Python datetime.toordinal() Method
-
Syntax of Python
datetime.toordinal()
Method -
Example 1: Use the
datetime.toordinal()
Method in Python -
Example 2: Enter Out-Of-Range Date as a Parameter to
datetime.toordinal()
Method -
Example 3: Find the Day Number Using the
datetime.toordinal()
Method -
Example 4: Maximum and Minimum Values of the
datetime.toordinal()
Method -
ValueError: year 0 is out of range
Encounter in Usingdatetime.toordinal()
Method - Conclusion
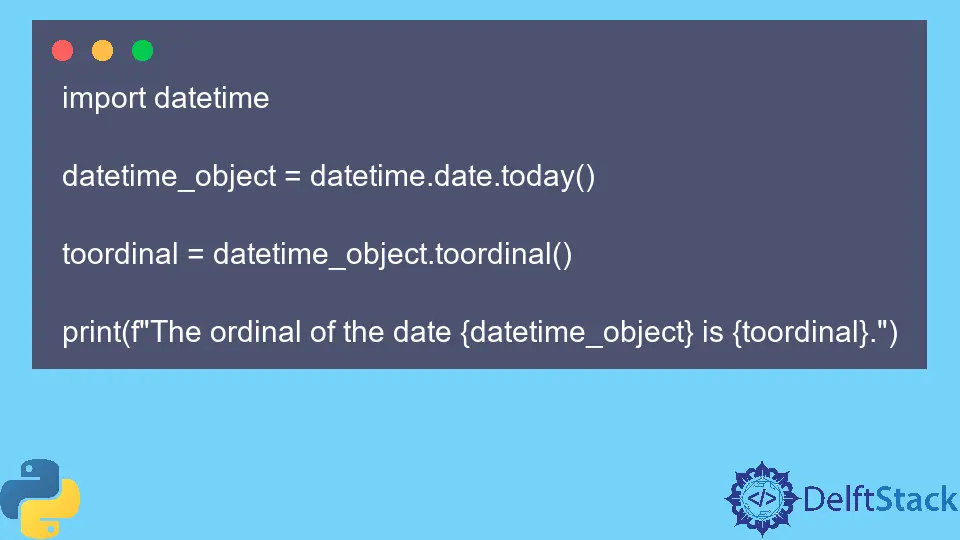
In programming, dealing with dates and times is a common task in many applications, from simple scripts to complex data analysis pipelines.
The datetime
module provides a range of functions and methods to work with dates and times efficiently.
One such method is toordinal()
, which converts a datetime
object into a proleptic Gregorian ordinal.
Python datetime.toordinal()
method is an efficient way of finding the proleptic Gregorian ordinal of the date.
The Gregorian calendar is the standard calendar used in most of the world today. It assigns a unique integer to each day, starting from January 1, 1 AD
, which is considered day 1.
Note that January 1 of year 1 has ordinal 1 and so on.
This integer representation of dates can be useful for various purposes, such as sorting, comparison, and calculations.
Syntax of Python datetime.toordinal()
Method
datetime.toordinal()
Parameters
This method doesn’t accept any parameter.
Return
This method returns an object representing the ordinal value for the specified datetime
object.
Example 1: Use the datetime.toordinal()
Method in Python
The said integer representation of dates is invaluable for tasks like sorting, comparison, and calculations.
The datetime.toordinal()
method facilitates this conversion, providing a straightforward way to transform a datetime
object into its corresponding ordinal representation.
Example code:
import datetime
# Obtain the current date as a datetime object
datetime_object = datetime.date.today()
# Convert the datetime object to its ordinal representation
toordinal = datetime_object.toordinal()
# Print the result
print(f"The ordinal of the date {datetime_object} is {toordinal}.")
It begins by importing the datetime
module, which contains the necessary functionalities for date and time manipulation.
The code then retrieves the current date using the date.today()
method, which returns a datetime
object representing the current date.
The toordinal()
method is then called on the datetime
object to convert it into its corresponding proleptic Gregorian ordinal.
Finally, it prints out the result using an f-string, displaying both the original datetime
object and its corresponding ordinal value.
Output:
The above code displays the Gregorian ordinal for today’s datetime
object.
Example 2: Enter Out-Of-Range Date as a Parameter to datetime.toordinal()
Method
The code snippet below demonstrates the usage of the toordinal()
method to convert a datetime
object representing a historical date into its corresponding ordinal value.
By understanding how this method works, we can effectively manipulate and analyze dates within Python programs.
Example code:
import datetime
# Create a datetime object representing January 1st, 18 AD
date = datetime.datetime(18, 1, 1)
# Convert the datetime object to its ordinal representation
toordinal = date.toordinal()
# Print the result
print(f"The ordinal value of the earliest Datetime {date} is {toordinal}")
It starts by importing the datetime
module, which provides the necessary functionalities for working with dates and times in Python.
The code then creates a datetime
object named date
, representing January 1st, 18 AD
. It’s worth noting that the datetime
constructor takes arguments in the order of year, month, and day.
The toordinal()
method is then invoked on the date
object, converting it into its proleptic Gregorian ordinal representation.
Finally, it prints out the result using an f-string, displaying both the original datetime
object and its corresponding ordinal value.
Output:
The date should be in the given range; otherwise, a ValueError
exception is raised.
Traceback (most recent call last):
File "main.py", line 3, in <module>
date = datetime.datetime(18, 0, 0)
ValueError: month must be in 1..12
Example 3: Find the Day Number Using the datetime.toordinal()
Method
The code snippet below showcases the utilization of the toordinal()
method to determine the ordinal representation of a significant historical event—the Independence Day of the United States celebrated on July 4, 1776
.
By understanding this method and its application in the context of historical dates, we can appreciate its value in various programming scenarios.
Example code:
import datetime
# Create a datetime object representing July 4, 1776
date = datetime.date(1776, 7, 4)
# Print the date of America's independence
print("America got its independence on {}".format(date))
# Convert the datetime object to its ordinal representation
toordinal = date.toordinal()
# Print the ordinal representation of America's independence
print(
"America got its independence on {}th day if you count from 01/01/01".format(
toordinal
)
)
It starts by importing the datetime
module, which provides functionalities for working with dates and times in Python.
The code then creates a datetime
object named date
, representing the significant date of July 4, 1776
, when the United States declared its independence from British rule.
Then, it prints a message indicating the date of America’s independence, formatted using the .format()
method to include the date
object.
Next, it calls the toordinal()
method on the date
object to convert it into its proleptic Gregorian ordinal representation.
Finally, we print a message indicating the ordinal representation of America’s independence, formatted to include the toordinal
value.
Output:
The output confirms that July 4, 1776
corresponds to the 663,678th day since January 1, 1 AD
, according to the proleptic Gregorian calendar.
Like the above code, you can mention any specific date you want to find the day number.
Example 4: Maximum and Minimum Values of the datetime.toordinal()
Method
The code snippet below demonstrates the use of the toordinal()
method to determine the ordinal representation of two extreme dates: the earliest possible date and the largest maximum date allowed in the Python datetime
module.
By examining these extreme cases, we can gain insights into the range and capabilities of the toordinal()
method when dealing with dates.
Example code:
import datetime
# Define the earliest possible date allowed
earliest_date = datetime.datetime(1, 1, 1, 0, 0, 0, 0)
# Print the ordinal number of the earliest possible date
print(
"The ordinal number of the earliest possible date allowed",
earliest_date,
" is: ",
earliest_date.toordinal(),
)
# Define the largest maximum date allowed
largest_date = datetime.datetime(9999, 12, 31, 23, 59, 59)
# Print the ordinal number of the largest maximum date
print(
"The ordinal number of the largest maximum date allowed",
largest_date,
" is: ",
largest_date.toordinal(),
)
It starts by importing the datetime
module, which provides functionalities for working with dates and times in Python.
