NumPy numpy.meshgrid Function
Suraj Joshi
Jan 30, 2023
-
Syntax of
numpy.meshgrid()
: -
Example Codes:
numpy.meshgrid()
Method to Generatemeshgrids
-
Example Codes: Set
indexing='ij'
innumpy.meshgrid()
Method to Generatemeshgrids
-
Example Codes: Set
sparse=True
innumpy.meshgrid()
Method to Generatemeshgrids
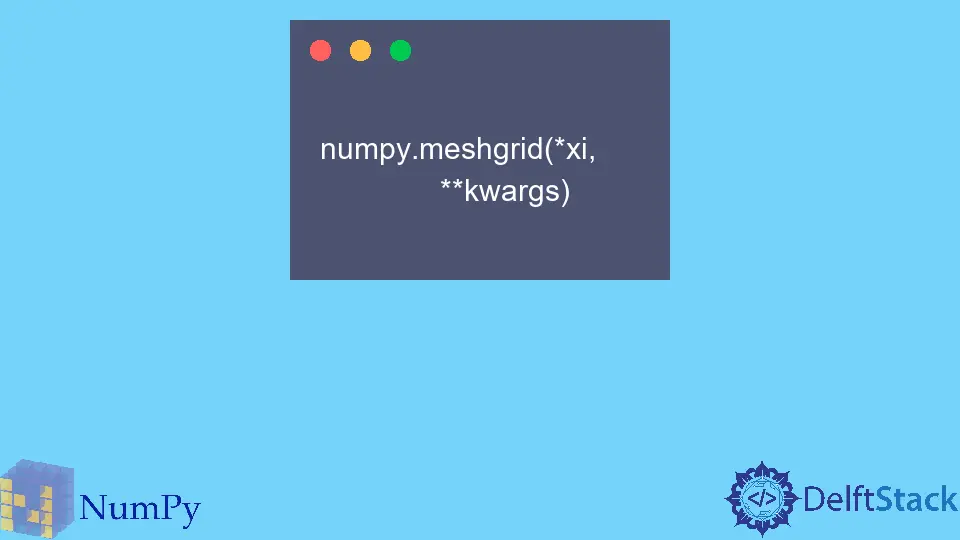
Python Numpynumpy.meshgrid()
function creates an N-dimensional rectangular grid from one-dimensional coordinate arrays x1, x2,…, xn
.
Syntax of numpy.meshgrid()
:
numpy.meshgrid(*xi, **kwargs)
Parameters
x1, x2,…, xn |
Array-like. 1-D array that represents the coordinates of the grid. |
indexing |
Array-like. defines the indexing of output. xy (Cartesian) or ij (matrix). |
sparse |
Boolean. Returns sparse grid in order to conserve memory(sparse=True ) |
copy |
Boolean. view into the original array is returned to conserve the memory(copy=True ) |
Return
Coordinate matrices from coordinate vectors.
Example Codes: numpy.meshgrid()
Method to Generate meshgrids
import numpy as np
x=np.linspace(2,5,4)
y=np.linspace(2,4,3)
xx,yy=np.meshgrid(x, y)
print("xx matrix:")
print(xx)
print("\n")
print("shape of xx matrix:")
print(xx.shape)
print("\n")
print("yy matrix:")
print(yy)
print("\n")
print("shape of yy matrix:")
print(yy.shape)
print("\n")
Output:
xx matrix:
[[2. 3. 4. 5.]
[2. 3. 4. 5.]
[2. 3. 4. 5.]]
shape of xx matrix:
(3, 4)
yy matrix:
[[2. 2. 2. 2.]
[3. 3. 3. 3.]
[4. 4. 4. 4.]]
shape of yy matrix:
(3, 4)
It creates matrix xx
and yy
such that the pairing of the corresponding element in each matrix gives the x
and y
coordinates of all the points in the grid.
Example Codes: Set indexing='ij'
in numpy.meshgrid()
Method to Generate meshgrids
import numpy as np
x=np.linspace(2,5,4)
y=np.linspace(2,4,3)
xx,yy=np.meshgrid(x,y,indexing='ij')
print("xx matrix:")
print(xx)
print("\n")
print("shape of xx matrix:")
print(xx.shape)
print("\n")
print("yy matrix:")
print(yy)
print("\n")
print("shape of yy matrix:")
print(yy.shape)
print("\n")
Output:
xx matrix:
[[2. 2. 2.]
[3. 3. 3.]
[4. 4. 4.]
[5. 5. 5.]]
shape of xx matrix:
(4, 3)
yy matrix:
[[2. 3. 4.]
[2. 3. 4.]
[2. 3. 4.]
[2. 3. 4.]]
shape of yy matrix:
(4, 3)
It creates the matrix xx
and yy
such that the pair formed corresponding elements of both elements from the index of matrix elements.
The matrices xx
and yy
are transposes of xx
and yy
in the earlier case.
Example Codes: Set sparse=True
in numpy.meshgrid()
Method to Generate meshgrids
import numpy as np
x=np.linspace(2,5,4)
y=np.linspace(2,4,3)
xx,yy=np.meshgrid(x,y,sparse=True)
print("xx matrix:")
print(xx)
print("\n")
print("shape of xx matrix:")
print(xx.shape)
print("\n")
print("yy matrix:")
print(yy)
print("\n")
print("shape of yy matrix:")
print(yy.shape)
print("\n")
Output:
xx matrix:
[[2. 3. 4. 5.]]
shape of xx matrix:
(1, 4)
yy matrix:
[[2.]
[3.]
[4.]]
shape of yy matrix:
(3, 1)
If we set sparse=True
in meshgrid()
method, it returns sparse grid to conserve memory.
Author: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn