Python datetime.datetime.timetuple() Method
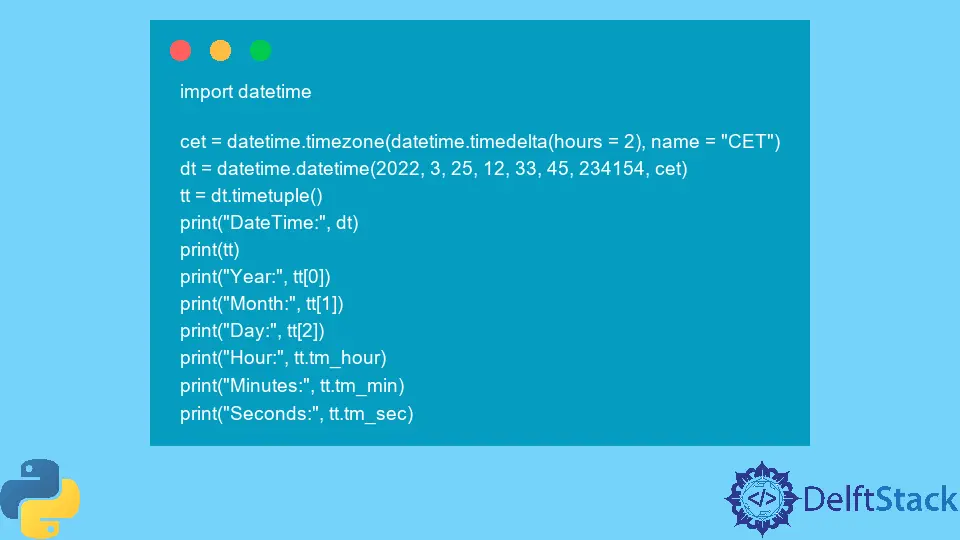
The datetime
library is a built-in Python library that gives numerous utilities such as classes, methods, and attributes to work with dates, time, date and time together (DateTime), timezones, and timedelta (time difference).
This library has a class, namely, datetime
, that eases the process of working with date and time. This class has multiple class functions that allow programmers to craft datetime
objects with the help of string formats such as ISO, timestamps, and ordinals.
Every datetime
object has several instance methods such as date()
, time()
, timetz()
, etc. One such method is the timetuple()
method.
The timetuple()
method returns an object of a named tuple time.struct_time
. The struct_time
is yet another object in the datetime
module.
In this article, we will talk about this method and this object in detail with the help of some relevant examples.
Syntax
<datetime-object > .timetuple()
Parameters
The timetuple()
method doesn’t accept any parameters.
Returns
The timetuple()
method returns an object of a named tuple time.struct_time
. Since it is an object of a named tuple, its values can be accessed using indexes and attributes’ names.
It has the following attributes.
Index | Attribute | Values |
---|---|---|
0 |
tm_year |
Any valid numerical year |
1 |
tm_mon |
[1, 12] |
2 |
tm_mday |
[1, 31] |
3 |
tm_hour |
[0, 23] |
4 |
tm_min |
[0, 59] |
5 |
tm_sec |
[0, 59] |
6 |
tm_wday |
[0, 6] , where 0 refers to Monday |
7 |
tm_yday |
[1, 366] |
8 |
tm_isdst |
0 , 1 , or -1 |
- | tm_zone |
An abbreviation of timezone name |
- | tm_gmtoff |
The offset east of UTC in seconds |
The time.struct_time
objects are returned by methods such as gmtime()
, localtime()
, and strptime()
. The year day or yday
is calculated using the following instruction.
yday = d.toordinal() - date(d.year, 1, 1).toordinal() + 1
The tm_isdst
Boolean flag of the output is set according to the dst()
method available on a timezone
object. Every class that subclasses the abstract tzinfo
class has to implement this method.
To learn more about the dst()
method and how its value is determined, refer to the official Python documentation here.
To learn about the named tuple time.struct_time
, refer to the official Python documentation here.
Example Code: Use datetime.datetime.timetuple()
Method in Python
import datetime
cet = datetime.timezone(datetime.timedelta(hours=2), name="CET")
dt = datetime.datetime(2022, 3, 25, 12, 33, 45, 234154, cet)
tt = dt.timetuple()
print("DateTime:", dt)
print(tt)
print("Year:", tt[0])
print("Month:", tt[1])
print("Day:", tt[2])
print("Hour:", tt.tm_hour)
print("Minutes:", tt.tm_min)
print("Seconds:", tt.tm_sec)
Output:
DateTime: 2022-03-25 12:33:45.234154+02:00
time.struct_time(
tm_year=2022,
tm_mon=3,
tm_mday=25,
tm_hour=12,
tm_min=33,
tm_sec=45,
tm_wday=4,
tm_yday=84,
tm_isdst=-1
)
Year: 2022
Month: 3
Day: 25
Hour: 12
Minutes: 33
Seconds: 45
- The Python code above first creates a timezone-aware
datetime
object with the timezone set to CET or Central European Time. - Next, it creates a
time.struct_time
object using thetimetuple()
instance method and prints it. - Furthermore, various attributes such as year, month, hour, and seconds are accessed using indexes and attribute names.
Note that if attributes are accessed using invalid indexes and wrong attribute names, the Python interpreter raises an IndexError
and an AttributeError
exception, respectively.