Then, it defines the earliest_date
variable representing the earliest possible date allowed, which is January 1, 1 AD
, at midnight.
It prints a message indicating the ordinal number of the earliest possible date, calculated using the toordinal()
method.
Next, it defines the largest_date
variable representing the largest maximum date allowed, which is December 31, 9999 AD, at 23:59:59
.
Finally, the code prints a message indicating the ordinal number of the largest maximum date, also calculated using the toordinal()
method.
Output:
The output displays the ordinal numbers corresponding to the earliest possible date and the largest maximum date allowed, indicating their positions on the proleptic Gregorian calendar.
The Gregorian calendar is only defined until 9999
, and a year number greater than this is not allowed.
ValueError: year 0 is out of range
Encounter in Using datetime.toordinal()
Method
In this example, we’re attempting to create a datetime
object with invalid values (year, month, and day set to 0).
This will result in a ValueError
because the datetime
constructor does not accept values outside the valid range.
As a result, the attempt to call toordinal()
on the invalid date will raise an error.
Example code:
import datetime
# Attempting to create a datetime object with invalid values
invalid_date = datetime.datetime(0, 0, 0)
# Trying to get the ordinal value of the invalid date
try:
ordinal_invalid_date = invalid_date.toordinal()
print("The ordinal value of the invalid date is:", ordinal_invalid_date)
except ValueError as e:
print("Error occurred:", e)
Output:
Traceback (most recent call last):
File "C:/Users/Admin/Desktop/main.py", line 13, in <module>
invalid_date = datetime.datetime(0, 0, 0)
ValueError: year 0 is out of range
Let’s correct the code snippet to handle the invalid date creation properly, specifically addressing the ValueError
when the year is set to 0.
This helps the program to be more functional and able to detect when the input values are invalid.
Corrected Code:
import datetime
def safe_toordinal(date):
try:
ordinal_date = date.toordinal()
return ordinal_date
except AttributeError:
print("Error: Input must be a valid datetime object.")
except ValueError as e:
print("Error occurred:", e)
# Attempting to create a datetime object with invalid values
try:
invalid_date = datetime.datetime(0, 1, 1)
except ValueError as e:
print("Error occurred:", e)
invalid_date = None
# Using the safe_toordinal function to handle potential errors
if invalid_date is not None:
ordinal_invalid_date = safe_toordinal(invalid_date)
if ordinal_invalid_date is not None:
print("The ordinal value of the invalid date is:", ordinal_invalid_date)
First, a function safe_toordinal()
is defined to encapsulate the conversion process within a try-except
block. Inside the function, toordinal()
is called on the input date
, and any potential AttributeError
or ValueError
exceptions are caught.
If an AttributeError
occurs, indicating that the input is not a valid datetime
object, an appropriate error message is printed. If a ValueError
occurs during the conversion, the specific error message is printed as well.
Next, an attempt is made to create a datetime
object with invalid values (year 0, month 1, day 1) within a try-except
block. If this attempt fails due to a ValueError
(e.g., year 0 is out of range), the specific error message is printed, and the invalid_date
variable is set to None
.
Finally, if invalid_date
is not None
, indicating that the datetime
object creation was successful, the safe_toordinal()
function is called to handle potential errors during the conversion. If the conversion is successful, the ordinal value of the invalid date is printed.
Output:
Error occurred: year 0 is out of range
Conclusion
In conclusion, Python’s datetime.toordinal()
method is a valuable tool for converting datetime
objects into their proleptic Gregorian ordinal representations.
It plays a crucial role in various programming scenarios, such as historical date analysis, chronological sorting, and date-based calculations. However, it’s essential to handle potential errors gracefully, especially when dealing with invalid input values that may result in exceptions like ValueError
.
By encapsulating the conversion process within a try-except
block and validating input data beforehand, developers can ensure the robustness and reliability of their datetime
-related operations.
Remember to anticipate potential errors and implement appropriate error handling mechanisms to make your code more resilient and user-friendly.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn