How to Share Data Between Controllers in AngularJS
- Share Data Between Parent and Child Controllers in AngularJS
- Share Data Between Controllers Using Factory or Service in AngularJS
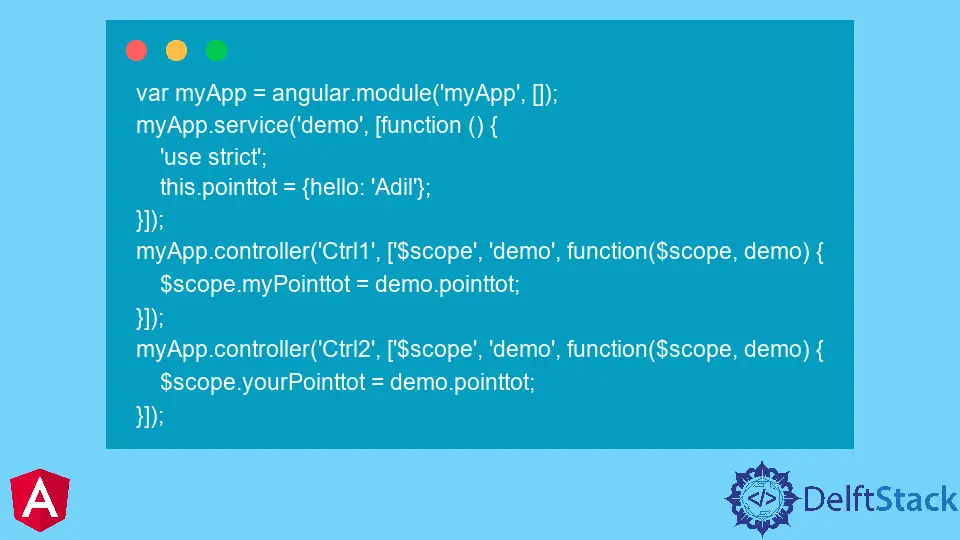
We can use several methods to share data between controllers in AngularJS, but here we will only discuss two ways. The first way is to scope variables. The second way is to use a factory
or service
.
Share Data Between Parent and Child Controllers in AngularJS
The scope of a child controller inherits the parent controller’s scope, allowing data to be shared simply by using controller inheritance.
When a child component is nested within a parent component, the child component can access data from the parent.
This is accomplished through the use of two-way data binding. The parent component can pass data to the child using either a property binding or an event binding.
The child can then pass this data back to the parent using a property binding or an event binding on its controller. This pattern is also used for sharing selections between related components.
The Scope Object
The scope object is created when a controller is instantiated, and it contains both model and view properties.
The model property holds or stores any information shared with other controllers. On the other hand, the view property has or stores information about displaying this information on the screen (such as HTML).
Share Data Between Controllers Using Factory or Service in AngularJS
In AngularJS, we can share data between controllers using factories or services.
Factories return an object with the methods to create and update objects. We can use factories when we want to create a new object in one controller and share it with another controller.
Services are used when we want to share data between controllers and other JavaScript files.
In conclusion, AngularJS factories and services are JS modules that execute a specific purpose and contain both methods and properties.
They may be injected into other components via dependency injection. We can define a shared variable in a factory, inject it into both controllers, and link scope variables to factory data in this method.
We must follow the steps below.
-
Register the factory or service.
-
Create a service or factory that will be responsible for fetching the data.
-
Add the service or factory to the module’s list of providers.
-
Create a controller with a function that will use the service.
Below is an example to help us understand how to share data between controllers using the two ways discussed above.
JavaScript code:
var myApp = angular.module('myApp', []);
myApp.service('demo', [function () {
'use strict';
this.pointtot = {hello: 'Adil'};
}]);
myApp.controller('Ctrl1', ['$scope', 'demo', function($scope, demo) {
$scope.myPointtot = demo.pointtot;
}]);
myApp.controller('Ctrl2', ['$scope', 'demo', function($scope, demo) {
$scope.yourPointtot = demo.pointtot;
}]);
HTML code:
<!DOCTYPE html>
<html ng-app="myApp">
<head>
<script src="http://code.angularjs.org/1.2.1/angular.js"></script>
<script src="script.js"></script>
</head>
<body>
<h1>Example of sharing data between controllers</h1>
<div ng-controller="Ctrl1">
<p>Write something in Controller 1 <input ng-model="myPointtot.hello" type="text" /></p>
</div>
<div ng-controller="Ctrl2">
<h4>Everything you write in controller 1, it will be copied to controller 2 and vice versa</h4>
<p>Write something in Controller 2 <input ng-model="yourPointtot.hello" type="text" /></p>
</div>
</body>
</html>
Click here to check the live demonstration of the code above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook