How to Filter Array in Angular
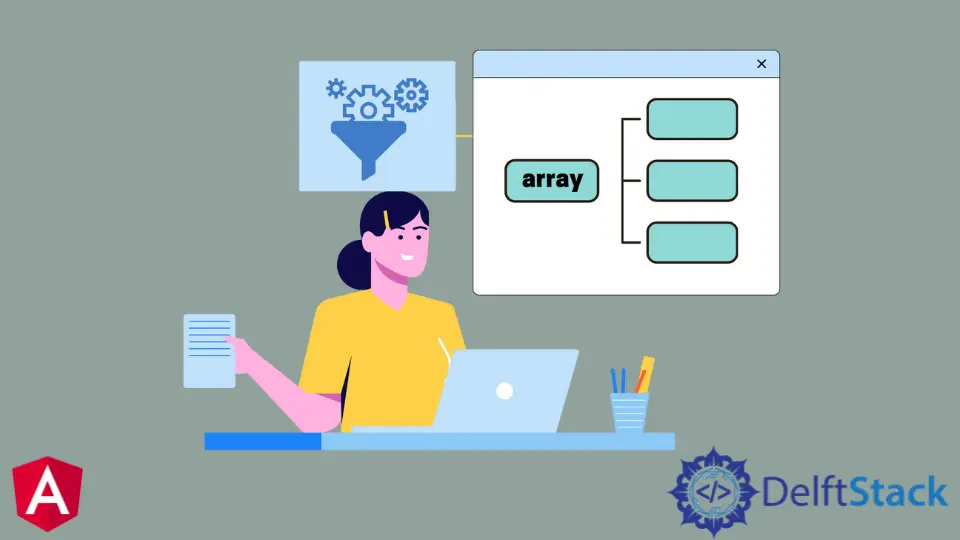
The filter()
method lets you apply a function to each element of an array and then return a new array containing only those elements for which the function returned true.
You can use the filter()
method to filter out elements in an array by their property values. This article will discuss the complete detail of filtering in Angular 2.
Angular 2 is an MVW Framework built on TypeScript and has a model-view-whatever architecture. It’s a complete rewrite of Angular 1 and takes advantage of the latest web technologies.
In Angular 2, arrays are represented by the type Array
. For example, an array of strings could be declared as follows.
var myArray = new Array();
myArray.push("John");
myArray.push("Mary");
myArray.push("Anna");
// myArray contains "John", "Mary" and "Anna"
Angular 2 Filter Array vs. Angular 1 Filter Array
Angular 2 filter is a template filter that takes an input and returns the transformed input. Angular 2 introduced the concept of pipes
which provides a way of changing data.
Pipes are created by using a pipe
. The pipe()
method creates a new pipe
and registers it with the current scope
under its name.
Whereas Angular 1 has built-in filters. These filters can be applied to an expression to perform a specific operation on the expression’s result.
The filter()
method is used to create a filter, and the apply()
method is used to apply the filter to an expression.
The difference between the Angular 2 filter and Angular 1 filter is you can use a pipe to chain multiple filters together in Angular 2. In contrast, you need to use parentheses to chain numerous filters in Angular 1.
To learn more about the Angular filter array, click here.
Now let’s create a filter in Angular 2 step by step. In Angular 2, the first thing we do is construct a new Pipe
. Below is how it will seem.
import { Pipe } from '@angular/core';
@Pipe({
name: 'AgePipe'
})
export class AgePipe {}
Use ngOnInit()
to Filter Array in Angular 2
The main thing used in the Angular filter is ngOnInit()
. Angular 2 has life cycle hooks that we can use to control how and when data bindings are updated.
The ngOnInit()
is invoked immediately after the first ngOnChanges()
, and before the ngDoCheck()
.
The ngOnInit
is one of the lifecycle hooks. Angular calls ngOnInit()
signal when the component is fully initialized.
We must import OnInit
from @angular/core
to use ngOnInit()
(actually, it is not required, but as a good practice, import the OnInit
). To learn more about ngOnInit
, click here.
When you use angular-CLI
to create a new component, ngOnInit
is automatically inserted. We will implement the ngOnInt()
in our example.
ngOnInit() {
this.setData();
}
setData() {
this.dataObject = HelloData;
}
The complete Typescript code is given below.
import { Component, OnInit } from '@angular/core';
import { Pipe } from '@angular/core';
@Pipe({
name: 'AgePipe'
})
export class AgePipe { }
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
dataObject = [];
filter = { RegCategoryName: '' }
constructor() { }
ngOnInit() {
this.setData();
}
setData() {
this.dataObject = HelloData;
}
filterIt($event) {
this.filter.RegCategoryName = $event.target.value;
this.dataObject = HelloData.filter(value => {
const data = { ...value };
data.List = data.List.map(ch => {
const list = { ...ch };
list.RegistrationCategory = list.RegistrationCategory.filter(gChild => {
return gChild.RegCategoryName.toLowerCase().indexOf(this.filter.RegCategoryName.toLowerCase()) !== -1
});
return list;
});
return data.List.some(list => !!list.RegistrationCategory.length);
});
}
}
const HelloData = [
{
"BookId": 1,
"Book": {
"BookId": 1,
"BookName": "When Life Begins",
},
"List": [
{
"RegistrationCategory": [
{
"RegistrationCategoryId": 203,
"RegCategoryName": "Abu Yahya"
}
],
"viewMore": false
}
]
},
{
"BookId": 2,
"Book": {
"BookId": 2,
"BookName": "British History",
},
"List": [
{
"RegistrationCategory": [
{
"RegistrationCategoryId": 204,
"RegCategoryName": "Usman"
}
],
"viewMore": false
}
]
},
{
"BookId": 3,
"Book": {
"BookId": 3,
"BookName": "Holidays",
},
"List": [
{
"BookId": 3,
"RegistrationCategory": [
{
"RegistrationCategoryId": 205,
"RegCategoryName": "Egon Homtic"
}
],
"viewMore": false
}
]
},
{
"BookId": 4,
"Book": {
"BookId": 4,
"BookName": "Destination",
"ShortCode": "ABC04",
},
"List": [
{
"RegistrationCategory": [
{
"RegistrationCategoryId": 205,
"RegCategoryName": "Steve Jobs"
}
],
"viewMore": false
},
]
}
]
After that, we will start working on HTML code, and our main concern is to work on ng-For
properly.
The ng-For
directive is used to iterate over a list of values and display each item in the list. It can be used as an alternative to ng-repeat
.
The ng-repeat
directive is often more appropriate when the data set contains many items, and we want to display them all on the page. But here, we are using a search filter to show the results according to the search command; that’s why ng-For
will be more useful than ng-repeat
.
<tr *ngFor="let data of dataObject">
<td>{{data.Book.BookName}}</td>
<td>
<div *ngFor="let cat of data.List">
<div *ngFor="let child of cat.RegistrationCategory">
The complete HTML code is given below.
Search by Id : <input type="text" (keyup)="filterIt($event)">
<table>
<tr>
<th>Books</th>
<th>Book Author (ID) Write Author name in the above search bar and see the magic of Angular 2 Filter</th>
</tr>
<tr *ngFor="let data of dataObject">
<td>{{data.Book.BookName}}</td>
<td>
<div *ngFor="let cat of data.List">
<div *ngFor="let child of cat.RegistrationCategory">
{{child.RegCategoryName}}
</div>
</div>
</td>
</tr>
</table>
Click here to check the live demonstration of the above code.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook