JavaScript array.prototype Property
-
Syntax of JavaScript
array.prototype
Property -
Example 1: Use
array.prototype
to Get the Custom Word Added in Elements of the Array -
Example 2: Use
array.prototype
With the Function to Create a Prototype for the Array
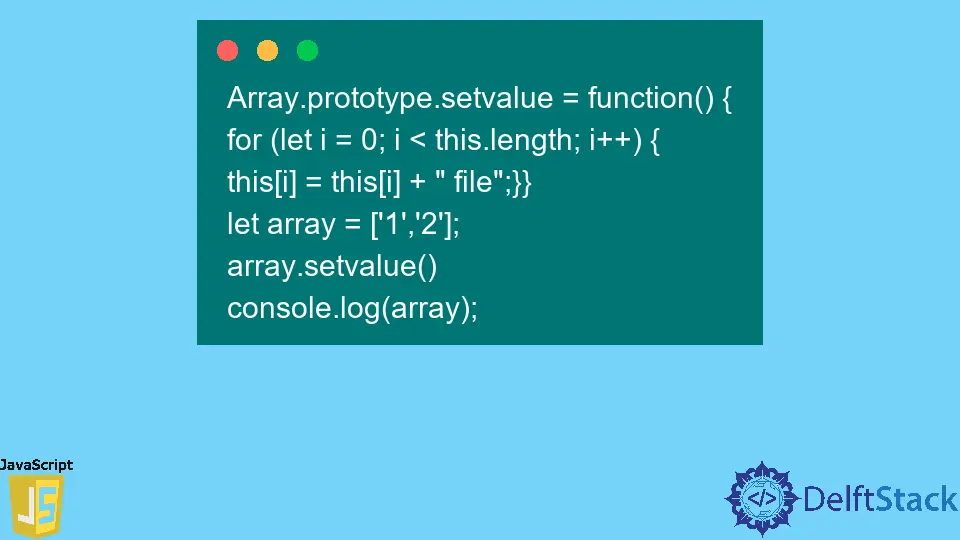
In JavaScript, the array.prototype
property can be used for every array. This method allows us to set functions or words for the elements in an array and change its length.
Syntax of JavaScript array.prototype
Property
Array.prototype.func = function() {
console.log(this[0]);
};
let arr = ["Delft","stack"];
arr.func();
Parameters
arr |
It is a reference array on which we need to call any function defined in array.prototype . |
this[i] |
It represents the value of the i th element of the arr . |
Return
This method changes the actual output of the array and returns the array based on the method used to create the prototype.
Example 1: Use array.prototype
to Get the Custom Word Added in Elements of the Array
The array.prototype
property allows us to add functions to create a prototype for the given array. In this example, we have created an array and added a function that will be used to create a prototype for an array with the array.prototype
property.
const array = ["JavaScript", " C++"];
Array.prototype.val= function () {
for (let i = 0; i < this.length; i++) {
this[i] = this[i].toUpperCase() + " Language";}};
array.val();
console.log(array);
Output:
[ 'JAVASCRIPT Language', ' C++ Language' ]
Example 2: Use array.prototype
With the Function to Create a Prototype for the Array
The array.prototype
property creates the prototype for the given array. In this example, we have created an array.
In the function, we used the increment method so that the prototype could be created for every element in the array. Then, we used the array.prototype
property to access the function and change the actual output of the array.
Array.prototype.setvalue = function() {
for (let i = 0; i < this.length; i++) {
this[i] = this[i] + " file";}}
let array = ['1','2'];
array.setvalue()
console.log(array);
Output:
[ '1 file', '2 file' ]
The array.prototype
method is supported in all browsers of the current version.