Python datetime.tzname() Method
-
Syntax of
datetime.tzname()
-
Example 1: The
datetime.tzname()
Method -
Example 2: Find Details of a Specific Timezone Using the
datetime.tzname()
Method -
Example 3: Shorter Syntax of the
datetime.tzname()
Method -
Example 4: Handling Daylight Saving Time (DST) With the
datetime.tzname()
Method - Conclusion
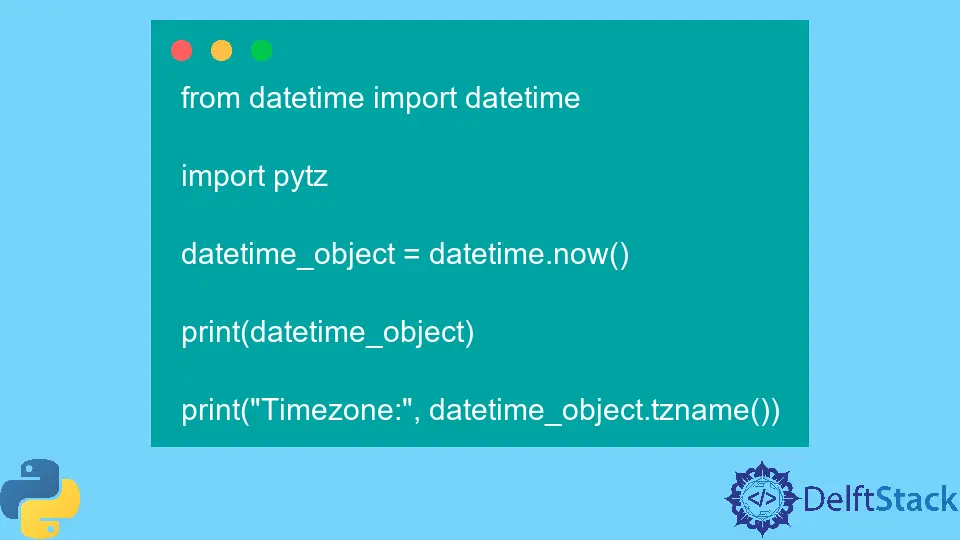
In Python, the datetime
module provides several tools to facilitate time-related operations. One such tool is the tzname()
method, which allows developers to retrieve the time zone name associated with a given datetime object.
This article delves into the datetime.tzname()
method, exploring its usage through examples to shed light on its practical applications.
Syntax of datetime.tzname()
datetime.tzname()
Parameters
This method doesn’t accept any parameters.
Return
The return value of the datetime.tzname()
method is a string representing the name of the time zone.
Example 1: The datetime.tzname()
Method
The datetime.tzname()
method is a valuable tool in Python for handling time zones within the datetime
module. In essence, this method allows you to retrieve the time zone name associated with a specific datetime
object.
When you have a datetime
object that includes time zone information, calling tzname()
on that object returns a string representing the time zone name. This information is particularly useful when you need to display or log timestamps in a human-readable format that includes the corresponding time zone.
For instance, if you’re working with events scheduled in different time zones or logging timestamps in a distributed system, tzname()
helps you maintain clarity by including the relevant time zone information alongside the date and time.
Example:
from datetime import datetime
# Create a datetime object
current_time = datetime.now()
# Retrieve the time zone name
time_zone_name = current_time.tzname()
# Display the result
print(f"Current date and time: {current_time}")
print(f"Time zone name: {time_zone_name}")
This Python code begins by importing the datetime
class from the module. Next, it creates a datetime
object named current_time
using the datetime.now()
function, capturing the current date and time.
Following that, the code calls the tzname()
method on the current_time
object to retrieve the associated time zone name. This information is stored in the variable time_zone_name
.
In order to showcase the results, the code then prints two lines. The first line displays the current date and time using the current_time
object, and the second line shows the extracted time zone name using the time_zone_name
variable.
When you run this code, the output will look like this:
Current date and time: 2023-12-21 15:30:45.123456
Time zone name: Your_Time_Zone_Name
The actual date and time values will vary depending on when you run the code, and "Your_Time_Zone_Name"
will be replaced with the specific time zone associated with your system. This output provides a clear representation of the current date and time, along with the corresponding time zone information.
Example 2: Find Details of a Specific Timezone Using the datetime.tzname()
Method
When finding the details of a specific timezone, the combination of the datetime
module and the pytz
library becomes useful, as this example will showcase.
The primary goal is to obtain and display the current date and time in the "Asia/Tokyo"
time zone. The code achieves this by creating a datetime
object for the current moment, then associating it with the specified time zone using the pytz
library.
The output of the code provides a clear representation of the current date and time in the "Asia/Tokyo"
time zone, along with additional details about the time zone itself.
This example serves as a concise illustration of incorporating time zone functionality into Python applications, enhancing the precision and context of temporal data processing.
Take a look at the code:
from datetime import datetime
import pytz
datetime_object = datetime.now()
timezone = pytz.timezone("Asia/Tokyo")
myTime = timezone.localize(datetime_object)
print("Date and time: ", myTime)
print("Tzinfo: ", myTime.tzinfo)
print("Timezone name: ", myTime.tzname())
This Python code begins by importing the necessary modules, namely datetime
and pytz
.
