Python datetime.isoformat() Method
-
Syntax of Python
datetime.isoformat()
Method -
Example 1: Use
datetime.isoformat()
Method in Python -
Example 2: Use Different Time-Specific Parameters in the
datetime.isoformat()
Method -
Example 3: Convert String to
datetime
Object Using thedatetime.isoformat()
Method
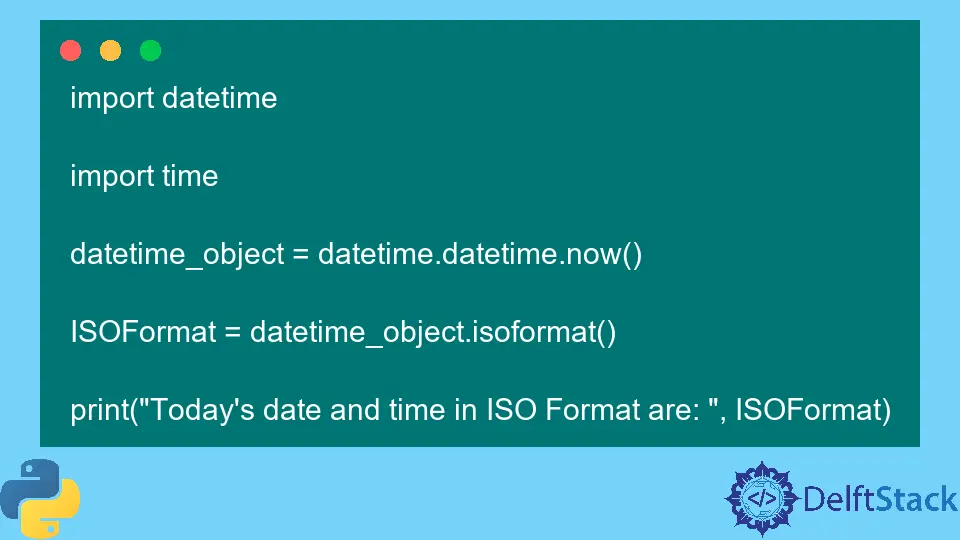
Python datetime.isoformat()
method is an efficient way of finding a string of date, time, and UTC offset corresponding to the time zone in ISO 8601
format. The standard ISO 8601
format uses date formats per the Gregorian calendar.
Syntax of Python datetime.isoformat()
Method
datetime.isoformat(sep, timespec)
Parameters
sep |
(Optional parameter) A separator character to be printed between the date and time fields. The default value is T . |
timespec |
(Optional parameter) A format specifier for the timespec . The default value is auto . The following values can also be entered as arguments: |
hours |
The returned time component will only have hours in HH format. |
minutes |
The returned time component will only have hours and minutes in the HH:MM format. |
seconds |
The returned time component will be displayed in the HH:MM:SS format. |
milliseconds |
The returned time component will be in the HH:MM:SS:mmm format, where mmm stands for milliseconds. |
microseconds |
The returned time component will be in the HH:MM:mmmmmm format, where mmmmmm stands for microseconds. |
auto |
The returned time component will be in HH:MM:SS format. If the microseconds component is available, then it will be printed. |
Return
The return type of this method is a date object in ISO 8601
format.
Example 1: Use datetime.isoformat()
Method in Python
import datetime
import time
datetime_object = datetime.datetime.now()
ISOFormat = datetime_object.isoformat()
print("Today's date and time in ISO Format are: ", ISOFormat)
Output:
Today's date and time in ISO Format are: 2022-08-29T15:14:33.711834
The above code gives us the date and time in the standard format.
Example 2: Use Different Time-Specific Parameters in the datetime.isoformat()
Method
import datetime
ISOFormat = datetime.datetime.now()
print(ISOFormat.isoformat("#", "auto"))
print(ISOFormat.isoformat("#", "hours"))
print(ISOFormat.isoformat("#", "minutes"))
print(ISOFormat.isoformat("#", "seconds"))
print(ISOFormat.isoformat("#", "milliseconds"))
print(ISOFormat.isoformat("#", "microseconds"))
Output:
2022-08-29#15:21:16.921250
2022-08-29#15
2022-08-29#15:21
2022-08-29#15:21:16
2022-08-29#15:21:16.921
2022-08-29#15:21:16.921250
The separator character is always printed between the date and time fields.
Example 3: Convert String to datetime
Object Using the datetime.isoformat()
Method
import datetime
datetime_object = datetime.datetime(2022, 8, 29, 8, 40, 34, 686)
print("Input Datetime:", datetime_object)
ISOFormat = datetime_object.isoformat()
print("ISO format:", ISOFormat)
Output:
Input Datetime: 2022-08-29 08:40:34.000686
ISO format: 2022-08-29T08:40:34.000686
The standard ISO 8601
format represents the datetime
object using a 4-digit year, followed by a two-digit month and a two-digit day.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn