Python sys.getswitchinterval() Method
-
Introduction to
sys.getswitchinterval()
in Python -
Syntax of the Python
sys.getswitchinterval()
Method -
Example 1: Use the
sys.getswitchinterval()
Method in Python -
Example 2: Change the Values of Time Intervals Using the
sys.getswitchinterval()
Method in Python -
Example 3: Use the Max Value of a Floating Point Number in the
sys.getswitchinterval()
Method - Practical Applications
-
Fine-Tuning With
sys.setswitchinterval()
- Considerations and Best Practices
- Conclusion
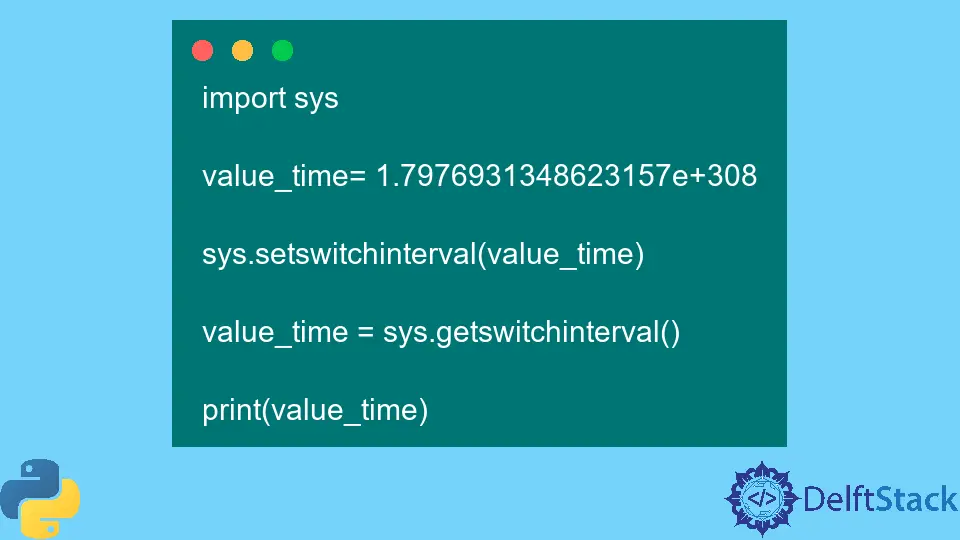
Python, renowned for its simplicity and versatility, offers a multitude of built-in functions and modules to empower developers. One such function, sys.getswitchinterval()
, plays a crucial role in managing the context-switching behavior of threads.
Python’s sys.getswitchinterval()
method is an efficient way of determining the ideal duration of the time slices allocated to Python threads executing simultaneously. The time is represented in floating point notation in seconds.
In this article, we will delve into the depths of sys.getswitchinterval()
, exploring its purpose, functionality, and practical applications.
Introduction to sys.getswitchinterval()
in Python
The sys
module in Python provides access to system-specific parameters and functions. One such function is the sys.getswitchinterval()
method that allows you to retrieve the current thread’s suggested switch interval.
Understanding Context Switching
Before delving into sys.getswitchinterval()
, it’s crucial to comprehend the concept of context switching. In a multi-threaded environment, the CPU allocates time slices to different threads.
Context switching refers to the process of saving the current state of a thread, switching to another thread, and later restoring the original thread’s state.
Efficient context switching is vital for maximizing CPU utilization and ensuring responsive and concurrent execution of threads.
How sys.getswitchinterval()
Works
sys.getswitchinterval()
returns the current thread’s suggested time-slice duration, measured in seconds. This duration represents the time before the Python interpreter considers switching to a different thread.
import sys
switch_interval = sys.getswitchinterval()
print(f"Current switch interval: {switch_interval} seconds")
This code uses the sys.getswitchinterval()
function to retrieve the current thread’s suggested switch interval and then prints it to the console.
The output will be the current switch interval, which may vary based on the system and Python interpreter settings.
Current switch interval: 0.005 seconds
Syntax of the Python sys.getswitchinterval()
Method
sys.getswitchinterval()
Parameters
No parameter is required. It is a non-callable object.
Return
This method returns the interpreter’s thread switch interval.
Example 1: Use the sys.getswitchinterval()
Method in Python
This method can only be used after importing the sys
library.
import sys
value_time = sys.getswitchinterval()
print("The switch time interval of the interpreter’s thread is: ", value_time)
We’re going to break down what each line does.
First, the import sys
imports the sys
module, which provides access to system-specific parameters and functions.
The value_time = sys.getswitchinterval()
line calls the sys.getswitchinterval()
function to retrieve the current thread’s suggested switch interval and assigns it to the variable value_time
.
Then, the print("The switch time interval of the interpreter’s thread is: ", value_time)
prints a message along with the value of value_time
. It displays the switch time interval of the interpreter’s thread.
When you run this script, it will output something similar to:
The switch time interval of the interpreter’s thread is: 0.005
The actual value may vary depending on the Python interpreter and system configuration.
Example 2: Change the Values of Time Intervals Using the sys.getswitchinterval()
Method in Python
Note that even if you enter an integer value as an input time interval, it is converted to the float data type.
import sys
value_time = sys.getswitchinterval()
print(
"Before changing, the switch interval of the interpreter's thread is= ", value_time
)
value_time = 1
sys.setswitchinterval(value_time)
value_time = sys.getswitchinterval()
print(
"After changing, the switch interval of the interpreter's thread is= ", value_time
)
This code utilizes the sys.getswitchinterval()
and sys.setswitchinterval()
functions to manage the switch interval of the interpreter’s thread.
It begins by importing the sys
module to access system-specific parameters and functions. The value_time
is assigned the current switch interval using sys.getswitchinterval()
.
Next, the original switch interval is printed to the console. The switch interval is then modified to 1
second.
Then, sys.setswitchinterval(value_time)
is used to implement this change.
The updated switch interval is retrieved and assigned back to value_time
. The final switch interval is printed to the console.
The output demonstrates the switch interval before and after modification:
Before changing, the switch interval of the interpreter's thread is= 0.005
After changing, the switch interval of the interpreter's thread is= 1.0
These values may vary depending on the specific Python interpreter and system settings.
Example 3: Use the Max Value of a Floating Point Number in the sys.getswitchinterval()
Method
In this example, we are setting the switch time interval to the max value of the floating point data type. This is done to make sure that no other thread is executed simultaneously.
import sys
value_time = 1.7976931348623157e308
sys.setswitchinterval(value_time)
value_time = sys.getswitchinterval()
print(value_time)
Output:
0.0
In this code, you’re attempting to set the switch interval to a very large value (1.7976931348623157e308
). This value is the maximum representable finite floating-point number in Python, which is approximately 1.7976931348623157 × 10^308
.
However, it’s important to note that setting the switch interval to such an extremely large value may lead to unexpected behavior. The switch interval is typically specified in seconds, and using such a large value may not be practical or meaningful in the context of thread switching.
The actual behavior may vary depending on the Python interpreter and system-specific limitations. It’s recommended to use reasonable values for the switch interval to ensure predictable and stable thread behavior.
Practical Applications
Performance Optimization
By inspecting the switch interval, you can fine-tune your application’s threading behavior to achieve optimal performance. This is particularly valuable in scenarios where responsiveness and resource utilization are critical.
Real-Time Systems
In real-time systems, meeting strict timing constraints is essential. Adjusting the switch interval can help in achieving precise timing for critical tasks.
Resource Allocation
Understanding the switch interval can aid in allocating resources effectively among concurrent tasks. It allows you to strike a balance between responsiveness and resource utilization.
Fine-Tuning With sys.setswitchinterval()
While sys.getswitchinterval()
retrieves the current switch interval, sys.setswitchinterval(value)
allows you to set a new switch interval.
import sys
new_interval = 0.005 # Example value in seconds
sys.setswitchinterval(new_interval)
This can be particularly useful when you need to adjust the threading behavior to align with specific performance requirements.
Considerations and Best Practices
- Use with Caution: Modifying switch intervals can have a significant impact on thread behavior. It’s crucial to thoroughly test and profile your application to ensure the changes yield the desired results.
- Platform Dependence: The behavior of
sys.getswitchinterval()
may vary based on the underlying operating system and Python interpreter implementation. - Avoid Unnecessary Tweaks: In most cases, the default switch interval provided by the Python interpreter is sufficient for typical applications. Fine-tuning should be considered when specific performance requirements or constraints necessitate it.
Conclusion
The sys.getswitchinterval()
method is a powerful tool for understanding and fine-tuning the context-switching behavior of threads in Python. By leveraging this function, you can optimize performance, meet timing constraints in real-time systems, and allocate resources effectively among concurrent tasks.
However, it’s important to approach thread tuning with caution, as excessive tweaking can lead to unintended consequences. Thorough testing and profiling are essential when adjusting switch intervals.
In summary, sys.getswitchinterval()
provides valuable insight into thread behavior, allowing developers to achieve optimal performance in multi-threaded applications.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn