How to Use Blur Event in Angular
- Understanding the Blur Event
- Implementing the Blur Event in Angular
- Using Template-Driven Forms with Blur Event
- Conclusion
- FAQ
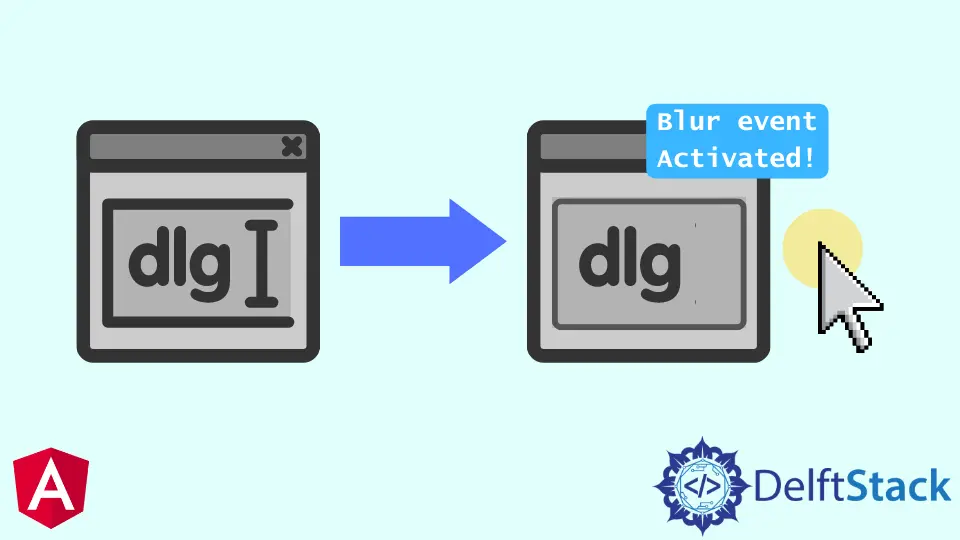
Angular is a powerful framework for building dynamic web applications, and understanding how to handle events is crucial for creating a smooth user experience. One of the lesser-discussed events is the blur event, which occurs when an element loses focus.
In this article, we will demonstrate how to use the blur event in Angular, providing you with practical examples and explanations to enhance your application’s interactivity. Whether you’re a beginner or an experienced developer, mastering the blur event can help you improve form validation, user feedback, and overall application functionality. Let’s dive in and explore how to effectively implement the blur event in your Angular applications.
Understanding the Blur Event
The blur event in Angular is triggered when an input element loses focus. This event is particularly useful for form validation, as it allows you to perform checks when the user navigates away from an input field. For instance, you might want to validate an email address or check if a required field is filled out. By using the blur event, you can provide instant feedback to the user, enhancing the overall user experience.
To implement the blur event in Angular, you can use the built-in event binding syntax. This involves attaching an event handler to the blur event of an input element. Let’s look at how to set this up in your Angular component.
Implementing the Blur Event in Angular
To begin, you will need to create an Angular component where you can implement the blur event. Below is a simple example demonstrating how to use the blur event to validate an email input field.
import { Component } from '@angular/core';
@Component({
selector: 'app-email-validation',
template: `
<input type="email" (blur)="validateEmail($event)" placeholder="Enter your email" />
<p *ngIf="errorMessage">{{ errorMessage }}</p>
`
})
export class EmailValidationComponent {
errorMessage: string = '';
validateEmail(event: FocusEvent) {
const input = event.target as HTMLInputElement;
const emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!emailPattern.test(input.value)) {
this.errorMessage = 'Please enter a valid email address.';
} else {
this.errorMessage = '';
}
}
}
In this example, we have created a simple email validation component. The validateEmail
method is triggered when the input field loses focus. Inside this method, we check if the entered email matches a basic regex pattern. If it doesn’t, an error message is displayed below the input field, guiding the user to correct their input.
Output:
Please enter a valid email address.
This example highlights how the blur event can be used effectively for real-time validation. By providing immediate feedback, you enhance the user experience and reduce the likelihood of form submission errors.
Using Template-Driven Forms with Blur Event
Angular also supports template-driven forms, which can simplify form handling. Using the blur event in this context can make validation even more straightforward. Here’s how you can implement the blur event in a template-driven form.
import { Component } from '@angular/core';
@Component({
selector: 'app-template-driven-form',
template: `
<form #form="ngForm">
<input name="username" ngModel (blur)="checkUsername(form)" placeholder="Enter your username" />
<p *ngIf="errorMessage">{{ errorMessage }}</p>
</form>
`
})
export class TemplateDrivenFormComponent {
errorMessage: string = '';
checkUsername(form: any) {
const username = form.value.username;
if (username.length < 3) {
this.errorMessage = 'Username must be at least 3 characters long.';
} else {
this.errorMessage = '';
}
}
}
In this example, we have a template-driven form with a username input field. When the input loses focus, the checkUsername
method is invoked. This method checks if the username is at least three characters long and displays an error message if it is not.
Output:
Username must be at least 3 characters long.
Using template-driven forms with the blur event allows for a clean and efficient way to manage user input, improving the overall usability of your application.
Conclusion
In conclusion, the blur event in Angular is a valuable tool for enhancing user experience through real-time validation and feedback. By implementing the blur event in your components and forms, you can ensure that users receive immediate guidance on their input, thereby reducing errors and improving the overall functionality of your application. Whether you are using reactive forms or template-driven forms, mastering the blur event can significantly elevate your Angular development skills. Start incorporating the blur event into your next project and see the difference it makes!
FAQ
-
What is the blur event in Angular?
The blur event is triggered when an input element loses focus, allowing developers to perform actions like validation. -
How can I use the blur event for form validation?
You can bind the blur event to an input field and invoke a validation method to check the input value when the field loses focus. -
Can I use the blur event with template-driven forms?
Yes, the blur event can be easily integrated into template-driven forms for real-time validation. -
What are some common use cases for the blur event?
Common use cases include validating email addresses, checking username length, and providing instant feedback on required fields. -
Is it possible to use the blur event with reactive forms?
Absolutely! The blur event can be utilized in reactive forms to enhance validation and user feedback.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn