr.h>
header and is commonly used on Unix-like systems to retrieve the value of an extended attribute associated with a file. The getxattr
function takes four arguments to retrieve an extended attribute value.
The first argument specifies a path in the filesystem to indicate the file for which EAs should be retrieved, and the second argument specifies an attribute name. The last two arguments are the pointer to the location where the value will be stored by getxattr
and the available buffer size on this address.
Note that the size of the attribute value can be retrieved by passing the value 0
as a size argument, and the return value of the call is the number of bytes for the needed buffer. However, when the second call is invoked to retrieve the value, it might be changed and have a different size; thus, it’s better to check for the error codes to guarantee faultless execution.
On the other hand, the listxattr
function retrieves the list of extended attribute names for the given file path. It takes three arguments, the first of which is the file path.
The next two arguments represent the buffer where the retrieved list will be stored, and its size will be specified in bytes. Note that this buffer is caller-allocated, and the caller is responsible for allocating sufficient memory.
Also, similar to the getxattr
, one can pass the value 0
as the third argument to this function, and the returned value will be the buffer size needed to store the full list.
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/xattr.h>
#include <unistd.h>
#define XATTR_SIZE 10000
#define errExit(msg) \
do { \
perror(msg); \
exit(EXIT_FAILURE); \
} while (0)
static void usageError(char *progName) {
fprintf(stderr, "Usage: %s [-x] file...\n", progName);
exit(EXIT_FAILURE);
}
int main(int argc, char *argv[]) {
char list[XATTR_SIZE], value[XATTR_SIZE];
ssize_t listLen, valueLen;
int ns, j, k, opt;
bool hexDisplay;
hexDisplay = 0;
while ((opt = getopt(argc, argv, "x")) != -1) {
switch (opt) {
case 'x':
hexDisplay = 1;
break;
case '?':
usageError(argv[0]);
}
}
if (optind >= argc) usageError(argv[0]);
for (j = optind; j < argc; j++) {
listLen = listxattr(argv[j], list, XATTR_SIZE);
if (listLen == -1) errExit("listxattr");
printf("%s:\n", argv[j]);
for (ns = 0; ns < listLen; ns += strlen(&list[ns]) + 1) {
printf(" name=%s; ", &list[ns]);
valueLen = getxattr(argv[j], &list[ns], value, XATTR_SIZE);
if (valueLen == -1) {
printf("couldn't get value");
} else if (!hexDisplay) {
printf("value=%.*s", (int)valueLen, value);
} else {
printf("value=");
for (k = 0; k < valueLen; k++) printf("%02x ", (unsigned char)value[k]);
}
printf("\n");
}
printf("\n");
}
exit(EXIT_SUCCESS);
}
This C program is a command-line utility designed to retrieve and display extended attributes (xattrs
) of one or more specified files. It uses the listxattr
and getxattr
functions to obtain the attribute names and values.
The program supports an optional -x
command-line option, which, when specified, displays attribute values in hexadecimal format. The extended attributes are printed for each specified file, including the attribute names and their corresponding values.
Error handling is implemented to manage potential issues during attribute retrieval. The program exits with a success status if execution is successful and with a failure status if any errors occur.
Overall, it provides a practical way to inspect extended attributes associated with files on supported filesystems.
Use the setxattr
Function to Set Extended Attributes on a File in C
Alternatively, we can set arbitrary attributes to the files using the setxattr
function, which takes the file’s pathname and name-value pair as separate arguments, the size of the value, and an integer that specifies the predefined values for different set operations.
If the last argument is zero, which is the default value, the extended attribute is created if it does not exist; otherwise, the value is replaced.
The XATTR_CREATE
macro can be specified to indicate only the create option, which will fail if the attribute exists, and XATTR_REPLACE
to perform replacement, which fails if the attribute does not exist.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/xattr.h>
#define errExit(msg) \
do { \
perror(msg); \
exit(EXIT_FAILURE); \
} while (0)
static void usageError(char *progName) {
fprintf(stderr, "Usage: %s file\n", progName);
exit(EXIT_FAILURE);
}
int main(int argc, char *argv[]) {
char *value;
if (argc < 2 || strcmp(argv[1], "--help") == 0) usageError(argv[0]);
value = "x attribute value";
if (setxattr(argv[1], "user.x", value, strlen(value), 0) == -1)
errExit("setxattr");
value = "y attribute value";
if (setxattr(argv[1], "user.y", value, strlen(value), 0) == -1)
errExit("setxattr");
exit(EXIT_SUCCESS);
}
This C program is a simple utility for setting extended attributes (xattrs
) on a specified file. The program expects a filename as a command-line argument and sets two user-defined extended attributes ("user.x"
and "user.y"
) with corresponding string values on that file.
If the command-line argument is missing or if it is "--help"
, the program displays a usage error. The setxattr
function is used to set the extended attributes, and the errExit
macro handles errors by printing an error message and exiting with a failure status.
The program exits with a success status after setting the attributes. In summary, this code demonstrates how to use the setxattr
function to associate custom metadata with a file on filesystems that support extended attributes.
Use the getfattr
Command-Line Utility to Show Extended Attributes of a File in C
The getfattr
command-line utility can be used to display extended attributes of a file. While not a direct C function, it’s a practical way to interact with xattrs
.
The getfattr
utility is a powerful tool that comes pre-installed on many Unix-like operating systems. It allows users to retrieve and display extended attributes of files and directories.
The basic syntax is:
getfattr[options] file
Here, file
represents the path to the file or directory whose extended attributes you want to inspect.
In order to incorporate the functionality of getfattr
into a C program, the system
function can be employed to execute the command. While this approach involves invoking a command-line utility externally, it can be practical and efficient for displaying extended attributes.
Below is an example C program that utilizes the getfattr
command within the code:
#include <stdio.h>
#include <stdlib.h>
void displayExtendedAttributes(const char *filePath) {
char command[100];
snprintf(command, sizeof(command), "getfattr -d %s", filePath);
// Execute the command using system()
int result = system(command);
if (result == -1) {
perror("Error executing getfattr");
exit(EXIT_FAILURE);
}
}
int main(int argc, char *argv[]) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <file>\n", argv[0]);
exit(EXIT_FAILURE);
}
const char *filePath = argv[1];
// Display extended attributes using getfattr
displayExtendedAttributes(filePath);
return 0;
}
In this code, the function displayExtendedAttributes
constructs a command using snprintf
to invoke getfattr
with the -d
option for displaying attribute values. It executes the command using system()
.
The main function checks if the program is provided with the correct number of command-line arguments. It retrieves the file path from the command line and calls the displayExtendedAttributes
function to showcase the extended attributes.
Considerations and Best Practices
- Check for Compatibility: Ensure that the filesystem and operating system support extended attributes.
- Error Handling: Implement robust error handling to handle potential issues during extended attribute retrieval.
- Security Considerations: Be cautious when dealing with extended attributes, especially if they contain sensitive information.
- Attribute Names: Be aware of the specific attribute names used on the filesystem.
Conclusion
Retrieving extended attributes in C provides a powerful way to access additional metadata associated with files. This guide has explored three methods:
- Using the
getxattr
function to get a specific attribute. - Utilizing the
listxattr
function to obtain a list of attribute names. - Employing the
getfattr
command-line utility for a more external approach.
Each method has its strengths and use cases, offering developers flexibility in handling extended attributes in their C programs.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook