Python sys.exc_info() Method
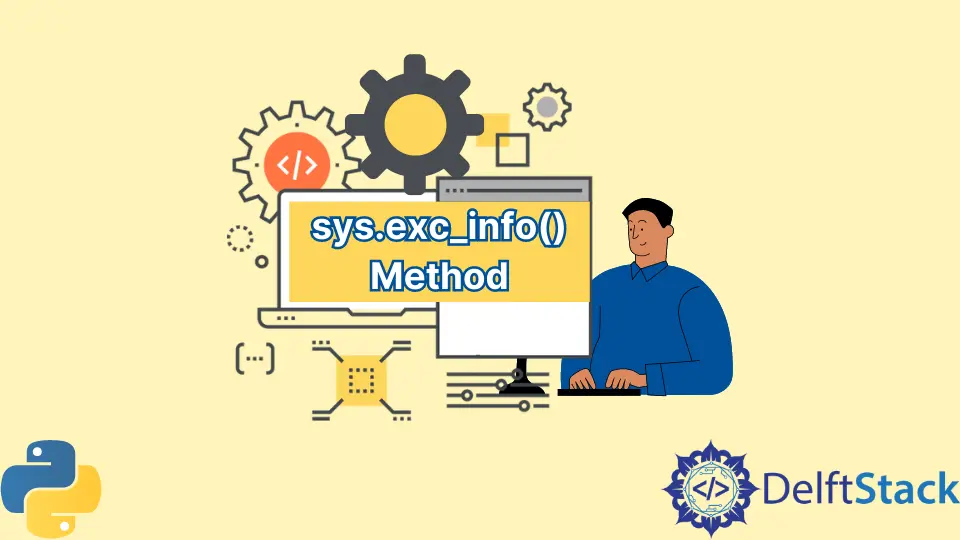
Python sys.exc_info()
method is an efficient way of getting information about the current exception that has occurred and is handled by the system. The data returned by this method is specific to both the current system thread and the current stack frame.
Syntax
sys.exc_info()
Parameters
no parameters |
It is a non-callable object. |
Returns
The method’s return type is a tuple
consisting of three values. The values are as follows: (type
, value
, traceback
)
type
: denotes thetype
of exception being handled.value
: it gets the instance of an exceptiontype
.traceback
: it returns atraceback
object that encapsulates the system call stack at the point where the exception originated.
Note that if the stack system handles no exception, a tuple
containing three None
values is returned.
Example Codes
Work With the sys.exc_info()
Method
import sys
import math
try:
x = 1 / 0
except:
tuples = sys.exc_info()
print(tuples)
Output:
(<class 'ZeroDivisionError'>, ZeroDivisionError('division by zero'), <traceback object at 0x7f6607d72240>)
Any number divided by 0
is not defined, so an exception is thrown. The method sys.exc_info()
gives detailed information about the exception.
Break Down the Tuple Returned by the sys.exc_info()
Method
import sys
import math
try:
x = 1 / 0
except:
tuples = sys.exc_info()
print("Type: ", tuples[0])
print("Value: ", tuples[1])
print("Traceback: ", tuples[2])
Output:
Type: <class 'ZeroDivisionError'>
Value: division by zero
Traceback: <traceback object at 0x7efc2f10b240>
The method sys.exc_info()
gives us information about the exception in the form of a tuple
. The tuple
can also be accessed element-wise, as shown above.
Use Mathematical Equation With the sys.exc_info()
Method
import sys
import math
a = 1
b = -8
c = 5
try:
root1 = (-(b) + (math.sqrt(b * b - 4 * a * c))) / (2 * a)
root2 = (-(b) - (math.sqrt(b * b - 4 * a * c))) / (2 * a)
print("Two real and distinct roots: ", root1, root2)
except:
e, p, t = sys.exc_info()
print("Two imaginary and distinct roots! Error generated: ", e, p)
a = 2
b = 2
c = 1
try:
root1 = (-(b) + (math.sqrt(b * b - 4 * a * c))) / (2 * a)
root2 = (-(b) - (math.sqrt(b * b - 4 * a * c))) / (2 * a)
print("Two real and distinct roots: ", root1, root2)
except:
e, p, t = sys.exc_info()
print("Two imaginary and distinct roots! Error generated: ", e, p)
Output:
Two real and distinct roots: 7.3166247903554 0.6833752096446002
Two imaginary and distinct roots! Error generated: <class 'ValueError'> math domain error
In the above code, we implemented the quadratic formula. The answers are shown in floating point notations when we have two distinct real roots.
In the second attempt, the output was two different imaginary numbers that the system couldn’t process, so an exception was thrown.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn