How to Access Parent Scope From the Child Controller in Angular
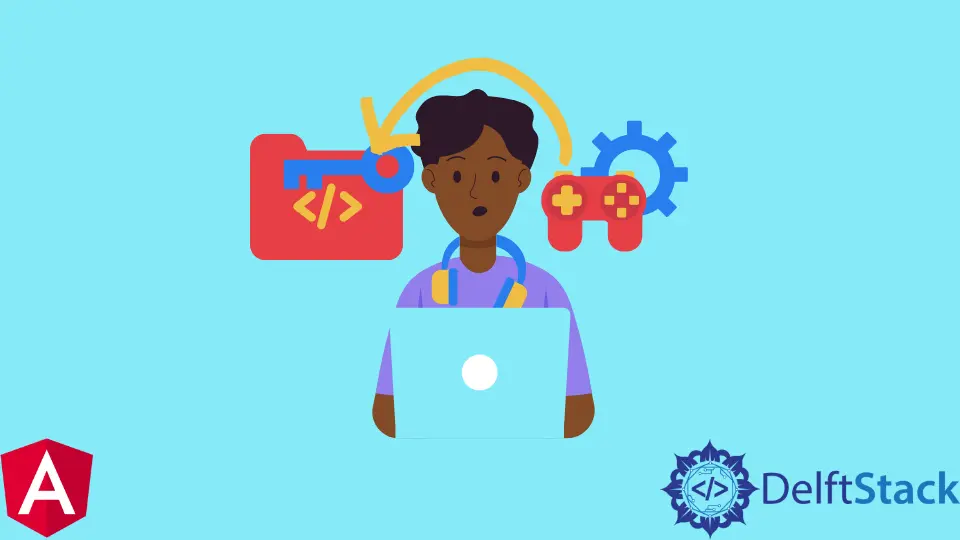
We will introduce how to access parent scope from the child controller in Angular.
Access Parent Scope From the Child Controller in Angular
When we work on a component-based library or framework, there are a lot of scenarios in which we have to share data between two components.
First, we will create a users
component.
# angular CLI
ng g c users
The above command will generate a new users
component.
We can see that there will be a new users folder inside the app folder. To display our users
component, we need to use the users selector
set in user.component.ts
.
So let’s display the users
component inside the app
component. First, we need to add the UsersComponent
in declarations of the app.module.ts
.
So, the app.module.ts
will look like below.
# angular
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
import { UsersComponent } from './users/users.component';
@NgModule({
imports: [ BrowserModule, FormsModule ],
declarations: [ AppComponent, HelloComponent, UsersComponent],
bootstrap: [ AppComponent ]
})
export class AppModule { }
We need to create a tag with users selector in the app.component.html
, and it will display anything we have added in the users.component.html
. Our code in the app.component.html
will look like below.
# angular
<hello name="{{ name }}"></hello>
<p>Start editing to see some magic happen :)</p>
<app-users></app-users>
Output:
As seen in the picture, the app-users
tag has displayed users works!
because, in users.component.html
, we have the following code.
# angular
<p>users works!</p>
Let’s convert it into a heading and send data from the parent component.
Firstly we will change our <p>
tag to <h3>
.
# angular
<h3>Child Component</h3>
Output:
We will send simple data from parent component to child component. We will define a variable inside the app.component.ts
file, so our code will be below.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
name = 'Angular ' + VERSION.major;
data = "User Name"
}
As seen in the code, we assigned User Name
to data
; let us send this data
to the child component. So, the app.component.html
will look like below.
# angular
<hello name="{{ name }}"></hello>
<p>Start editing to see some magic happen :)</p>
<app-users [data] = "data"></app-users>
By binding [data]
with the variable data
, we send the child component the data, but it is not completely bound yet.
We must import the input
in the users.component.ts
file. Input
is a decorator which will read the data we have sent to our child component and display it in the child component.
Inside our UsersComponent
class, we will read the property we bound in the app.component.html
inside the app-users
tag. So, our code in the users.component.ts
will look like below.
# angular
import { Component, OnInit, Input } from '@angular/core';
@Component({
selector: 'app-users',
templateUrl: './users.component.html',
styleUrls: ['./users.component.css']
})
export class UsersComponent implements OnInit {
@Input() data
constructor() { }
ngOnInit() {
}
}
As seen in the code, we used @Input() data
to bind the [data]
from the app-users
tag in the app.component.html
. We will display the data we have received inside the users.component.html
file.
# angular
<h3>Child Component</h3>
<h4>Data Recieved:</h4>
<p>{{data}}</p>
Output:
We have received data from parent component to child component. We will now discuss how to share an object from parent to child.
Let’s try to share an object by changing the data we send.
# angular
data = {
name: 'Rana Hasnain',
age: 25
}
Output:
We can see that we display the [object Object]
instead of data from that object. What we must do now is modify our code in the users.component.html
file and change {{data}}
to {{data.name}}
or {{data.age}}
, and it will display the object.
# angular
<h3>Child Component</h3>
<h4>Data Received:</h4>
<p>Name: {{ data.name }}</p>
<p>Age: {{ data.age }}</p>
Output:
So now we can see that we have shared data and objects from parent to child components.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Angular Controller
- Controller as Syntax in AngularJS
- How to Create Multiple Controllers in One Page in AngularJS
- How to Share Data Between Controllers in AngularJS