Python datetime.time.replace() Method
- Syntax
-
Example 1: Adjusting Time Components Using
datetime.time.replace()
-
Example 2: Use
datetime.time.replace()
to Modify Time and Timezone Details -
Example 3: Use
datetime.time.replace()
to Convert a Timezone-Awaretime
to a Naive Instance -
Example 4: Setting Daily Reminder With Adjustable Time Using
datetime.time.replace()
- Conclusion
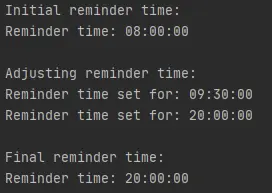
Python is a dynamic and popular general-purpose programming language. It is enriched with numerous use cases, has access to various third-party libraries, and has a ton of support from its community.
It offers multiple built-in modules, one of them being the datetime
module, which provides powerful functionalities to work with dates and times.
This module offers methods and classes such as date
, datetime
, tzinfo
, timezone
, and timedelta
to work with date and time. Sometimes, while working with time
objects, you might need to modify specific components like hours, minutes, or seconds.
The replace()
method of the datetime.time
class comes in handy for such scenarios. This method creates a new time
object with the specified components replaced with new values while keeping the rest unchanged.
This can be particularly useful when you want to generate new time instances with slight modifications, such as setting a different hour or minute.
In this article, we will cover this method in detail with the help of some relevant examples.
Syntax
time.replace(
hour=self.hour,
minute=self.minute,
second=self.second,
microsecond=self.microsecond,
tzinfo=self.tzinfo,
fold=0,
)
Parameters
Parameters | Type | Description |
---|---|---|
hour |
Integer | An hour value between 0 and 23, inclusive. |
minute |
Integer | A minute value between 0 and 59, inclusive. |
second |
Integer | A second value between 0 and 59, inclusive. |
microsecond |
Integer | A microsecond value between 0 and 999999, inclusive. |
tzinfo |
A subclass of datetime.tzinfo |
A concrete class subclassing the abstract datetime.tzinfo class. |
fold |
Integer | A binary field representing an invalid date. Here, 1 means an invalid date. |
Returns
The replace()
method returns a new time
object with all the same attributes as the original time
object, except for those attributes for which new values were provided.
Note that if tzinfo
is set to None
, a timezone-unaware datetime
object is returned for which no conversions are performed for time values.
By default, for naïve or timezone-unaware objects, time is stored according to UTC or Coordinated Universal Time.
Example 1: Adjusting Time Components Using datetime.time.replace()
Suppose we have a time
object representing an event scheduled at 5:30 PM
. However, we need to change the minute component to 45
.
We can achieve this using the replace()
method of the datetime.time
class.
Example code:
import datetime
# Define the original time
original_time = datetime.time(17, 30)
# Print the original time
print("Original Time:", original_time)
# Replace the minute component with 45
adjusted_time = original_time.replace(minute=45)
# Print the adjusted time
print("Adjusted Time:", adjusted_time)
We import the datetime
module and define the original time
object original_time
using the datetime.time()
constructor, specifying 17
(5 PM
) for the hour and 30
for the minute (representing 5:30 PM
).
The original time is printed first.
Then, using the replace()
method, we create a new time
object adjusted_time
with the minute component replaced by 45
.
Lastly, the adjusted time is printed.
Output:
It will now output 5:45PM
as the adjusted time.
This example demonstrates how to utilize the datetime.time.replace()
method to modify specific components of a time
object, such as hours, minutes, or seconds while preserving other components unchanged.
Example 2: Use datetime.time.replace()
to Modify Time and Timezone Details
The code below demonstrates the usage of the datetime.time.replace()
method to manipulate time
objects while also incorporating timezone information.
It showcases how to create, modify, and handle time
objects in Python effectively.
Example code:
import datetime
# Define timezones
cet = datetime.timezone(datetime.timedelta(hours=2), name="CET")
ist = datetime.timezone(datetime.timedelta(hours=5, minutes=30), name="IST")
# Create a time object with timezone information
time = datetime.time(12, 33, 45, 234154, cet)
# Print original time and timezone
print("Time:", time)
print("Timezone:", time.tzname())
# Replace time components
new_time = time.replace(hour=10, minute=11, second=30, microsecond=999999)
print("Changed Time:", new_time)
# Change timezone
time = time.replace(tzinfo=ist)
print("Time:", time)
print("Changed Timezone:", time.tzname())
We import the datetime
module to use its function in the code.
Two timezone objects, cet
(Central European Time) and ist
(Indian Standard Time), are defined using datetime.timezone()
, specifying the UTC offsets.
Then, a time
object time
is created using the datetime.time()
constructor, with hour, minute, second, microsecond, and timezone provided as arguments.
The original time and timezone information are printed using print()
.
Next, the replace()
method is used to create a new time
object new_time
, where specific components (hour, minute, second, microsecond) are modified.
We change the timezone of the original time
object using the replace()
method with the tzinfo
argument set to ist
.
Finally, the modified time and timezone information are printed.
Output:
The time changed from 12:33:45.234154+02:00
to 12:33:45.234154+05:30
. The tzname()
method verifies that the timezone is IST.
The code demonstrates how the datetime.time.replace()
method allows for easy manipulation of time
objects, enabling modifications to specific components such as hour, minute, second, and microsecond.
Example 3: Use datetime.time.replace()
to Convert a Timezone-Aware time
to a Naive Instance
The code exemplifies the process of converting a timezone-aware datetime.time
object to a naive instance using the replace()
method.
This operation is valuable in scenarios where you need to standardize time representation across different parts of your application or when timezone information becomes unnecessary.
It illustrates how to set a time
object’s timezone to None
, effectively removing the timezone information.
Example code:
import datetime
# Define timezone (CET - Central European Time)
cet = datetime.timezone(datetime.timedelta(hours=2), name="CET")
# Create a time object with timezone information
time = datetime.time(12, 33, 45, 234154, cet)
# Print original time and timezone
print("Time:", time)
print("Timezone:", time.tzname())
# Remove timezone information
time = time.replace(tzinfo=None)
print("Changed Timezone:", time)
print("Timezone:", time.tzname())
We begin by importing the datetime
module. Next, we define the Central European Time (CET) timezone object (cet
) using the datetime.timezone()
constructor, specifying a UTC offset of 2 hours ahead of UTC.
Then, we create a timezone-aware time
object named time
using the datetime.time()
constructor, supplying hour, minute, second, microsecond, and timezone arguments.
The original time and timezone information are printed.
Subsequently, we utilize the replace()
method to convert the timezone-aware time
object into a naive instance by setting its tzinfo
attribute to None
.
Finally, we print the changed timezone information, which should now be None
, indicating that the instance is no longer associated with any timezone.
Output:
From the output, we can understand that the time values did not revert to UTC-based values; only the timezone changed. We can verify that by using the tzname()
method.
The timezone name is printed as None
, indicating no timezone is present. Moreover, we can confirm that from the output.
The updated time
object doesn’t have a ±HH:MM
value at the end of it (12:33:45.234154
), unlike the original object where the value is +02:00
(12:33:45.234154+02:00
), signaling that there is no timezone.
Note that the
tzname()
method sometimes raises an exception if it fails to find a string name for the timezone. To bypass that, we can usetry-except
blocks and catch the exception.
Example 4: Setting Daily Reminder With Adjustable Time Using datetime.time.replace()
For a practical application example, consider a situation where a user wants to set a daily reminder for a task, such as taking medication, at a certain time.
However, the user may want to adjust the reminder time occasionally without recreating the entire reminder.
Example code:
import datetime
# Define the default reminder time
reminder_time = datetime.time(8, 0) # Set for 8:00 AM initially
# Function to set the reminder time
def set_reminder(hour, minute):
global reminder_time
reminder_time = reminder_time.replace(hour=hour, minute=minute)
print("Reminder time set for:", reminder_time)
# Set the initial reminder time
print("Initial reminder time:")
print("Reminder time:", reminder_time)
# Adjust the reminder time
print("\nAdjusting reminder time:")
set_reminder(9, 30) # Set reminder for 9:30 AM
set_reminder(20, 0) # Set reminder for 8:00 PM
# Print the final reminder time
print("\nFinal reminder time:")
print("Reminder time:", reminder_time)
We begin by importing the datetime
module to access its functionalities.
We set a default reminder time of 8:00 AM
using the datetime.time()
constructor.
Subsequently, we define a function set_reminder()
to adjust the reminder time flexibly. Within this function, we utilize the replace()
method to modify the hour and minute components of the reminder_time
object.
We print the initial reminder time to display the default setting. Then, we invoke the set_reminder()
function twice, altering the reminder time to 9:30 AM
and later to 8:00 PM
.
Finally, we print the updated reminder time to confirm the modifications.
Output:
The reminder time now is 8:00PM
.
This example showcases a practical application of the datetime.time.replace()
method in setting daily reminders with adjustable time.
Conclusion
The datetime
module, among its many offerings, provides robust functionalities for handling dates and times. Within this module, the replace()
method of the datetime.time
class stands out for its ability to modify time
objects efficiently.
This method enables developers to adjust specific components of time
objects, such as hours, minutes, or seconds, while maintaining other attributes unchanged.
Through various examples, we’ve demonstrated the versatility of the replace()
method, from adjusting time components for specific reminders to converting timezone-aware instances to naive ones.
These examples illustrate the method’s practical applications across different scenarios, showcasing its importance in datetime
manipulation tasks.
By mastering the replace()
method and understanding its nuances, Python developers can enhance their ability to manage and manipulate time-related data effectively in their applications.