Python math.log() Method
- Syntax
- Example 1: Natural Logarithm of a Number (One Argument)
- Example 2: Natural Logarithm of a Number (Two Arguments)
- Example 3: If Input Is Not a Number
-
Example 4: If Input Is Less Than or Equal to
0
- Example 5: If Input Is Infinity
-
Example 6: If Input Is
NaN
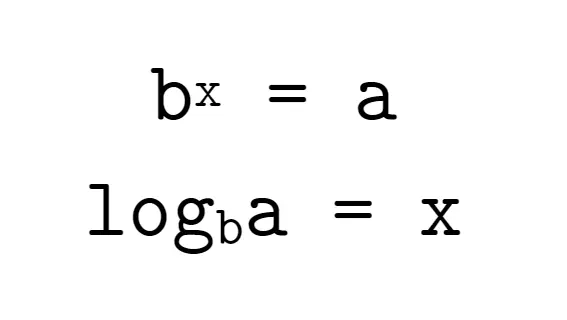
The Python programming language offers a library, math
, that contains implementation for various mathematical operations such as trigonometric functions and logarithmic functions.
Logarithm refers to the inverse function of exponentiation. Simply put, the logarithm of a number a
is the exponent or number (x
) to which another number, the base b
, should be raised to make that number a
.
In this article, we will discuss a method, log()
, available in the math
module that computes the logarithm of a number.
Syntax
math.log(x, base)
Parameters
Type | Description | |
---|---|---|
x |
Float | A non-negative integer and non-zero value. |
base |
Float | A base value for the log in the ranges (0, 1) and (1, ∞) . |
Return
The log()
method returns different results for different sets of parameters. If it gets a single argument, say x
, it returns the natural logarithm of x
or logarithm to the base of the mathematical constant e
.
If it gets two arguments, say x
and base
, it returns the logarithm of x
to the base of base
or log(x) / log(base)
.
logₘn = logₓn / logₓm
where m and n are real numbers greater than zero and x is in the ranges (0, 1) and (1, ∞).
Example 1: Natural Logarithm of a Number (One Argument)
import math
print(math.log(0.000001))
print(math.log(1))
print(math.log(0.341))
print(math.log(99999))
print(math.log(2352.579))
Output:
-13.815510557964274
0.0
-1.07587280169862
11.512915464920228
7.7632674521921885
The Python code above computes the natural logarithm value for 0.000001
, 1
, 0.341
, 99999
, and 2352.579
. Note that these values are non-negative and non-zero.
The natural logarithm returns negative results for inputs in the range (0, 1)
. On the flip side, the range [1, ∞)
produces positive results.
Example 2: Natural Logarithm of a Number (Two Arguments)
import math
print(math.log(0.000001, math.e))
print(math.log(1, 0.1234))
print(math.log(0.341, 5324))
print(math.log(99999, 0.00001))
print(math.log(2352.579, 1.000001))
Output:
-13.815510557964274
-0.0
-0.1253933900998167
-0.9999991314066932
7763271.334463926
Example 3: If Input Is Not a Number
import math
print(math.log("this is a string"))
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(math.log("this is a string"))
TypeError: must be real number, not str
The Python code above takes a string as an input. Since, the log()
method expects a numerical value, it raises a TypeError
.
Example 4: If Input Is Less Than or Equal to 0
import math
print(math.log(0))
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(math.log(0))
ValueError: math domain error
The Python code above takes 0
as input, and since 0
is out of the log()
method’s domain, it raises a ValueError
.
import math
print(math.log(-54.2))
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
print(math.log(-54.2))
ValueError: math domain error
The Python code above takes a value less than 0
as input, and such values are invalid inputs for the log()
method. Hence, it raises a ValueError
.
Example 5: If Input Is Infinity
import math
print(math.log(math.inf))
Output:
inf
Example 6: If Input Is NaN
import math
print(math.log(math.nan))
Output:
nan