Python datetime.time() Class
Musfirah Waseem
Jan 30, 2023
Python
Python Datetime
-
Syntax of Python
datetime.time()
Class -
Example 1: Use the
datetime.time()
Class in Python -
Example 2: Enter Out of Range Values in
datetime.time()
Class -
Example 3: Display Some Arguments of the
datetime.time()
Class
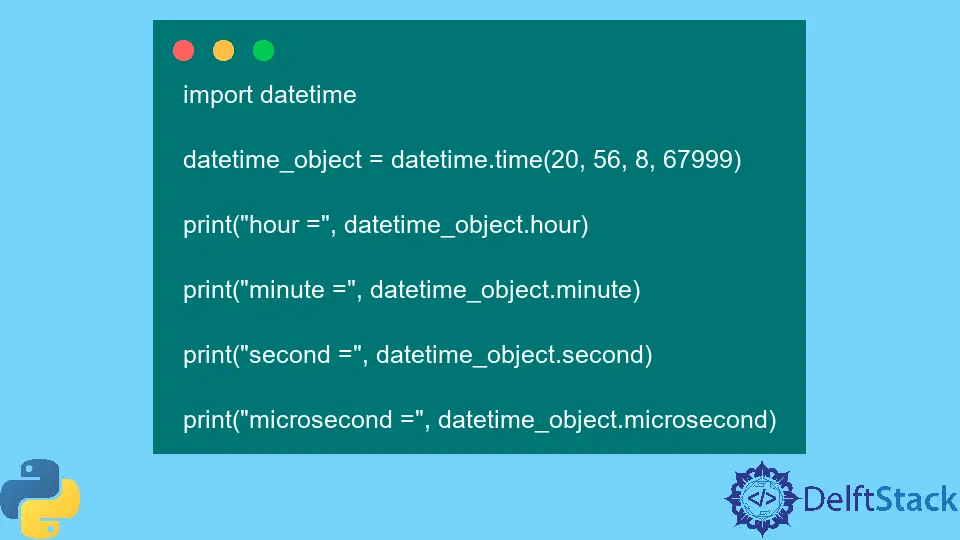
Python datetime.time()
class is an efficient way of handling local time in Python. When an object of the datetime.time()
class is instantiated, it represents the time in a specified format.
Syntax of Python datetime.time()
Class
datetime.time()
datetime.time(hour, minute, second, microsecond, tzinfo)
Parameters
hour |
(optional) It is an integer in the range: 0 <= hour < 24 . |
minute |
(optional) It is an integer in the range: 0 <= minute < 60 . |
second |
(optional) It is an integer in the range: 0 <= second < 60 . |
microsecond |
(optional) It is an integer in the range: 0 <= microsecond < 1000000 . |
tzinfo |
(optional) By default it is set to None . It is an instance of a tzinfo subclass. |
Return
This class does not return a value.
Example 1: Use the datetime.time()
Class in Python
import datetime
a = datetime.time()
print("Minimum time value= ", a)
b = datetime.time(23, 59, 59, 999999)
print("Maximum time value= ", b)
c = datetime.time(hour=11, minute=34, second=56)
print("Time entered= ", c)
Output:
Minimum time value= 00:00:00
Maximum time value= 23:59:59.999999
Time entered= 11:34:56
The above code shows only the attributes which we have specified.
Example 2: Enter Out of Range Values in datetime.time()
Class
import datetime
datetime_object = datetime.time(24, 59, 59, 999999)
print("The date and time entered are: ", datetime_object)
Output:
Traceback (most recent call last):
File "main.py", line 3, in <module>
datetime_object = datetime.time(24, 59, 59, 999999)
ValueError: hour must be in 0..23
The hour, minute, and second can never be out of range. So, any argument entered outside the results of the above-specified range in a ValueError
exception.
Example 3: Display Some Arguments of the datetime.time()
Class
import datetime
datetime_object = datetime.time(20, 56, 8, 67999)
print("hour =", datetime_object.hour)
print("minute =", datetime_object.minute)
print("second =", datetime_object.second)
print("microsecond =", datetime_object.microsecond)
Output:
hour = 20
minute = 56
second = 8
microsecond = 67999
We can use the .
dot notation to access specific parts of the time
object.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Musfirah Waseem
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn