Pandas DataFrame DataFrame.isin() Function
-
Syntax of
pandas.DataFrame.isin(values)
-
Example Codes:
DataFrame.isin()
Withiterable
as the Input -
Example Codes:
DataFrame.isin()
WithDictionary
as the Input -
Example Codes:
DataFrame.isin()
WithSeries
as the Input -
Example Codes:
DataFrame.isin()
WithDataFrame
as the Input
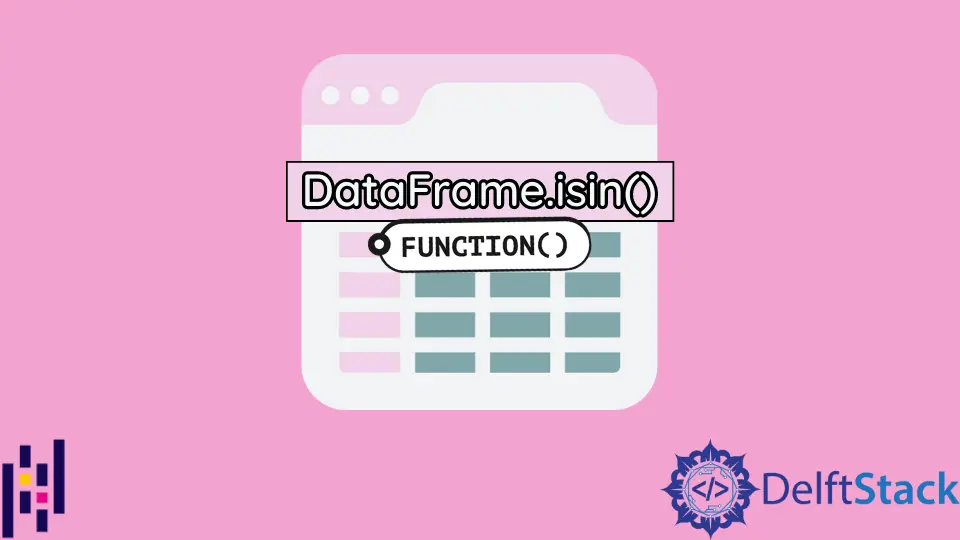
pandas.DataFrame.isin(values)
function checks whether each element in the caller DataFrame contains the value specified in the input values
.
Syntax of pandas.DataFrame.isin(values)
DataFrame.isin(values)
Parameters
values |
iterable - list , tuple , set , etc. Dictionary , Series DataFrame |
Return
It returns a DataFrame
of Booleans of the same dimension of the caller DataFrame
indicating whether each element contains the input values
.
Example Codes: DataFrame.isin()
With iterable
as the Input
When the Python iterable
is the input, Pandas DataFrame.isin()
function checks whether each value in the DataFrame
contains any value in the iterable
.
import pandas as pd
df = pd.DataFrame({'Sales': [100, 200], 'Profit': [200, 400]})
df = df.isin([200, 400])
print(df)
The caller DataFrame
is
Sales Profit
0 100 200
1 200 400
Output:
Sales Profit
0 False True
1 True True
Here, 200 and 400 present in the list [200, 400]
, therefore, the values in the returned DataFrame
whose original values are 200 and 400 are True
. 100
is not in the list [200, 400]
, therefore, the value in its position returns False
.
Example Codes: DataFrame.isin()
With Dictionary
as the Input
If the input value type is Dictionary
, isin()
function checks not only the values but also the key
. It returns True
only when the column name is the same as the key
and the cell value contains in the value
of the dictionary.
import pandas as pd
df = pd.DataFrame({"Sales": [100, 200], "Profit": [200, 400]})
df = df.isin({"Sales": [200, 400]})
print(df)
Output:
Sales Profit
0 False False
1 True False
In the first example, values of the Profit
columns are both True
but are False
in this example because the column name is different from the key
in the input dictionary.
It returns True
in the Sales
column if the value is contained in the value
of the dictionary - [200, 400]
.
Example Codes: DataFrame.isin()
With Series
as the Input
If the input value type is Pandas Series
, isin()
function checks whether the element per column is the same as the value in the same index of the input Series
.
import pandas as pd
df = pd.DataFrame({"Sales": [100, 200], "Profit": [200, 400]})
valueSeries = pd.Series([200, 400])
print(valueSeries)
df = df.isin(valueSeries)
print(df)
Output:
0 200
1 400
dtype: int64
Sales Profit
0 False True
1 False True
The elements in the Profit
column are the same as the elements in the input Series
element-wise, therefore, it returns True
for both elements in that column.
Example Codes: DataFrame.isin()
With DataFrame
as the Input
If the input value type is Pandas DataFrame
, isin()
function checks each element in the caller DataFrame
is the same as the element of the input DataFrame
at the same position.
It returns True
when values are identical, or False
in the case of mismatch.
import pandas as pd
df = pd.DataFrame({"Sales": [100, 200], "Profit": [200, 400]})
print(df)
valueDf = pd.DataFrame({"Sales": [100, 200], "Profit": [200, 300]})
print(valueDf)
df = df.isin(valueDf)
print(df)
Output:
Sales Profit
0 100 200
1 200 400
Sales Profit
0 100 200
1 200 300
Sales Profit
0 True True
1 True False
The value in the position (1, 1)
returns False
because the values are different between the caller DataFrame and the input DataFrame.
isin()
function checks not only the value element-wise, but it also checks whether the name of the column is identical. It returns False
if the column names are different even if the value is the same in these two DataFrames.Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook