Array of Objects in Angular
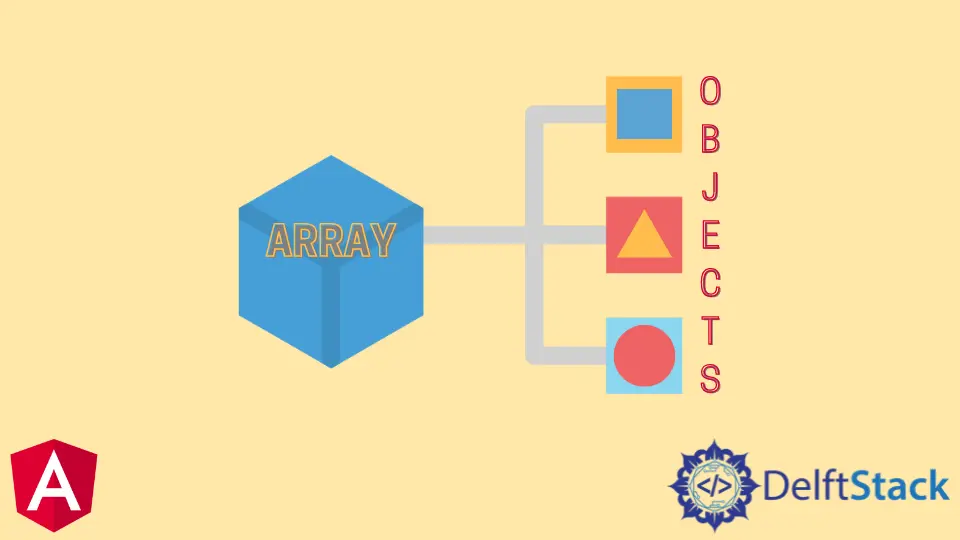
In this article, we discuss the Array of objects in Angular with examples and how it works.
Array of Objects in Angular
While working on Angular applications, there are many situations where we have to create arrays of objects. Let’s start with an example of an array of objects in TypeScript.
We have to declare an array of objects by giving them values and types of Array, to hold an array of objects. An array of objects is populated and show a radio button display or on the Dropdown.
There are many ways we can make an object of an array. We can declare and reset an array by using any object type declared.
The objects have the properties to contain keys and values. We will show how an array of strings can be declared and reset in the given syntax.
# Angular
shapes:Array<string> = ['Triangle','Square','Rectangle'];
Output:
In typescript, we can claim an Object using any type. Let’s create a fruits
object in the following example.
# Angular
public fruits: Array<any> = [
{ title: "Banana", color: "Yellow" },
{ title: "Apple", color: "Red" },
{ title: "Guava", color: "Green" },
{ title: "Strawberry", color: "Red" }
];
The object(<any>
) type can be replaced.
# Angular
public fruits: Array<object> = [
{ title: "Banana", color: "Yellow" },
{ title: "Apple", color: "Red" },
{ title: "Guava", color: "Green" },
{ title: "Strawberry", color: "Red" }
];
Alias Object Type Array in Angular
Operating the type keyword in typescript permits making new alias for a custom type. Designed Fruit
object alias and assembled an array of type alias.
# AngularJs
type Fruit = Array<{ id: number; name: string }>;
In the next example, we create a Fruit
alias of array type and initialize Array with object values.
Code - app.component.ts
:
# Angular
import { Component } from '@angular/core';
type Fruit = Array<{ id: number; name: string }>;
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
fruits: Fruit = [
{ id: 1, name: "Apple" },
{ id: 2, name: "Banana" },
{ id: 3, name: "Guava" },
{ id: 4, name: "Strawberry" }
];
constructor() {}
ngOnInit() {}
}
Now, we display the object on the front end file.
Code - app.component.html
:
# angular
<ul>
<li *ngFor="let fruit of fruits">{{fruit.name}}</li>
</ul>
Output:
We can declare an array of objects utilizing the interface type. The overhead approach has some flaws if the object has multiple properties and is hurdles to handle, and this approach makes an interface to hold object data in Angular and typescript.
It is useful to handle data reaching from the backend/database via REST API(RESTful APIs
). We can make an interface by using the command shown below.
# Angular
ng g interface Fruit
It develops a fruit.ts
file.
# Angular
export interface fruits {
id: number;
name: string;
constructor(id,name) {
this.id = id;
this.name = name;
}
}
In the Angular TypeScript component, We can make an interface for fruits.
# Angular
import { Component, OnInit } from "@angular/core";
import {Fruit} from './Fruit'
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"]
})
export class appComponent implements OnInit {
public fruits: Fruit[] = [
{ id: 1, name: "Apple" },
{ id: 2, name: "Banana" },
{ id: 3, name: "Guava" },
{ id: 4, name: "Strawberry" }
];
constructor() {}
ngOnInit() {
console.log(this.fruits)
}
}
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn