JavaScript Array.reverse() Method
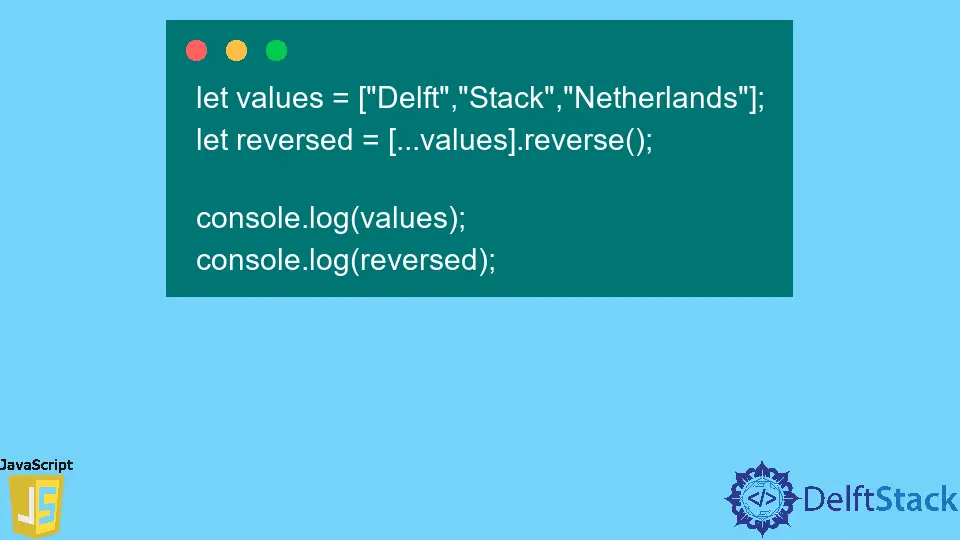
The array.reverse
is used to reverse an array in place, which means this method reverses the original array without using extra space. Reversing an array means the first element of the array goes to the last position, the second element goes to the second last, and so on.
Syntax
let array = [10, 20, 30];
array.reverse();
Parameters
It does not take any parameters.
Returns
The Array.reverse()
method reverses the an and returns the reference to that array.
Example Codes
Let’s use different code examples to learn Array.reverse()
method.
Use Array.reverse()
to Reverse an Array of Numbers
We created an array of numbers and used the Array.reverse()
to reverse the array.
After that, we printed the reversed array, and you can observe that the original array is reversed rather than returning the new reversed array.
let array = [10,20,30];
array.reverse();
console.log(array);
Output:
[ 30, 20, 10 ]
Create Shallow Copy of Array & Use the Array.reverse()
to Reverse It
Sometimes, we need to keep the original array as it is, but we also need a reversed array. In such cases, we can create a shallow copy of the array using the spread operator (...
), as shown in the example below.
We can observe in the output that the original array is as it is, and the Array.reverse()
method has returned the reference to the shallow copy of the original array.
let values = ["Delft","Stack","Netherlands"];
let reversed = [...values].reverse();
console.log(values);
console.log(reversed);
Output:
[ 'Delft', 'Stack', 'Netherlands' ]
[ 'Netherlands', 'Stack', 'Delft' ]
Note that we can also reverse the array-like object using the Array.reverse()
method.