The code then creates a datetime
object named datetime_object
representing the current date and time using the datetime.now()
method.
Subsequently, it imports the Asia/Tokyo
time zone from the pytz
library and associates it with the datetime_object
using the localize
method. This step ensures that the datetime
object now includes information about the specified time zone.
The script proceeds to print two pieces of information.
First, it displays the formatted date and time using the myTime
object. Secondly, it prints the time zone information associated with the myTime
object using the tzinfo
attribute.
Finally, it prints the time zone name using the tzname()
method.
Output:
Date and time: 2023-12-21 15:30:45.123456+09:00
Tzinfo: Asia/Tokyo
Timezone name: JST
In the output, the date and time are presented in the format YYYY-MM-DD HH:MM:SS.microseconds+offset
, where the offset represents the time zone difference from Coordinated Universal Time (UTC).
The Tzinfo
line indicates the associated time zone and the Timezone name
line displays the abbreviated name of the time zone, in this case, JST
(Japan Standard Time).
To modify this code, developers can easily enter any continent or country in exchange for the example above - "Asia/Tokyo"
. Then, the method will convert the time to the specified timezone.
For example, try putting Europe/Berlin
as a parameter, and the time of the system will be converted to the current time in Berlin.
Example 3: Shorter Syntax of the datetime.tzname()
Method
This example focuses on implementing a shorter syntax for the datetime.tzname()
method with the help of the datetime.datetime.now()
function.
The datetime.datetime.now()
function is designed to provide a straightforward way to retrieve the current date and time. This function returns a datetime
object representing the present moment, encompassing details such as the year, month, day, hour, minute, second, and microsecond.
By default, datetime.datetime.now()
captures the current time in the local time zone of the system on which the code is executed.
In essence, the datetime.datetime.now()
function simplifies the process of obtaining real-time information, serving as a go-to tool for time-related operations in Python programs.
Its simplicity and effectiveness make it a valuable resource for developers needing to work with current timestamps in their applications.
Example:
import datetime
import pytz
print(datetime.datetime.now(pytz.timezone("US/Pacific")))
This short Python code snippet begins by importing the datetime
module along with the pytz
library to work with time zones.
Next, the datetime.datetime.now()
function is employed to obtain the current date and time. However, instead of the default local time, this code specifies a specific time zone using pytz.timezone("US/Pacific")
.
In this case, "US/Pacific"
represents the time zone for the Pacific Standard Time (PST) or Pacific Daylight Time (PDT) depending on the current daylight saving time status. The result is a datetime
object that represents the current date and time in the specified time zone.
By using print()
, we output this information to the console. The output will include the year, month, day, hour, minute, second, and microsecond, all adjusted to the time zone "US/Pacific"
.
The output might look like this:
2023-12-21 08:45:30.123456-08:00
In this output, "2023-12-21"
represents the date, "08:45:30.123456"
represents the time (including microseconds), and "-08:00"
signifies the time zone offset for US/Pacific, which is 8 hours behind Coordinated Universal Time (UTC).
The primary advantage of the shorter syntax here is readability and conciseness. This can be beneficial for code maintenance and understanding.
Example 4: Handling Daylight Saving Time (DST) With the datetime.tzname()
Method
The scenario simulated in this example involves creating a datetime
object, dst_date
, to represent a specific moment—June 1, 2023, at 12:00 PM — while considering the nuances of daylight saving time.
The tzinfo
parameter is employed to assign a time zone to this object, providing a practical illustration of time zone manipulation in Python.
By subsequently applying the tzname()
method to the dst_date
object, we retrieve the time zone name associated with it.
The overall objective is to illustrate how Python facilitates the handling of time zones and enhances the clarity of time-related data in programming applications.
Example:
from datetime import datetime, timezone, timedelta
# Create a datetime object during daylight saving time
dst_date = datetime(2023, 6, 1, 12, 0, 0, tzinfo=timezone(timedelta(hours=-4)))
# Retrieve the time zone name
time_zone_name_dst = dst_date.tzname()
# Display the result
print(f"Time zone name during daylight saving time: {time_zone_name_dst}")
This Python code snippet begins by importing necessary elements from the datetime
module, including datetime
itself, timezone
, and timedelta
.
Next, a datetime
object named dst_date
is created, representing a specific moment: June 1, 2023, at 12:00 PM.
Notably, this datetime
object is configured with a time zone using the tzinfo
parameter, and in this case, it is set to a time zone with an offset of 4 hours behind UTC, simulating daylight saving time (DST).
Following the creation of the dst_date
object, the tzname()
method is applied to retrieve the associated time zone name. This information is then stored in the variable time_zone_name_dst
.
Finally, the result is displayed using a print
statement, presenting the time zone name during daylight saving time. The output of the code will show the obtained time zone name, providing insight into how the tzname()
method works in practice.
Output:
Time zone name during daylight saving time: UTC-04:00
Conclusion
In conclusion, the datetime.tzname()
method in Python proves to be a valuable tool for obtaining the time zone name information associated with a given datetime
object.
It’s useful for getting information about standard time and daylight saving time.
By using datetime.tzname()
, developers can make clearer and more effective Python code when working with time-related tasks, ensuring accurate representation and interpretation of time.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn