Python datetime.date.date.fromtimestamp() Method
- Syntax
-
Example 1: Get POSIX Timestamp From Timezone-Aware
datetime
Objects -
Example 2: Get POSIX Timestamp From Naive
datetime
Objects -
Example 3: Use
fromtimestamp()
to Get Local Date From a POSIX Timestamp -
Example 4: Errors and Exceptions Occurring When Using
fromtimestamp()
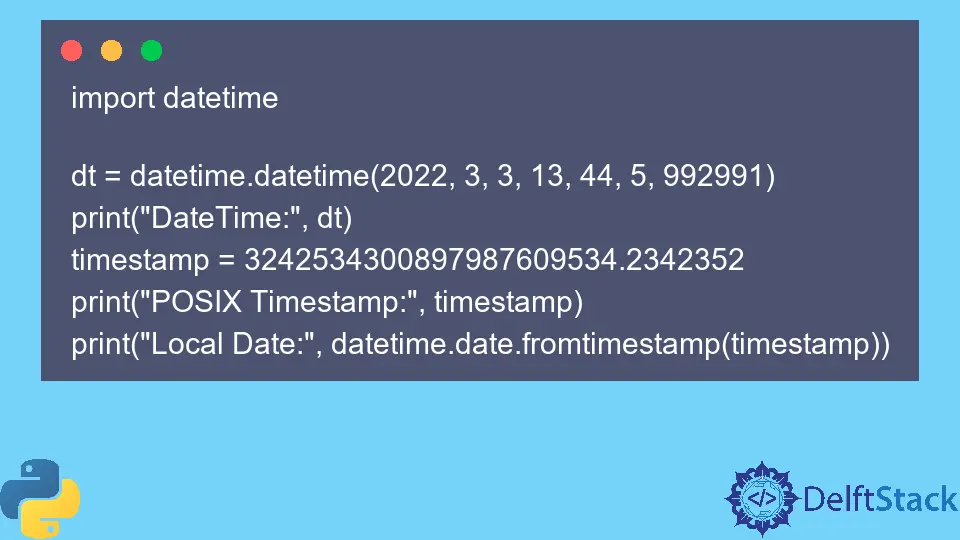
The datetime
library is built-in and a rich Python library that offers various utilities such as classes, methods, structures, and attributes to work with dates, time, date and time combined (DateTime), timezones, and timedelta (time difference).
This module contains a class, namely, date
, that eases the flow of working with dates. This class has several methods that allow developers to create date
objects from string formats, timestamps, ISO format, and ordinals.
This article will comprehensively discuss one member function, fromtimestamp()
, that helps us create date
objects from POSIX or Portable Operating System Interface timestamps. POSIX timestamps are also known as Unix timestamps.
POSIX is a system representing time as the number of seconds elapsed since 00:00:00 UTC on 1 January 1970
. This date is also known as the Unix epoch
.
Syntax
datetime.date.fromtimestamp(timestamp)
Parameters
To get a POSIX or Portable Operating System Interface timestamp, we can use the timestamp()
method available on datetime
objects. This method returns a float value representing a POSIX timestamp.
Returns
The fromtimestamp()
method returns the local date associated with a POSIX or Unix timestamp.
Example 1: Get POSIX Timestamp From Timezone-Aware datetime
Objects
In timezone-aware datetime
objects, it is straightforward to get the timestamp. Internally, the return value of the following instruction is the result of the timestamp()
method.
(dt - datetime(1970, 1, 1, tzinfo=timezone.utc)).total_seconds()
Refer to the following Python code.
import datetime
cet = datetime.timezone(datetime.timedelta(hours=2), name="CET")
dt = datetime.datetime(2022, 3, 3, 13, 44, 5, 992991, cet)
print("DateTime:", dt)
print("Timestamp:", dt.timestamp())
Output:
DateTime: 2022-03-03 13:44:05.992991+02:00
Timestamp: 1646307845.992991
Example 2: Get POSIX Timestamp From Naive datetime
Objects
There are two ways to fetch the timestamp in naive datetime
objects unaware of the timezone. It is possible to get the POSIX timestamp using the timestamp()
without using the upcoming two approaches, but sometimes, the timestamp()
method raises an OverflowError
.
It does so because it calculates the timestamp using the mktime()
method available in the C platform and assumes that naive objects store local system time. Moreover, the datetime
objects operate on a huge domain of values, which are not often available using the mktime()
method.
Hence, we get exceptions. To learn more about it, refer to the official documentation here.
Coming back to the two approaches, in the first method, we manually set the timezone of the datetime
object to UTC and invoke the timestamp()
method. Refer to the following Python code for this approach.
import datetime
dt = datetime.datetime(2022, 3, 3, 13, 44, 5, 992991)
print("DateTime:", dt)
timestamp = dt.replace(tzinfo=datetime.timezone.utc).timestamp()
print("Timestamp:", timestamp)
Output:
DateTime: 2022-03-03 13:44:05.992991
Timestamp: 1646315045.992991
And in the second approach, we calculate the time by performing some datetime
object mathematics. Refer to the following Python code for this approach.
import datetime
dt = datetime.datetime(2022, 3, 3, 13, 44, 5, 992991)
print("DateTime:", dt)
timestamp = (dt - datetime.datetime(1970, 1, 1)) / datetime.timedelta(seconds=1)
print("Timestamp:", timestamp)
Output:
DateTime: 2022-03-03 13:44:05.992991
Timestamp: 1646315045.992991
Example 3: Use fromtimestamp()
to Get Local Date From a POSIX Timestamp
import datetime
dt = datetime.datetime(2022, 3, 3, 13, 44, 5, 992991)
print("DateTime:", dt)
timestamp = dt.replace(tzinfo=datetime.timezone.utc).timestamp()
print("POSIX Timestamp:", timestamp)
print("Local Date:", datetime.date.fromtimestamp(timestamp))
Output:
DateTime: 2022-03-03 13:44:05.992991
POSIX Timestamp: 1646315045.992991
Local Date: 2022-03-03
The Python code above first creates a naive datetime
object. Next, the first approach we discussed above fetches the timestamp from the datetime
object.
Next, the fromtimestamp()
class method extracts the local date from the POSIX timestamp.
Example 4: Errors and Exceptions Occurring When Using fromtimestamp()
OverflowError
The fromtimestamp()
method, in case the timestamp
is out of the range of values supported by the platform C localtime()
method, raises an OverflowError
error. Refer to the following Python code for an example.
import datetime
dt = datetime.datetime(2022, 3, 3, 13, 44, 5, 992991)
print("DateTime:", dt)
timestamp = 3242534300897987609534.2342352
print("POSIX Timestamp:", timestamp)
print("Local Date:", datetime.date.fromtimestamp(timestamp))
Output:
DateTime: 2022-03-03 13:44:05.992991
POSIX Timestamp: 3.2425343008979875e+21
Traceback (most recent call last):
File "main.py", line 7, in <module>
print("Local Date:", datetime.date.fromtimestamp(timestamp))
OverflowError: timestamp out of range for platform time_t
ValueError
The fromtimestamp()
method (in case the timestamp represents an invalid year value) raises a ValueError
error. Refer to the following Python code for an example.
import datetime
dt = datetime.datetime(2022, 3, 3, 13, 44, 5, 992991)
print("DateTime:", dt)
timestamp = -100000000000
print("POSIX Timestamp:", timestamp)
print("Local Date:", datetime.date.fromtimestamp(timestamp))
Output:
DateTime: 2022-03-03 13:44:05.992991
POSIX Timestamp: -100000000000
Traceback (most recent call last):
File "main.py", line 7, in <module>
print("Local Date:", datetime.date.fromtimestamp(timestamp))
ValueError: year -1199 is out of range
OSError
The fromtimestamp()
method, if the localtime()
method, called internally fails, raises an OSError
error.