JavaScript array.splice() Method
-
Syntax of JavaScript
array.splice()
Method -
Example 1: Use
array.splice()
to Add New Elements Without Removing Any Elements -
Example 2: Use
array.splice()
to Remove Numbers From an Array -
Example 3: Remove and Add Array Elements With
array.splice()
-
Example 4: Replace Elements Using
array.splice()
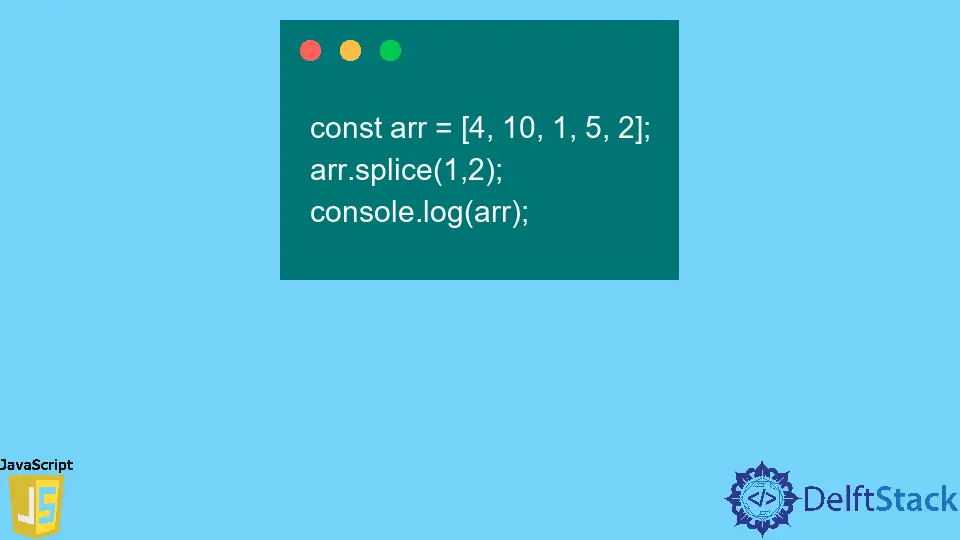
In JavaScript, the array.splice()
method adds or removes the elements of an array starting from the index position. We can also use this method to add the elements of another array.
Syntax of JavaScript array.splice()
Method
refarray.splice(index,0);
refarray.splice(index,howmany,item1,item2,...itemN);
Parameters
index |
To position in the array where the new elements will be added or removed. |
howmany |
To define the number of elements that need to be removed. |
item1,item2 |
The elements that will be added. |
Return
This method returns the given array after removing or adding the elements.
Example 1: Use array.splice()
to Add New Elements Without Removing Any Elements
We can use the array.splice()
method to add elements in a given array without removing any single element. This method requires the index position as a parameter for the new element.
In this example, we have created an array with two elements. Then, we used the array.splice()
method to add two new elements.
const arr = ["JavaScript","C++"];
arr.splice(1, 0, "Python", "Ruby");
console.log(arr);
Output:
[ 'JavaScript', 'Python', 'Ruby', 'C++' ]
Example 2: Use array.splice()
to Remove Numbers From an Array
Users can use the array.splice()
method to remove elements from the array. When we pass 2 parameters in the array.splice()
method, it removes the howmany
number of elements from the startIndex
.
In this example, we have created an array of 5 numbers. Then, we used the array.splice()
method to remove two elements from the index position of 1.
In the output, users can see that we got the array of 3 numbers after removing the two elements.
const arr = [4, 10, 1, 5, 2];
arr.splice(1,2);
console.log(arr);
Output:
[ 4,5,2 ]
Example 3: Remove and Add Array Elements With array.splice()
Programmers can add or remove array elements using the array.splice()
method by performing a single operation.
In the example below, we have taken 1 as a startIndex
, 1 as a howmany
, and 4 different items to add from the startIndex
. We have removed the 1 element from the startIndex
and added the 4 other elements at that position.
This way, users can add or remove the elements by the single call of array.splice()
.
const arr = ["Delft","stack","Netherland."];
arr.splice(1,1,"is","a","city","of");
console.log(arr);
Output:
[ 'Delft', 'is', 'a', 'city', 'of', 'Netherland.' ]
Example 4: Replace Elements Using array.splice()
If users want to replace array elements using the array.splice()
method, they have to pass the same number of items
as a parameter as the value of howmany
.
In this example, we have passed 1 as a value of howmany
and passed 1 item
as a parameter that will replace a single element in the given array.
const arr = ["Welcome","Programmers","on","DelftStack"];
arr.splice(1,1,"Users");
console.log(arr);
Output:
[ 'Welcome', 'Users', 'on', 'DelftStack' ]
The array.splice()
method adds or removes the elements of the given array.