Python datetime.time.time.strftime() Method
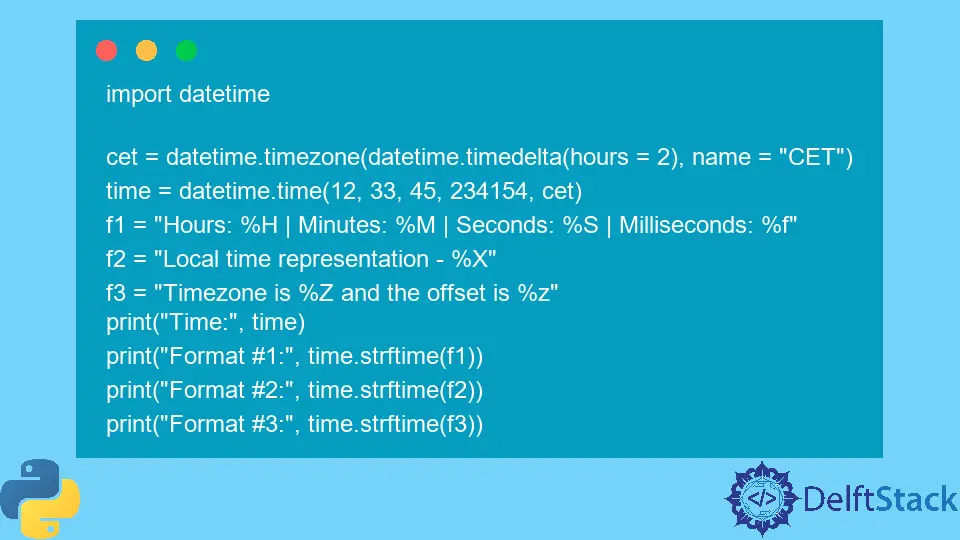
Python is a dynamically typed and famous general-purpose programming language. Currently, it is employed for a variety of use cases.
Because of the ease and robustness that it delivers, it has a ton of support from the community. Python has a built-in module, datetime
, that makes functioning with dates, times, time deltas, and time zones a piece of cake.
The datetime
library provides several methods and classes such as datetime
, date
, time
, tzinfo
, timezone
, and timedelta
to work with dates and times. These classes are saturated with numerous functions and attributes and offer dynamic utilities.
Every datetime.time
object of this module has access to a member function, namely, strftime()
, that lets developers convert a time
object to a string representation with the help of placeholders. More formally, these placeholders are also known as format codes.
Every format code is associated with a specific value in a time
object, and this method is smart enough to recognize them and replace them with relevant values.
In this article, we will dive deep into the strftime()
method and format codes and comprehensively understand both with the help of some pertinent examples.
Syntax
time.strftime(format)
Parameters
Parameters | Type | Description |
---|---|---|
format |
String | A string with format codes representing a string format for the time object. |
The following are the format codes used in the format string.
Format Code | Description | Examples |
---|---|---|
%H |
A zero-padded decimal number for an hour in a 24-hour clock. | 00, 01, 02, 03, …, 23 |
%I |
A zero-padded decimal number for an hour in a 12-hour clock. | 01, 02, 03, 04, …, 12 |
%p |
A locale’s equivalent of either AM or PM. | AM, PM, am, and pm |
%M |
A zero-padded decimal number for minutes. | 00, 01, 02, 03, …, 59 |
%S |
A zero-padded decimal number for seconds. | 00, 01, 02, 03, …, 59 |
%f |
A six-digit zero-padded decimal number for milliseconds. | 000000, 000001, 000002, 000003, …, 999999 |
%z |
A UTC offset in the format ±HHMM[SS[.ffffff]] . In the case of a naive object, it is an empty string. |
+0000, -030712.345216, +1030 |
%Z |
Name of the time zone. | UTC, IST, CET, GMT |
%X |
Time representation in the local system. | 17:24:03, 01:22:58 |
Returns
The strftime()
method returns a string representation of a time
object.
Example Code: Use datetime.time.time.strftime()
in Python
import datetime
cet = datetime.timezone(datetime.timedelta(hours=2), name="CET")
time = datetime.time(12, 33, 45, 234154, cet)
f1 = "Hours: %H | Minutes: %M | Seconds: %S | Milliseconds: %f"
f2 = "Local time representation - %X"
f3 = "Timezone is %Z and the offset is %z"
print("Time:", time)
print("Format #1:", time.strftime(f1))
print("Format #2:", time.strftime(f2))
print("Format #3:", time.strftime(f3))
Output:
Time: 12:33:45.234154+02:00
Format #1: Hours: 12 | Minutes: 33 | Seconds: 45 | Milliseconds: 234154
Format #2: Local time representation - 12:33:45
Format #3: Timezone is CET and the offset is +0200
- The Python code above first creates a timezone-aware
time
object and sets the timezone to CET or Central European Time which is2
hours ahead of UTC. - Next, it declares
3
string formats for the time object. - The first format uses hour, minutes, seconds, and milliseconds format codes.
- The second format uses the format code for local time representation.
- The third format uses format codes for the timezone’s name and timezone offset.
- Finally, using the
strftime()
method, the time is printed in these three formats.