How to open vs fopen in C
-
Use the
fopen
Function to Open or Create a File in C -
Use the
open
Function to Open or Create a File in C -
Use the
creat
Function to Open and Create a File
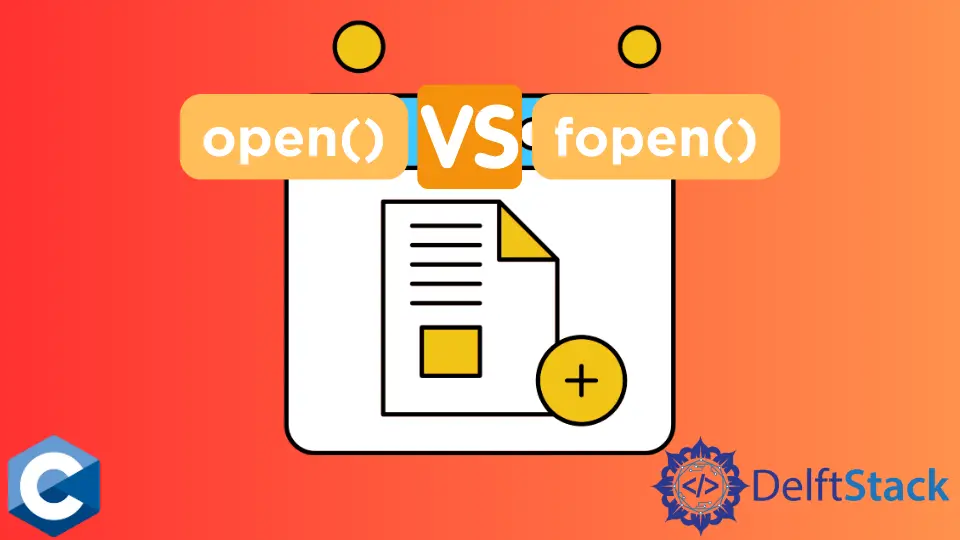
This article will demonstrate multiple methods about using open
vs fopen
functions in C.
Use the fopen
Function to Open or Create a File in C
fopen
is the C standard library function for handling the opening of the files as stream objects. In contrast with the open
function, which is intrinsically a system call, fopen
associates the FILE
pointer object to the given file. It takes two arguments; the first represents a pathname to the file to be opened, and the second argument the mode in which to open the file.
Note that fopen
has multiple predefined values for the mode parameter, all of which are detailed on the function manual page. In the following example code, we specify the w+
mode, which opens the file for reading and writing along with truncating the contents and positioning the stream at the beginning. Note that if the given function path does not exist, the call creates a new file for writing.
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
const char* str = "Arbitrary string to be written to a file.\n";
int main(void) {
const char* filename = "innn.txt";
FILE* output_file = fopen(filename, "w+");
if (!output_file) {
perror("fopen");
exit(EXIT_FAILURE);
}
fwrite(str, 1, strlen(str), output_file);
printf("Done Writing!\n");
fclose(output_file);
exit(EXIT_SUCCESS);
}
Output:
Done Writing!
Use the open
Function to Open or Create a File in C
In contrast, open
function is essentially a lower-level system service called even when fopen
is used. Note that system calls are usually provided to the end-users with C library wrapper functions, but their characteristics and performance use cases differ from those in the C stio
library. E.g. open
takes the second argument as type int
and optional third argument specifying file mode bits when a new file is created. In the following example, we demonstrate the similar functionality as in the previous sample code.
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
const char* str = "Arbitrary string to be written to a file.\n";
int main(void) {
const char* filename = "innn.txt";
int fd = open(filename, O_RDWR | O_CREAT);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
write(fd, str, strlen(str));
printf("Done Writing!\n");
close(fd);
exit(EXIT_SUCCESS);
}
The open
call creates a new file descriptor and returns its value to the caller; otherwise, -1
is returned on failure and errno
set accordingly. The next code example shows the open
call with the optional mode argument specifying S_IRWXU
. These symbols are macros defined in the <fcntl.h>
header and denote the file’s different permission flags. S_IRWXU
implies that the file owner will have read/write/execute permissions on a newly created file.
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
const char* str = "Arbitrary string to be written to a file.\n";
int main(void) {
const char* filename = "innn.txt";
int fd = open(filename, O_CREAT | O_WRONLY | O_APPEND, S_IRWXU);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
write(fd, str, strlen(str));
printf("Done Writing!\n");
close(fd);
exit(EXIT_SUCCESS);
}
Use the creat
Function to Open and Create a File
Another system call included with the open
is the creat
function, which can be specifically used to create a new file, or if the file already exists, truncate it to zero length. Note that this function is essentially equal to the open
call with the following arguments - O_CREAT | O_WRONLY | O_TRUNC
. Thus, it takes only two arguments compared to open
, omitting the second flags argument.
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
const char* str = "Arbitrary string to be written to a file.\n";
int main(void) {
const char* filename = "innn.txt";
int fd = creat(filename, S_IRWXG);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
write(fd, str, strlen(str));
printf("Done Writing!\n");
close(fd);
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook